元组是 Python 中重要的数据结构,提供了一种存储有序且不可变数据集合的便捷方法。
在本博客中,您将了解有关 Python 中元组的所有内容,包括创建、切片、方法等等。
让我们直接开始吧!?
Python 中的元组
元组是数据项的有序集合。在元组中,您可以将多个项目存储在单个变量中。
元组是不可变的,即创建后您无法更改它们。
创建元组
元组使用圆括号 () 定义,项目之间用逗号分隔。
元组可以包含不同数据类型的项。
例如:
tuple1 = (1,2,36,3,15) tuple2 = ("Red", "Yellow", "Blue") tuple3 = (1, "John",12, 5.3) print(tuple1) # (1, 2, 36, 3, 15) print(tuple2) # ('Red', 'Yellow', 'Blue') print(tuple3) # (1, 'John', 12, 5.3)
单项元组
要创建包含一项的元组,请在该项后面添加一个逗号。如果没有逗号,Python 会将其视为整数类型。
例如:
tuple1 = (1) # This is an integer. print(type(tuple1)) # <class> tuple2 = (1,) # This is a tuple. print(type(tuple2)) # <class> </class></class>
元组长度
您可以使用 len() 函数查找元组的长度(元组中的项目数)。
例如:
tuple1 = (1,2,36,3,15) lengthOfTuple = len(tuple1) print(lengthOfTuple) # 5
访问元组项
您可以使用索引访问元组项/元素。每个元素都有其唯一的索引。
第一个元素的索引从 0 开始,第二个元素的索引从 1 开始,依此类推。
例如:
fruits = ("Orange", "Apple", "Banana") print(fruits[0]) # Orange print(fruits[1]) # Apple print(fruits[2]) # Banana
您还可以从元组末尾访问元素(-1 表示最后一个元素,-2 表示倒数第二个元素,依此类推),这称为负索引。
例如:
fruits = ("Orange", "Apple", "Banana") print(fruits[-1]) # Banana print(fruits[-2]) # Apple print(fruits[-3]) # Orange # for understanding, you can consider this as fruits[len(fruits)-3]
检查元组中是否存在某个项目
您可以使用 in 关键字检查元组中是否存在某个元素。
示例1:
fruits = ("Orange", "Apple", "Banana") if "Orange" in fruits: print("Orange is in the tuple.") else: print("Orange is not in the tuple.") #Output: Orange is in the tuple.
示例2:
numbers = (1, 57, 13) if 7 in numbers: print("7 is in the tuple.") else: print("7 is not in the tuple.") # Output: 7 is not in the tuple.
元组切片
您可以通过给出开始、结束和跳转(跳过)参数来获取一系列元组项目。
语法:
tupleName[start : end : jumpIndex]
注意:跳转索引是可选的。
示例1:
# Printing elements within a particular range numbers = (1, 57, 13, 6, 18, 54) # using positive indexes(this will print the items starting from index 2 and ending at index 4 i.e. (5-1)) print(numbers[2:5]) # using negative indexes(this will print the items starting from index -5 and ending at index -3 i.e. (-2-1)) print(numbers[-5:-2])
输出:
(13, 6, 18) (57, 13, 6)
示例2:
当没有提供结束索引时,解释器将打印直到末尾的所有值。
# Printing all elements from a given index to till the end numbers = (1, 57, 13, 6, 18, 54) # using positive indexes print(numbers[2:]) # using negative indexes print(numbers[-5:])
输出:
(13, 6, 18, 54) (57, 13, 6, 18, 54)
示例3:
当没有提供开始索引时,解释器会打印从开始到提供的结束索引的所有值。
# Printing all elements from start to a given index numbers = (1, 57, 13, 6, 18, 54) #using positive indexes print(numbers[:4]) #using negative indexes print(numbers[:-2])
输出:
(1, 57, 13, 6) (1, 57, 13, 6)
示例 4:
您可以通过给出跳转索引来打印替代值。
# Printing alternate values numbers = (1, 57, 13, 6, 18, 54) # using positive indexes(here start and end indexes are not given and 2 is jump index.) print(numbers[::2]) # using negative indexes(here start index is -2, end index is not given and 2 is jump index.) print(numbers[-2::2])
输出:
(1, 13, 18) (18)
操作元组
元组不可变,因此无法添加、删除或更改项目。但是,您可以将元组转换为列表,修改列表,然后将其转换回元组。
例如:
tuple1 = (1,2,36,3,15) tuple2 = ("Red", "Yellow", "Blue") tuple3 = (1, "John",12, 5.3) print(tuple1) # (1, 2, 36, 3, 15) print(tuple2) # ('Red', 'Yellow', 'Blue') print(tuple3) # (1, 'John', 12, 5.3)
连接元组
您可以使用运算符连接两个元组。
例如:
tuple1 = (1) # This is an integer. print(type(tuple1)) # <class> tuple2 = (1,) # This is a tuple. print(type(tuple2)) # <class> </class></class>
输出:
tuple1 = (1,2,36,3,15) lengthOfTuple = len(tuple1) print(lengthOfTuple) # 5
元组方法
Tuple 有以下内置方法:
数数()
此方法返回元素在元组中出现的次数。
语法:
fruits = ("Orange", "Apple", "Banana") print(fruits[0]) # Orange print(fruits[1]) # Apple print(fruits[2]) # Banana
例如:
fruits = ("Orange", "Apple", "Banana") print(fruits[-1]) # Banana print(fruits[-2]) # Apple print(fruits[-3]) # Orange # for understanding, you can consider this as fruits[len(fruits)-3]
指数()
此方法返回元组中给定元素的第一次出现。
注意:如果在元组中找不到该元素,此方法会引发 ValueError。
例如:
fruits = ("Orange", "Apple", "Banana") if "Orange" in fruits: print("Orange is in the tuple.") else: print("Orange is not in the tuple.") #Output: Orange is in the tuple.
