在 C 语言中使用 Boost 创建线程池
在 C 语言中,使用 Boost 库创建线程池需要一个简单的过程。
首先,实例化一个 asio::io_service 和一个 thread_group。随后,使用连接到 io_service 的线程填充 thread_group。然后可以利用 boost::bind 函数将任务分配给线程。
要停止线程,只需停止 io_service 并将所有线程组合起来即可。
必要的头文件是:
#include <boost> #include <boost> #include <boost></boost></boost></boost>
下面提供了一个示例实现:
// Establish an io_service and a thread_group (essentially a pool) boost::asio::io_service ioService; boost::thread_group threadpool; // Commence ioService processing loop boost::asio::io_service::work work(ioService); // Add threads to pool (e.g., two in this case) threadpool.create_thread( boost::bind(&boost::asio::io_service::run, &ioService) ); threadpool.create_thread( boost::bind(&boost::asio::io_service::run, &ioService) ); // Assign tasks to thread pool via ioService.post() // Refer to "http://www.boost.org/doc/libs/1_54_0/libs/bind/bind.html#with_functions" for details on boost::bind ioService.post(boost::bind(myTask, "Hello World!")); ioService.post(boost::bind(clearCache, "./cache")); ioService.post(boost::bind(getSocialUpdates, "twitter,gmail,facebook,tumblr,reddit")); // Halt ioService processing loop (no new tasks will execute after this point) ioService.stop(); // Wait and combine threads in thread pool threadpool.join_all();
(来源:Recipes
)阿西奥)以上是如何在 C 中使用 Boost 创建线程池?的详细内容。更多信息请关注PHP中文网其他相关文章!
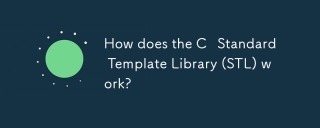
本文解释了C标准模板库(STL),重点关注其核心组件:容器,迭代器,算法和函子。 它详细介绍了这些如何交互以启用通用编程,提高代码效率和可读性t
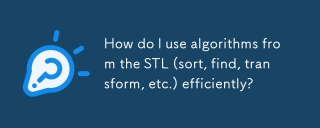
本文详细介绍了c中有效的STL算法用法。 它强调了数据结构选择(向量与列表),算法复杂性分析(例如,std :: sort vs. std vs. std :: partial_sort),迭代器用法和并行执行。 常见的陷阱
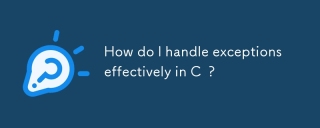
本文详细介绍了C中的有效异常处理,涵盖了尝试,捕捉和投掷机制。 它强调了诸如RAII之类的最佳实践,避免了不必要的捕获块,并为强大的代码登录例外。 该文章还解决了Perf
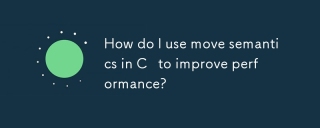
本文讨论了使用C中的移动语义来通过避免不必要的复制来提高性能。它涵盖了使用std :: Move的实施移动构造函数和任务运算符,并确定了关键方案和陷阱以有效
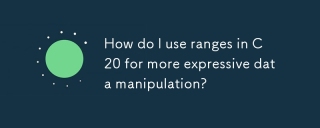
C 20范围通过表现力,合成性和效率增强数据操作。它们简化了复杂的转换并集成到现有代码库中,以提高性能和可维护性。
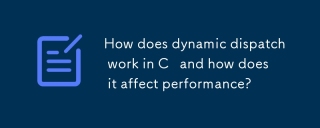
本文讨论了C中的动态调度,其性能成本和优化策略。它突出了动态调度会影响性能并将其与静态调度进行比较的场景,强调性能和之间的权衡
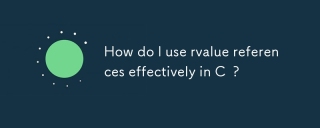
文章讨论了在C中有效使用RVALUE参考,以进行移动语义,完美的转发和资源管理,重点介绍最佳实践和性能改进。(159个字符)


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

Atom编辑器mac版下载
最流行的的开源编辑器
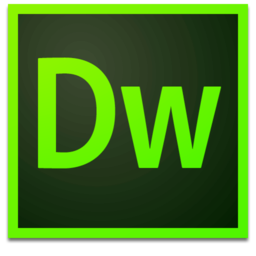
Dreamweaver Mac版
视觉化网页开发工具
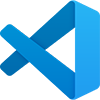
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。
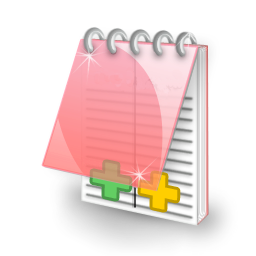
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能