在没有结构体绑定的情况下将 YAML 转换为 JSON
使用 YAML 格式的动态数据时,通常需要将其转换为 JSON 以便进一步处理。然而,直接将 YAML 解组为空的 interface{} 可能会在遇到 map[interface{}]interface{} 类型时导致问题,如给定场景所示。
解决方案:递归类型转换
为了解决这个问题,使用了一个名为convert()的递归函数。该函数迭代interface{}值并执行以下转换:
- 将map[interface{}]interface{}值转换为map[string]interface{}。
- 将 []interface{} 值转换为 []interface{}。
通过这样做,输出的值可以安全地编组为 JSON 字符串。
示例
以下是如何使用 Convert() 函数的示例:
import ( "fmt" "log" "github.com/go-yaml/yaml" "encoding/json" ) func convert(i interface{}) interface{} { switch x := i.(type) { case map[interface{}]interface{}: m2 := map[string]interface{}{} for k, v := range x { m2[k.(string)] = convert(v) } return m2 case []interface{}: for i, v := range x { x[i] = convert(v) } } return i } func main() { // Define the YAML string const s = `Services: - Orders: - ID: $save ID1 SupplierOrderCode: $SupplierOrderCode - ID: $save ID2 SupplierOrderCode: 111111 ` // Unmarshal the YAML string into an empty interface var body interface{} if err := yaml.Unmarshal([]byte(s), &body); err != nil { log.Fatal(err) } // Recursively convert the interface to a map[string]interface{} body = convert(body) // Marshal the converted interface into a JSON string if b, err := json.Marshal(body); err != nil { log.Fatal(err) } else { fmt.Println("Converted JSON:", string(b)) } }
输出
程序的输出是转换后的JSON:
Converted JSON: {"Services":[{"Orders":[ {"ID":"$save ID1","SupplierOrderCode":"$SupplierOrderCode"}, {"ID":"$save ID2","SupplierOrderCode":111111}]}]}
注释
- 通过转换为Go映射,元素的顺序会丢失,因此可能不适合顺序很重要的场景。
- convert() 函数的优化和改进版本已作为库发布在 github.com/icza/dyno 上。它为处理动态 YAML 数据提供了便利和灵活性。
以上是如何在 Go 中安全地将 YAML 转换为 JSON,而不丢失数据完整性?的详细内容。更多信息请关注PHP中文网其他相关文章!
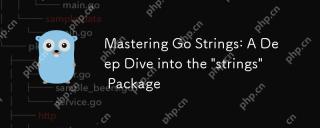
你应该关心Go语言中的"strings"包,因为它提供了处理文本数据的工具,从基本的字符串拼接到高级的正则表达式匹配。1)"strings"包提供了高效的字符串操作,如Join函数用于拼接字符串,避免性能问题。2)它包含高级功能,如ContainsAny函数,用于检查字符串是否包含特定字符集。3)Replace函数用于替换字符串中的子串,需注意替换顺序和大小写敏感性。4)Split函数可以根据分隔符拆分字符串,常用于正则表达式处理。5)使用时需考虑性能,如
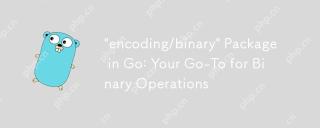
“编码/二进制”软件包interingoisentialForHandlingBinaryData,oferingToolSforreDingingAndWritingBinaryDataEfficely.1)Itsupportsbothlittle-endianandBig-endianBig-endianbyteorders,CompialforOss-System-System-System-compatibility.2)
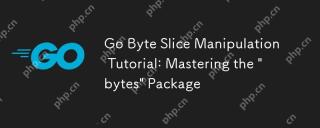
掌握Go语言中的bytes包有助于提高代码的效率和优雅性。1)bytes包对于解析二进制数据、处理网络协议和内存管理至关重要。2)使用bytes.Buffer可以逐步构建字节切片。3)bytes包提供了搜索、替换和分割字节切片的功能。4)bytes.Reader类型适用于从字节切片读取数据,特别是在I/O操作中。5)bytes包与Go的垃圾回收器协同工作,提高了大数据处理的效率。
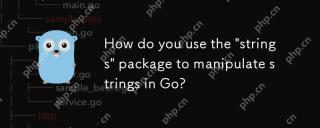
你可以使用Go语言中的"strings"包来操纵字符串。1)使用strings.TrimSpace去除字符串两端的空白字符。2)用strings.Split将字符串按指定分隔符拆分成切片。3)通过strings.Join将字符串切片合并成一个字符串。4)用strings.Contains检查字符串是否包含特定子串。5)利用strings.ReplaceAll进行全局替换。注意使用时要考虑性能和潜在的陷阱。
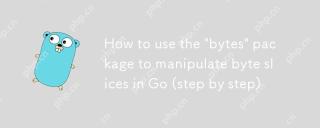
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
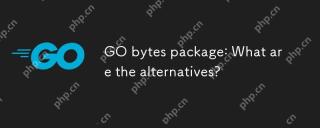
thealternativestogo'sbytespackageincageincludethestringspackage,bufiopackage和customstructs.1)thestringspackagecanbeusedforbytemanipulationforbytemanipulationbybyconvertingbytestostostostostostrings.2))
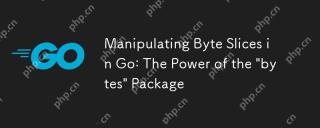
“字节”包装封装forefforeflyManipulatingByteslices,CocialforbinaryData,网络交易和andfilei/o.itoffersfunctionslikeIndexForsearching,BufferForhandLinglaRgedLargedLargedAtaTasets,ReaderForsimulatingStreamReadReadImreAmreadReamReadinging,以及Joineffiter和Joineffiter和Joineffore
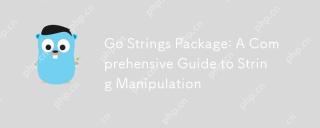
go'sstringspackageIscialforficientficientsTringManipulation,uperingToolSlikestrings.split(),strings.join(),strings.replaceall(),andStrings.contains.contains.contains.contains.contains.contains.split.split(split()strings.split()dividesStringoSubSubStrings; 2)strings.joins.joins.joinsillise.joinsinelline joinsiline joinsinelline; 3);


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。

SublimeText3 英文版
推荐:为Win版本,支持代码提示!

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中
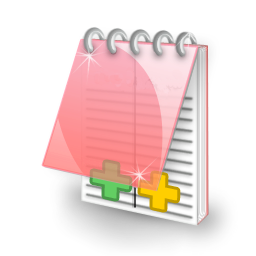
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能