您可以为搜索指定起始索引。例如:
numbers = (1, 57, 13) if 7 in numbers: print("7 is in the tuple.") else: print("7 is not in the tuple.") # Output: 7 is not in the tuple.
今天就这些。
希望对您有帮助。
感谢您的阅读。
我在学习 Python 语言时创建了详细的 Python 笔记,而且仅需 1 美元!在这里获取它们:立即下载
有关更多此类内容,请点击此处。
在 X(Twitter) 上关注我,获取日常 Web 开发技巧。
继续编码!!
以上是掌握 Python 中的元组:综合指南的详细内容。更多信息请关注PHP中文网其他相关文章!
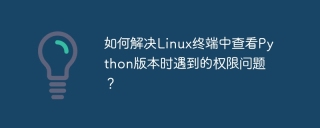
Linux终端中查看Python版本时遇到权限问题的解决方法当你在Linux终端中尝试查看Python的版本时,输入python...
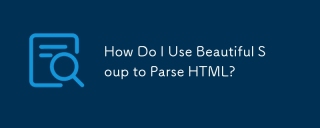
本文解释了如何使用美丽的汤库来解析html。 它详细介绍了常见方法,例如find(),find_all(),select()和get_text(),以用于数据提取,处理不同的HTML结构和错误以及替代方案(SEL)
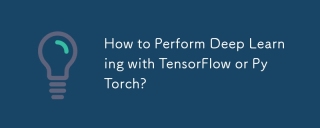
本文比较了Tensorflow和Pytorch的深度学习。 它详细介绍了所涉及的步骤:数据准备,模型构建,培训,评估和部署。 框架之间的关键差异,特别是关于计算刻度的
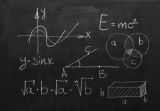
Python的statistics模块提供强大的数据统计分析功能,帮助我们快速理解数据整体特征,例如生物统计学和商业分析等领域。无需逐个查看数据点,只需查看均值或方差等统计量,即可发现原始数据中可能被忽略的趋势和特征,并更轻松、有效地比较大型数据集。 本教程将介绍如何计算平均值和衡量数据集的离散程度。除非另有说明,本模块中的所有函数都支持使用mean()函数计算平均值,而非简单的求和平均。 也可使用浮点数。 import random import statistics from fracti
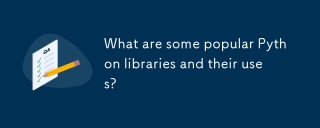
本文讨论了诸如Numpy,Pandas,Matplotlib,Scikit-Learn,Tensorflow,Tensorflow,Django,Blask和请求等流行的Python库,并详细介绍了它们在科学计算,数据分析,可视化,机器学习,网络开发和H中的用途
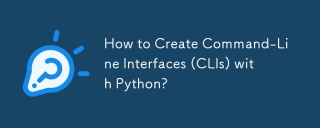
本文指导Python开发人员构建命令行界面(CLIS)。 它使用Typer,Click和ArgParse等库详细介绍,强调输入/输出处理,并促进用户友好的设计模式,以提高CLI可用性。

在使用Python的pandas库时,如何在两个结构不同的DataFrame之间进行整列复制是一个常见的问题。假设我们有两个Dat...
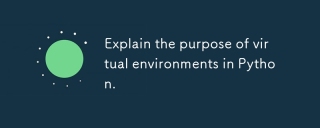
文章讨论了虚拟环境在Python中的作用,重点是管理项目依赖性并避免冲突。它详细介绍了他们在改善项目管理和减少依赖问题方面的创建,激活和利益。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),

SublimeText3汉化版
中文版,非常好用
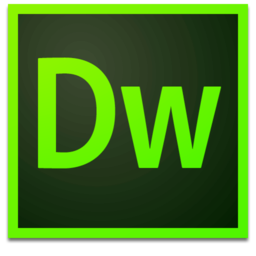
Dreamweaver Mac版
视觉化网页开发工具
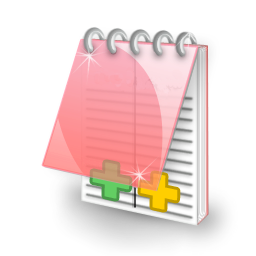
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。