最新的 ECMAScript 版本 ES15 引入了一些新功能,为 Javascript 开发人员提供出色的开发体验。这些增强功能跨越不同领域,从更新的 Javascript 语法和数据处理到安全性、性能和提高开发人员生产力的工具方面的进步。
1. 数组分组
ES15 中最令人兴奋(也是我个人最喜欢的功能之一)的功能是 Object.groupBy() 方法。
这种方式简化了根据特定标准对数组中的元素进行分组的方式。这使得数据操作更加高效并且不易出错。
示例:
const cities = [ { name: 'Melbourne', country: 'Australia' }, { name: 'Auckland', country: 'New Zealand' }, { name: 'Sydney', country: 'Australia' }, { name: 'Brisbane', country: 'Australia' }, { name: 'Wellington', country: 'New Zealand' } ]; const groupedByCountry = Object.groupBy(cities, fruit => fruit.country); console.log(groupedByCountry); // Output: // { // "Australia": [ // { "name": "Melbourne", "country": "Australia" }, // { "name": "Sydney", "country": "Australia" }, // { "name": "Brisbane", "country": "Australia" } // ], // "New Zealand": [ // { "name": "Auckland", "country": "New Zealand" }, // { "name": "Wellington", "country": "New Zealand" } // ] // }
通过使用此功能,我们可以减少对传统上用于数组分组的自定义函数或第三方库的需求。
此外,通过此功能,我们可以通过直接表达我们的意图来使我们的代码更易于理解和维护
2. 管道运算符(|>)
有时我们需要使用多个函数作为链接过程。在这种情况下,我们可以使用管道运算符 (|>) 来简化链接过程。
示例:
const double = (x) => x * 2; const increment = (x) => x + 1; const square = (x) => x * x; const result = 5 |> double |> increment |> square; // Output: 121
上面的传统做法是这样的
const double = (x) => x * 2; const increment = (x) => x + 1; const square = (x) => x * x; const result = square(increment(double(5))); console.log(result); // Output: 121
通过使用管道运算符,我们可以使用更函数式的编程风格。由此,我们可以通过消除深度嵌套函数调用的复杂性来使代码更具可读性。
3. 方法链接运算符 (?.())
ES15 通过引入新的方法链运算符扩展了可选链。此方法链接运算符增加了深度嵌套对象中方法调用的安全性。
示例:
const data = { user: { getName: () => 'Tim' } }; console.log(data.user?.getName?.()); // Output: 'Alice' console.log(data.user?.getAge?.()); // Output: undefined
方法链接运算符 (?.()) 允许您安全地调用可能为 null 或未定义的对象上的方法。这降低了调用方法引起的运行时错误的风险。
4. 设置方法增强
ES15 引入了对 Set 对象的多项增强功能,包括 union、intersection、difference 和 symmetryDifference 等新方法。这些方法简化了常见的集合操作。
const setA = new Set([1, 2, 3]); const setB = new Set([3, 4, 5]); const unionSet = setA.union(setB); const differenceSet = setA.difference(setB); const intersectionSet = setA.intersection(setB); const symmetricDifferenceSet = setA.symmetricDifference(setB); console.log(unionSet); // Output: {1, 2, 3, 4, 5} console.log(differenceSet); // Output: {1, 2} console.log(intersectionSet); // Output: {3} console.log(symmetricDifferenceSet); // Output: {1, 2, 4, 5}
联盟
Set 实例的 union() 方法接受一个集合并返回一个新集合,其中包含该集合和给定集合中的一个或两个中的元素。差异
Set 实例的 Difference() 方法接受一个集合并返回一个新集合,其中包含该集合中但不包含给定集合中的元素。交叉路口
Set 实例的 intersection() 方法接受一个集合并返回一个新集合,其中包含该集合和给定集合中的元素。对称差异
Set 实例的 symmetryDifference() 方法接受一个集合并返回一个新集合,其中包含位于该集合或给定集合中的元素,但不同时位于两者中。
5. 增强的 JSON 模块
在之前的 ECMAScript 版本中,开发人员依赖捆绑器或加载器来导入 JSON 文件。 ES15 现在支持动态导入和模式验证,可以更轻松地处理结构化数据并确保导入的数据符合预期格式。
您现在可以直接导入 JSON 数据,就像导入 JavaScript 模块一样。
示例:
const cities = [ { name: 'Melbourne', country: 'Australia' }, { name: 'Auckland', country: 'New Zealand' }, { name: 'Sydney', country: 'Australia' }, { name: 'Brisbane', country: 'Australia' }, { name: 'Wellington', country: 'New Zealand' } ]; const groupedByCountry = Object.groupBy(cities, fruit => fruit.country); console.log(groupedByCountry); // Output: // { // "Australia": [ // { "name": "Melbourne", "country": "Australia" }, // { "name": "Sydney", "country": "Australia" }, // { "name": "Brisbane", "country": "Australia" } // ], // "New Zealand": [ // { "name": "Auckland", "country": "New Zealand" }, // { "name": "Wellington", "country": "New Zealand" } // ] // }
const double = (x) => x * 2; const increment = (x) => x + 1; const square = (x) => x * x; const result = 5 |> double |> increment |> square; // Output: 121
但是,此更改可能会破坏依赖较旧的非标准导入 JSON 方式的代码,或者某些构建工具配置有较旧的行为。
以上是JavaScript ES 中令人难以置信的新特性(4)的详细内容。更多信息请关注PHP中文网其他相关文章!
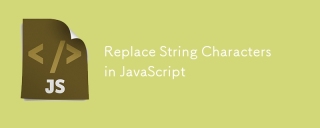
JavaScript字符串替换方法详解及常见问题解答 本文将探讨两种在JavaScript中替换字符串字符的方法:在JavaScript代码内部替换和在网页HTML内部替换。 在JavaScript代码内部替换字符串 最直接的方法是使用replace()方法: str = str.replace("find","replace"); 该方法仅替换第一个匹配项。要替换所有匹配项,需使用正则表达式并添加全局标志g: str = str.replace(/fi
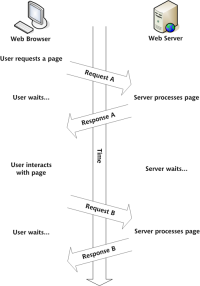
因此,在这里,您准备好了解所有称为Ajax的东西。但是,到底是什么? AJAX一词是指用于创建动态,交互式Web内容的一系列宽松的技术。 Ajax一词,最初由Jesse J创造
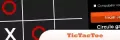
10款趣味横生的jQuery游戏插件,让您的网站更具吸引力,提升用户粘性!虽然Flash仍然是开发休闲网页游戏的最佳软件,但jQuery也能创造出令人惊喜的效果,虽然无法与纯动作Flash游戏媲美,但在某些情况下,您也能在浏览器中获得意想不到的乐趣。 jQuery井字棋游戏 游戏编程的“Hello world”,现在有了jQuery版本。 源码 jQuery疯狂填词游戏 这是一个填空游戏,由于不知道单词的上下文,可能会产生一些古怪的结果。 源码 jQuery扫雷游戏
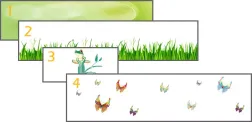
本教程演示了如何使用jQuery创建迷人的视差背景效果。 我们将构建一个带有分层图像的标题横幅,从而创造出令人惊叹的视觉深度。 更新的插件可与JQuery 1.6.4及更高版本一起使用。 下载

