在 C 中不使用 if/switch 将枚举值打印为文本
在 C 中,枚举提供了一种便捷的方式来表示一组命名常量。但是,当打印枚举值时,默认行为是显示其数字表示形式。
假设我们有一个像这样的枚举:
enum Errors { ErrorA = 0, ErrorB, ErrorC, };
如果我们尝试使用打印枚举值std::cout:
Errors anError = ErrorA; std::cout <p>我们将得到数值 0 而不是文本表示“ErrorA”。出现此问题的原因是 std::cout 缺乏将枚举转换为字符串的内置支持。</p><h3 id="解决方案">解决方案</h3><p><strong>1.使用映射</strong></p><p>一种方法是创建一个将每个枚举值与其文本表示关联的映射:</p><pre class="brush:php;toolbar:false">#include <map> #include <string_view> std::ostream& operator result; #define INSERT_ELEMENT(p) result.emplace(p, #p); INSERT_ELEMENT(ErrorA); INSERT_ELEMENT(ErrorB); INSERT_ELEMENT(ErrorC); #undef INSERT_ELEMENT return result; }; return out <p><strong>2。使用结构数组进行线性搜索</strong></p> <p>另一种选择是使用结构数组,每个结构数组包含一个枚举值及其文本表示形式,然后执行线性搜索:</p> <pre class="brush:php;toolbar:false">#include <string_view> std::ostream& operatorstr; i++) { if (i->value == value) { s = i->str; break; } } return out <p><strong>3。使用 switch/case</strong></p> <p>最后,我们还可以使用 switch/case 语句:</p> <pre class="brush:php;toolbar:false">#include <string> std::ostream& operator<p><strong>测试解决方案:</strong></p> <p>为了测试这些解决方案,我们可以使用以下代码创建一个可执行文件:</p> <pre class="brush:php;toolbar:false">#include <iostream> int main(int argc, char** argv) { std::cout <p>运行此可执行文件应输出枚举值的文本表示形式:</p> <pre class="brush:php;toolbar:false">ErrorA ErrorB ErrorC
以上是如何在不使用 if/switch 语句的情况下将 C 枚举值打印为文本?的详细内容。更多信息请关注PHP中文网其他相关文章!

Gulc是一个高性能的C库,优先考虑最小开销,积极的内衬和编译器优化。 其设计非常适合高频交易和嵌入式系统等关键应用程序,其设计强调简单性,模型
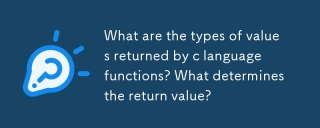
本文详细介绍了C函数返回类型,包括基本(int,float,char等),派生(数组,指针,结构)和void类型。 编译器通过函数声明和返回语句确定返回类型,执行
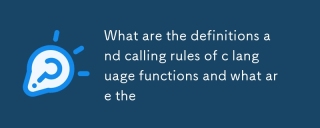
本文解释了C函数声明与定义,参数传递(按值和指针),返回值以及常见的陷阱,例如内存泄漏和类型不匹配。 它强调了声明对模块化和省份的重要性

本文详细介绍了字符串案例转换的C功能。 它可以通过ctype.h的toupper()和tolower()解释,并通过字符串迭代并处理零终端。 常见的陷阱,例如忘记ctype.h和修改字符串文字是
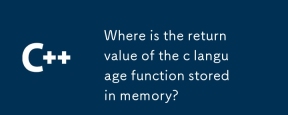
本文研究C函数返回值存储。 较小的返回值通常存储在寄存器中以备速度;较大的值可能会使用指针来记忆(堆栈或堆),影响寿命并需要手动内存管理。直接ACC
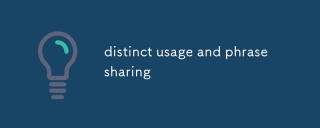
本文分析了形容词“独特”的多方面用途,探索其语法功能,常见的短语(例如,“不同于”,“完全不同”),以及在正式与非正式中的细微应用
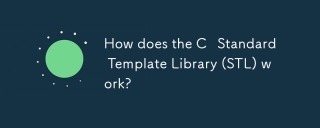
本文解释了C标准模板库(STL),重点关注其核心组件:容器,迭代器,算法和函子。 它详细介绍了这些如何交互以启用通用编程,提高代码效率和可读性t
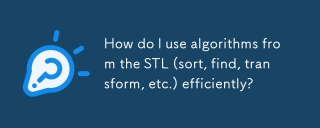
本文详细介绍了c中有效的STL算法用法。 它强调了数据结构选择(向量与列表),算法复杂性分析(例如,std :: sort vs. std vs. std :: partial_sort),迭代器用法和并行执行。 常见的陷阱


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具
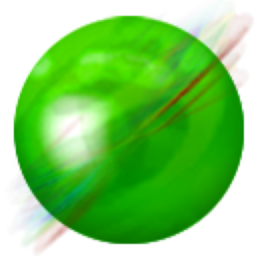
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

SublimeText3 Linux新版
SublimeText3 Linux最新版

记事本++7.3.1
好用且免费的代码编辑器