如何有效地“最大化”并发 HTTP 请求?
在尝试 Go 的性能时,您可能会在尝试并发执行大量 HTTP 请求时遇到限制。本文探讨了所面临的挑战,并提供了实现最大并发性的解决方案。
问题
您最初的方法涉及启动大量 goroutine 并行发送 HTTP 请求,期望它们利用所有可用的 CPU。但是,由于文件描述符限制,您会遇到错误。
解决方案
要克服这些限制,请考虑以下方法:
- 使用有界信号量通道来控制并发: 实现一个缓冲通道作为信号量,限制并发请求的数量。通过调整通道缓冲区大小和调整 GOMAXPROCS,您可以优化系统的并发性。
- 通过调度程序利用工作池: 采用工作池模式,将请求排队到通道中。调度程序填充通道,而一组工作人员一次处理一个请求。这种方法可确保维持最大并发请求数。
- 利用专用的消费者 Goroutine 来处理响应:为了避免阻塞请求处理,请使用专用的 Goroutine 来消费来自 Worker 的响应水池。这可确保连续执行请求,使您的系统能够处理更多并发请求。
优化代码
以下是包含这些优化的代码的修改版本:
package main import ( "fmt" "net/http" "runtime" "sync" "time" ) var ( reqs int concurrent int work chan *http.Request results chan *http.Response ) func init() { reqs = 1000000 concurrent = 200 } func main() { runtime.GOMAXPROCS(runtime.NumCPU()) work = make(chan *http.Request, concurrent) results = make(chan *http.Response) start := time.Now() // Create a semaphore channel to limit concurrency sem := make(chan struct{}, concurrent) // Create a dispatcher to populate the work channel go func() { for i := 0; i <p>通过调整并发变量并观察结果,您可以确定系统的最佳并发级别,“最大化”其并发 HTTP 的能力请求。</p>
以上是如何在 Go 中最大化并发 HTTP 请求?的详细内容。更多信息请关注PHP中文网其他相关文章!
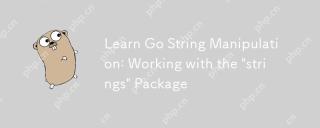
Go的"strings"包提供了丰富的功能,使字符串操作高效且简单。1)使用strings.Contains()检查子串。2)strings.Split()可用于解析数据,但需谨慎使用以避免性能问题。3)strings.Join()适用于格式化字符串,但对小数据集,循环使用 =更有效。4)对于大字符串,使用strings.Builder构建字符串更高效。
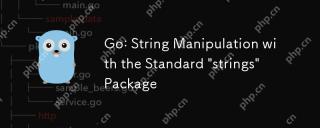
Go语言使用"strings"包进行字符串操作。1)拼接字符串使用strings.Join函数。2)查找子串使用strings.Contains函数。3)替换字符串使用strings.Replace函数,这些函数高效且易用,适用于各种字符串处理任务。
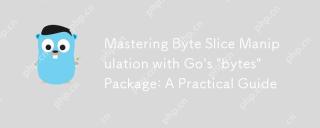
资助bytespackageingoisesential foreffited byteSemanipulation,uperingFunctionsLikeContains,index,andReplaceForsearchingangingAndModifyingBinaryData.itenHancesperformanceNandCoderAceAnibility,MakeitiTavitalToolToolToolToolToolToolToolToolToolForhandLingBinaryData,networkProtocols,networkProtocoLss,networkProtocols,andetFilei
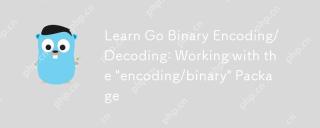
Go语言使用"encoding/binary"包进行二进制编码与解码。1)该包提供binary.Write和binary.Read函数,用于数据的写入和读取。2)需要注意选择正确的字节序(如BigEndian或LittleEndian)。3)数据对齐和错误处理也是关键,确保数据的正确性和性能。
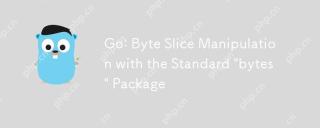
1)usebybytes.joinforconcatenatinges,2)bytes.bufferforincrementalWriter,3)bytes.indexorbytes.indexorbytes.indexbyteforsearching bytes.bytes.readereforrednerncretinging.isnchunk.ss.ind.inc.softes.4)
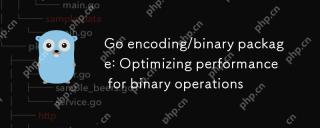
theencoding/binarypackageingoiseforporptimizingBinaryBinaryOperationsDuetoitssupportforendiannessessandefficityDatahandling.toenhancePerformance:1)usebinary.nativeendiandiandiandiandiandiandiandian nessideendian toavoid avoidByteByteswapping.2)
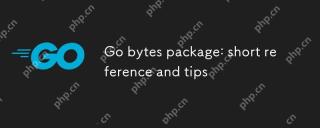
Go的bytes包主要用于高效处理字节切片。1)使用bytes.Buffer可以高效进行字符串拼接,避免不必要的内存分配。2)bytes.Equal函数用于快速比较字节切片。3)bytes.Index、bytes.Split和bytes.ReplaceAll函数可用于搜索和操作字节切片,但需注意性能问题。
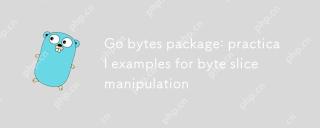
字节包提供了多种功能来高效处理字节切片。1)使用bytes.Contains检查字节序列。2)用bytes.Split分割字节切片。3)通过bytes.Replace替换字节序列。4)用bytes.Join连接多个字节切片。5)利用bytes.Buffer构建数据。6)结合bytes.Map进行错误处理和数据验证。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。
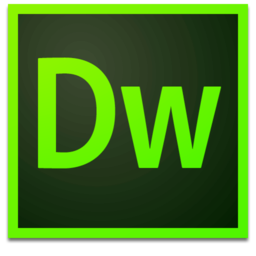
Dreamweaver Mac版
视觉化网页开发工具

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。
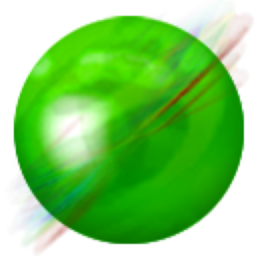
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境
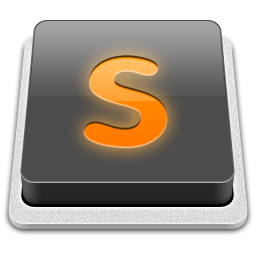
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)