本文讨论了在浏览器中优化JavaScript性能的策略,重点是减少执行时间并最大程度地减少对页面负载速度的影响。
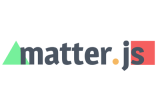
Matter.js是一个用JavaScript编写的2D刚体物理引擎。此库可以帮助您轻松地在浏览器中模拟2D物理。它提供了许多功能,例如创建刚体并为其分配质量、面积或密度等物理属性的能力。您还可以模拟不同类型的碰撞和力,例如重力摩擦力。 Matter.js支持所有主流浏览器。此外,它也适用于移动设备,因为它可以检测触摸并具有响应能力。所有这些功能都使其值得您投入时间学习如何使用该引擎,因为这样您就可以轻松创建基于物理的2D游戏或模拟。在本教程中,我将介绍此库的基础知识,包括其安装和用法,并提供一
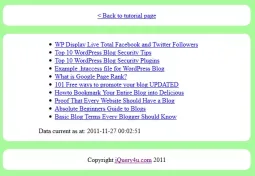
本文演示了如何使用jQuery和ajax自动每5秒自动刷新DIV的内容。 该示例从RSS提要中获取并显示了最新的博客文章以及最后的刷新时间戳。 加载图像是选择


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

Atom编辑器mac版下载
最流行的的开源编辑器
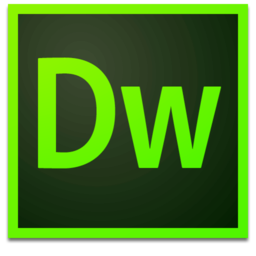
Dreamweaver Mac版
视觉化网页开发工具
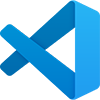
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。
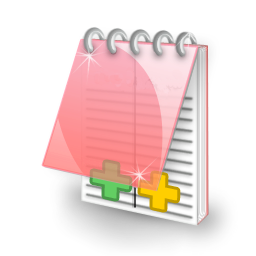
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能