在 Java 中模拟 Unix“tail -f”
在 Java 中实现“tail -f”实用程序的功能需要找到一个有效的方法能够监视文件是否有新添加并允许程序连续读取这些新行的技术或库。要实现此目标,请考虑以下方法:
Apache Commons Tailer 类
一个值得注意的选项是 Apache Commons IO 中的 Tailer 类。此类提供了用于监视文件及其修改的简单解决方案。它允许连续读取添加到文件中的新行,类似于“tail -f”的行为。可以使用以下代码实现与 Tailer 类的集成:
Tailer tailer = Tailer.create(new File("application.log"), new TailerListener() { @Override public void handle(String line) { // Perform desired operations on the newly added line } }); tailer.run();
自定义文件监控实现
或者,可以创建一个定期的自定义文件监控实现检查文件大小的变化并相应地更新其内部缓冲区。这种方法需要更深入地了解 Java 中的文件系统行为和事件侦听机制。这是一个简化的示例:
import java.io.File; import java.io.IOException; import java.nio.file.*; import java.nio.file.attribute.BasicFileAttributes; public class TailFileMonitor implements Runnable { private File file; private long lastFileSize; public TailFileMonitor(File file) { this.file = file; this.lastFileSize = file.length(); } @Override public void run() { Path filePath = file.toPath(); try { WatchKey watchKey = filePath.getParent().register(new WatchService(), StandardWatchEventKinds.ENTRY_MODIFY); while (true) { WatchKey key = watchKey.poll(); if (key == watchKey) { for (WatchEvent> event : key.pollEvents()) { WatchEvent.Kind> kind = event.kind(); if (kind == StandardWatchEventKinds.ENTRY_MODIFY && event.context().toString().equals(file.getName())) { tailFile(); } } } key.reset(); } } catch (IOException e) { // Handle IO exceptions } } private void tailFile() { long currentFileSize = file.length(); if (currentFileSize != lastFileSize) { try (BufferedReader br = new BufferedReader(new FileReader(file))) { br.skip(lastFileSize); String line; while ((line = br.readLine()) != null) { // Process newly added lines } lastFileSize = currentFileSize; } catch (IOException e) { // Handle IO exceptions } } } }
要使用自定义监视器,请创建一个实例并将其作为线程执行。它将不断监视文件的更改并相应地更新缓冲区。
以上是如何用Java实现Unix'tail -f”功能?的详细内容。更多信息请关注PHP中文网其他相关文章!
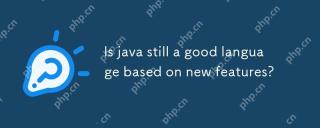
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
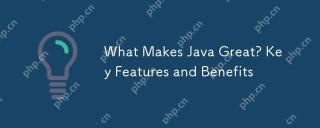
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
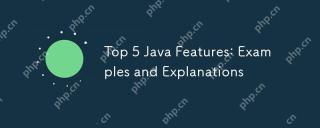
Java的五大特色是多态性、Lambda表达式、StreamsAPI、泛型和异常处理。1.多态性让不同类的对象可以作为共同基类的对象使用。2.Lambda表达式使代码更简洁,特别适合处理集合和流。3.StreamsAPI高效处理大数据集,支持声明式操作。4.泛型提供类型安全和重用性,编译时捕获类型错误。5.异常处理帮助优雅处理错误,编写可靠软件。
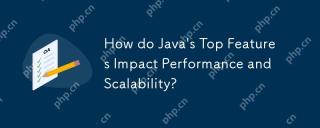
java'stopfeatureSnificallyEnhanceItsperFormanCeanDscalability.1)对象 - 方向 - incipleslike-polymormormormormormormormormormormormormorableablefleandibleandscalablecode.2)garbageCollectionAutoctionAutoctionAutoctionAutoctionAutoctionautomorymanatesmemorymanateMmanateMmanateMmanagementButCancausElatenceiss.3)
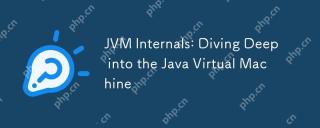
JVM的核心组件包括ClassLoader、RuntimeDataArea和ExecutionEngine。1)ClassLoader负责加载、链接和初始化类和接口。2)RuntimeDataArea包含MethodArea、Heap、Stack、PCRegister和NativeMethodStacks。3)ExecutionEngine由Interpreter、JITCompiler和GarbageCollector组成,负责bytecode的执行和优化。
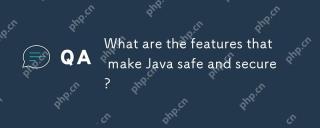
Java'ssafetyandsecurityarebolsteredby:1)strongtyping,whichpreventstype-relatederrors;2)automaticmemorymanagementviagarbagecollection,reducingmemory-relatedvulnerabilities;3)sandboxing,isolatingcodefromthesystem;and4)robustexceptionhandling,ensuringgr
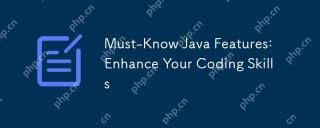
javaoffersseveralkeyfeaturesthatenhancecodingskills:1)对象 - 方向 - 方向上的贝利奥洛夫夫人 - 启动worldentities
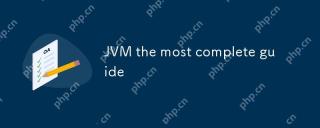
thejvmisacrucialcomponentthatrunsjavacodebytranslatingitolachine特定建筑,影响性能,安全性和便携性。1)theclassloaderloader,links andinitializesClasses.2)executionEccutionEngineExecutionEngineExecutionEngineExecuteByteCuteByteCuteByteCuteBytecuteBytecuteByteCuteByteCuteByteCuteBytecuteByteCodeNinstRonctientions.3)Memo.3)Memo


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
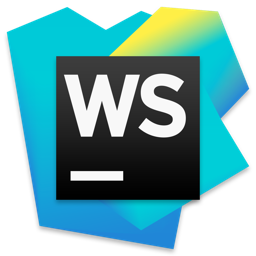
WebStorm Mac版
好用的JavaScript开发工具

SublimeText3汉化版
中文版,非常好用

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),
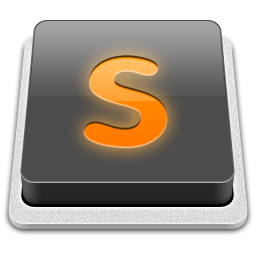
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具