Python 语法和基本结构入门
现在您已经安装了 Python 并运行了您的第一个程序,让我们深入研究一些构成每个 Python 程序支柱的基本概念。在这篇文章中,我们将介绍 Python 的语法、运算符和输入/输出操作,为编写函数式代码奠定基础。
1. 基本语法和结构
Python 的语法设计得简洁且易于阅读,但在深入进行更复杂的编码之前,您需要了解一些基本规则。
缩进
与许多其他编程语言不同,Python 使用缩进来定义代码块,而不是大括号 ({}) 或关键字。这使您的代码在视觉上更干净,但也意味着您必须与间距保持一致。
代码示例:使用缩进
# Correct indentation if True: print("This is properly indented!") # Incorrect indentation (will cause an error) if True: print("This is not properly indented!")
评论
注释用于使代码更具可读性并记录其功能。 Python 使用 # 符号进行单行注释。
# This is a single-line comment print("Python ignores comments when running the code.")
对于多行注释,请使用三引号:
""" This is a multi-line comment. """
关键字和标识符
关键字:Python 中的保留字,如 if、else、for 和 def。您不能将它们用作变量名称。
标识符:用于变量、函数或类的名称。它们必须以字母或下划线 (_) 开头,并且不能包含特殊字符。
2.Python中的运算符
运算符是用于对变量和值执行运算的符号。 Python 提供了多种运算符。
算术运算符
用于基本数学运算:
a = 10 b = 3 print(a + b) # Addition print(a - b) # Subtraction print(a * b) # Multiplication print(a / b) # Division print(a % b) # Modulus print(a ** b) # Exponentiation print(a // b) # Floor division
比较运算符
比较两个值并返回布尔值(True 或 False):
print(a > b) # Greater than print(a = b) # Greater than or equal to print(a <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/173253464048351.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="Basic Concepts – Operators and More"></p> <p><strong>逻辑运算符</strong></p> <p>组合条件语句:<br> </p> <pre class="brush:php;toolbar:false">x = True y = False print(x and y) # Logical AND print(x or y) # Logical OR print(not x) # Logical NOT
3. 输入输出
接受输入
Python 的 input() 函数允许您通过在程序执行期间接收输入来与用户交互。
代码示例:简单输入
name = input("What is your name? ") print(f"Hello, {name}!")
显示输出
print()函数用于显示信息。您还可以使用 f 字符串进行格式化输出:
# Correct indentation if True: print("This is properly indented!") # Incorrect indentation (will cause an error) if True: print("This is not properly indented!")
4. 迷你项目:基本计算器
让我们将所有内容放在一起创建一个简单的计算器程序,该程序接受用户输入,执行基本操作并显示结果。
代码示例:基本计算器
# This is a single-line comment print("Python ignores comments when running the code.")
练习练习
为了巩固您所学的知识,请尝试以下额外练习:
- 偶数或奇数检查器: 创建一个程序,将数字作为输入并打印它是偶数还是奇数。
""" This is a multi-line comment. """
- 扩展计算器: 通过添加对模数和指数的支持来增强基本计算器。例如:
a = 10 b = 3 print(a + b) # Addition print(a - b) # Subtraction print(a * b) # Multiplication print(a / b) # Division print(a % b) # Modulus print(a ** b) # Exponentiation print(a // b) # Floor division
结论
了解Python的语法、运算符和输入/输出操作是成为自信程序员的第一步。有了这些基础,您就可以处理更高级的主题和项目了。
尝试一下练习,并在下面的评论中告诉我们您的表现!我们很乐意看到您的结果,并在您遇到困难时为您提供帮助。
以上是基本概念 – 运算符等的详细内容。更多信息请关注PHP中文网其他相关文章!
