根据数据绘制多色线段
要将数据点可视化为一条线,我们可以使用 matplotlib。在这里,我们有两个列表,“latt”和“lont”,分别表示纬度和经度坐标。目标是绘制一条连接数据点的线,每段 10 个点分配一个唯一的颜色。
方法 1:单独的线图
对于一个小线段的数量,可以为每个线段创建具有不同颜色的单独线图。以下示例代码演示了这种方法:
<code class="python">import numpy as np import matplotlib.pyplot as plt # Assume the list of latitude and longitude is provided # Generate uniqueish colors def uniqueish_color(): return plt.cm.gist_ncar(np.random.random()) # Create a plot fig, ax = plt.subplots() # Iterate through the data in segments of 10 for start, stop in zip(latt[:-1], latt[1:]): # Extract coordinates for each segment x = latt[start:stop] y = lont[start:stop] # Plot each segment with a unique color ax.plot(x, y, color=uniqueish_color()) # Display the plot plt.show()</code>
方法 2:大型数据集的线集合
对于涉及大量线段的大型数据集,使用 Line收藏可以提高效率。下面是一个示例:
<code class="python">import numpy as np import matplotlib.pyplot as plt from matplotlib.collections import LineCollection # Prepare the data as a sequence of line segments segments = np.hstack([latt[:-1], latt[1:]]).reshape(-1, 1, 2) # Create a plot fig, ax = plt.subplots() # Create a LineCollection object coll = LineCollection(segments, cmap=plt.cm.gist_ncar) # Assign random colors to the segments coll.set_array(np.random.random(latt.shape[0])) # Add the LineCollection to the plot ax.add_collection(coll) ax.autoscale_view() # Display the plot plt.show()</code>
总之,两种方法都可以有效地为不同数据点段绘制不同颜色的线条。选择取决于要绘制的线段的数量。
以上是如何使用 Python 从数据中绘制多色线段?的详细内容。更多信息请关注PHP中文网其他相关文章!
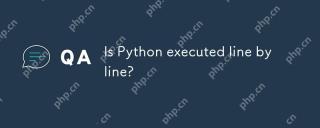
Python不是严格的逐行执行,而是基于解释器的机制进行优化和条件执行。解释器将代码转换为字节码,由PVM执行,可能会预编译常量表达式或优化循环。理解这些机制有助于优化代码和提高效率。
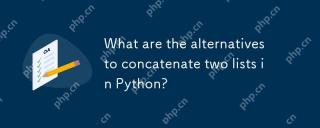
可以使用多种方法在Python中连接两个列表:1.使用 操作符,简单但在大列表中效率低;2.使用extend方法,效率高但会修改原列表;3.使用 =操作符,兼具效率和可读性;4.使用itertools.chain函数,内存效率高但需额外导入;5.使用列表解析,优雅但可能过于复杂。选择方法应根据代码上下文和需求。
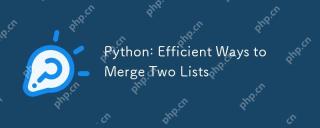
有多种方法可以合并Python列表:1.使用 操作符,简单但对大列表不内存高效;2.使用extend方法,内存高效但会修改原列表;3.使用itertools.chain,适用于大数据集;4.使用*操作符,一行代码合并小到中型列表;5.使用numpy.concatenate,适用于大数据集和性能要求高的场景;6.使用append方法,适用于小列表但效率低。选择方法时需考虑列表大小和应用场景。
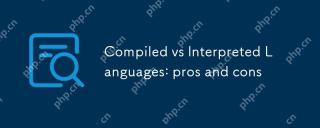
CompiledLanguagesOffersPeedAndSecurity,而interneterpretledlanguages provideeaseafuseanDoctability.1)commiledlanguageslikec arefasterandSecureButhOnderDevevelmendeclementCyclesclesclesclesclesclesclesclesclesclesclesclesclesclesclesclesclesclesandentency.2)cransportedeplatectentysenty
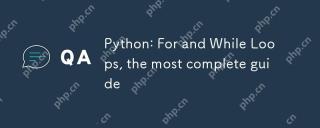
Python中,for循环用于遍历可迭代对象,while循环用于条件满足时重复执行操作。1)for循环示例:遍历列表并打印元素。2)while循环示例:猜数字游戏,直到猜对为止。掌握循环原理和优化技巧可提高代码效率和可靠性。
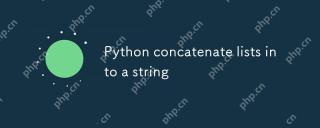
要将列表连接成字符串,Python中使用join()方法是最佳选择。1)使用join()方法将列表元素连接成字符串,如''.join(my_list)。2)对于包含数字的列表,先用map(str,numbers)转换为字符串再连接。3)可以使用生成器表达式进行复杂格式化,如','.join(f'({fruit})'forfruitinfruits)。4)处理混合数据类型时,使用map(str,mixed_list)确保所有元素可转换为字符串。5)对于大型列表,使用''.join(large_li
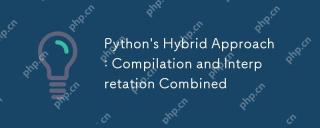
pythonuseshybridapprace,ComminingCompilationTobyTecoDeAndInterpretation.1)codeiscompiledtoplatform-Indepententbybytecode.2)bytecodeisisterpretedbybythepbybythepythonvirtualmachine,增强效率和通用性。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。

禅工作室 13.0.1
功能强大的PHP集成开发环境
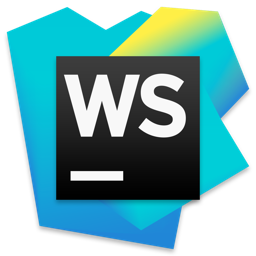
WebStorm Mac版
好用的JavaScript开发工具

SublimeText3汉化版
中文版,非常好用