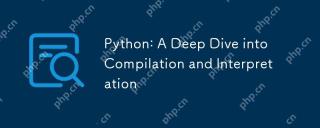
pythonisehybridmodelofcompilationand interpretation:1)thepythoninterspretercompilesourcececodeintoplatform- interpententbybytecode.2)thepytythonvirtualmachine(pvm)thenexecuteCutestestestesteSteSteSteSteSteSthisByTecode,BelancingEaseofuseWithPerformance。
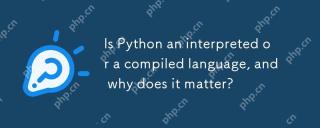
pythonisbothinterpretedAndCompiled.1)它的compiledTobyTecodeForportabilityAcrosplatforms.2)bytecodeisthenInterpreted,允许fordingfordforderynamictynamictymictymictymictyandrapiddefupment,尽管Ititmaybeslowerthananeflowerthanancompiledcompiledlanguages。
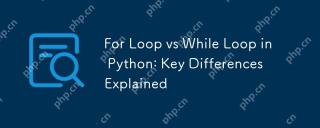
在您的知识之际,而foroopsareideal insinAdvance中,而WhileLoopSareBetterForsituations则youneedtoloopuntilaconditionismet
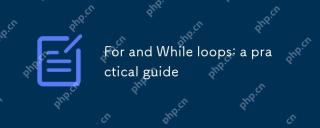
ForboopSareSusedwhenthentheneMberofiterationsiskNownInAdvance,而WhileLoopSareSareDestrationsDepportonAcondition.1)ForloopSareIdealForiteratingOverSequencesLikelistSorarrays.2)whileLeleLooleSuitableApeableableableableableableforscenarioscenarioswhereTheLeTheLeTheLeTeLoopContinusunuesuntilaspecificiccificcificCondond
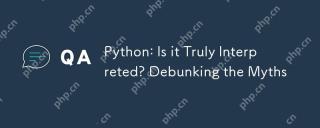
pythonisnotpuroly interpred; itosisehybridablectofbytecodecompilationandruntimeinterpretation.1)PythonCompiLessourceceCeceDintobyTecode,whitsthenexecececected bytybytybythepythepythepythonvirtirtualmachine(pvm).2)
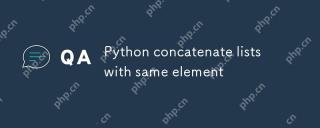
concateNateListsinpythonwithTheSamelements,使用:1)operatototakeepduplicates,2)asettoremavelemavphicates,or3)listCompreanspearensionforcontroloverduplicates,每个methodhasdhasdifferentperferentperferentperforentperforentperforentperfortenceandordormplications。
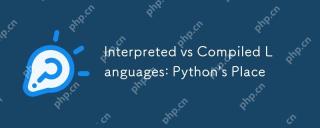
pythonisanterpretedlanguage,offeringosofuseandflexibilitybutfacingperformancelanceLimitationsInCricapplications.1)drightingedlanguageslikeLikeLikeLikeLikeLikeLikeLikeThonexecuteline-by-line,允许ImmediaMediaMediaMediaMediaMediateFeedBackAndBackAndRapidPrototypiD.2)compiledLanguagesLanguagesLagagesLikagesLikec/c thresst
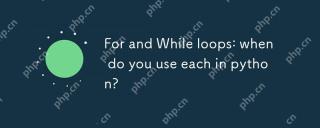
Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
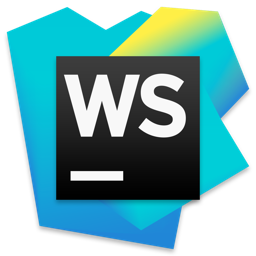
WebStorm Mac版
好用的JavaScript开发工具

SublimeText3汉化版
中文版,非常好用

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),
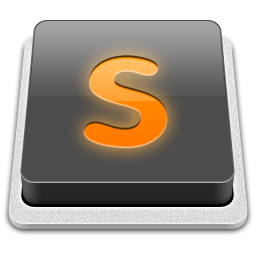
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具