不必照顾上下文类型的技巧很简单!
如果您使用上下文 API,那么一个问题就是其类型的照顾。
另一个是必须使用多个导入才能在需要时使用它。
通过这个示例,我们解决了这两个问题,并使 React Context API 的使用变得快速、简单。
复制粘贴示例
复制、粘贴,然后将所有“示例”替换为您需要命名的内容,然后就可以开始了。
(之后会有完整评论版本。)
import { createContext, useCallback, useContext, useDeferredValue, useMemo, useState, } from 'react'; function useContextValue(init: number = 0) { const [state, setState] = useState(init); const doubleValue = state * 2; const defferedStringValue = useDeferredValue(state.toString()); const reset = useCallback(() => { setState(init); }, []); const value = useMemo( () => ({ state, doubleValue, defferedStringValue, reset, }), [ state, doubleValue, defferedStringValue, reset, ], ); return value; } type ExampleContext = ReturnType<typeof usecontextvalue>; const Context = createContext<examplecontext>(null!); Context.displayName = 'ExampleContext'; export function ExampleContextProvider({ children, initValue = 0, }: { children: React.ReactNode; initValue?: number; }) { const value = useContextValue(initValue); return <context.provider value="{value}">{children}</context.provider>; } export function useExample() { const value = useContext(Context); if (!value) { throw new Error('useExample must be used within a ExampleContextProvider'); } return value; } </examplecontext></typeof>
评论版
import { createContext, useCallback, useContext, useDeferredValue, useMemo, useState, } from 'react'; /** * We create a custom hook that will have everything * that would usually be in the context main function * * this way, we can use the value it returns to infer the * type of the context */ function useContextValue(init: number = 0) { // do whatever you want inside const [state, setState] = useState(init); const doubleValue = state * 2; const defferedStringValue = useDeferredValue(state.toString()); // remember to memoize functions const reset = useCallback(() => { setState(init); }, []); // and also memoize the final value const value = useMemo( () => ({ state, doubleValue, defferedStringValue, reset, }), [ state, doubleValue, defferedStringValue, reset, ], ); return value; } /** * Since we can infer from the hook, * no need to create the context type by hand */ type ExampleContext = ReturnType<typeof usecontextvalue>; const Context = createContext<examplecontext>(null!); Context.displayName = 'ExampleContext'; export function ExampleContextProvider({ children, /** * this is optional, but it's always a good to remember * that the context is still a react component * and can receive values other than just the children */ initValue = 0, }: { children: React.ReactNode; initValue?: number; }) { const value = useContextValue(initValue); return <context.provider value="{value}">{children}</context.provider>; } /** * We also export a hook that will use the context * * this way, we can use it in other components * by importing just this one hook */ export function useExample() { const value = useContext(Context); /** * this will throw an error if the context * is not used within the provider * * this also avoid the context being "undefined" */ if (!value) { throw new Error('useExample must be used within a ExampleProvider'); } return value; } </examplecontext></typeof>
最后的话
就是这样。 Context API 比应有的更简单、更精细,但对于需要使用它的情况来说,它是一个强大的工具。
请记住,React Context API 不是 Redux(或其他状态管理器),您不应该将整个应用程序状态放入其中。
嗯,可以,但这可能会导致不必要的问题。
这是用 React 编写的
以上是完全类型化、简单的 React Context API 示例的详细内容。更多信息请关注PHP中文网其他相关文章!
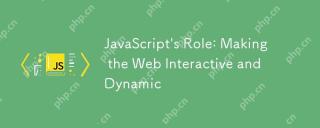
JavaScript是现代网站的核心,因为它增强了网页的交互性和动态性。1)它允许在不刷新页面的情况下改变内容,2)通过DOMAPI操作网页,3)支持复杂的交互效果如动画和拖放,4)优化性能和最佳实践提高用户体验。
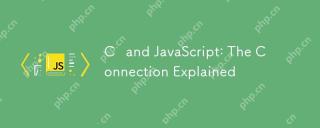
C 和JavaScript通过WebAssembly实现互操作性。1)C 代码编译成WebAssembly模块,引入到JavaScript环境中,增强计算能力。2)在游戏开发中,C 处理物理引擎和图形渲染,JavaScript负责游戏逻辑和用户界面。
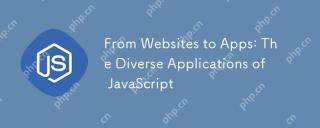
JavaScript在网站、移动应用、桌面应用和服务器端编程中均有广泛应用。1)在网站开发中,JavaScript与HTML、CSS一起操作DOM,实现动态效果,并支持如jQuery、React等框架。2)通过ReactNative和Ionic,JavaScript用于开发跨平台移动应用。3)Electron框架使JavaScript能构建桌面应用。4)Node.js让JavaScript在服务器端运行,支持高并发请求。
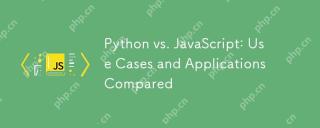
Python更适合数据科学和自动化,JavaScript更适合前端和全栈开发。1.Python在数据科学和机器学习中表现出色,使用NumPy、Pandas等库进行数据处理和建模。2.Python在自动化和脚本编写方面简洁高效。3.JavaScript在前端开发中不可或缺,用于构建动态网页和单页面应用。4.JavaScript通过Node.js在后端开发中发挥作用,支持全栈开发。
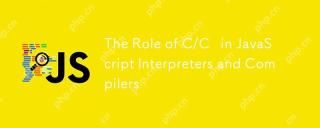
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
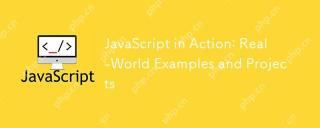
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
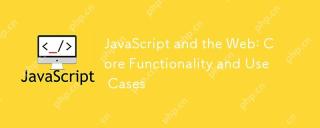
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
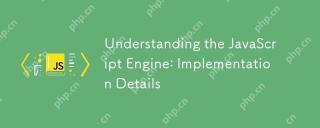
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。
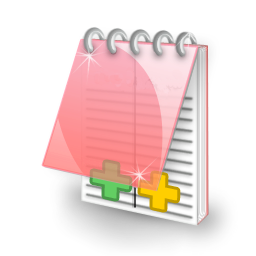
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能
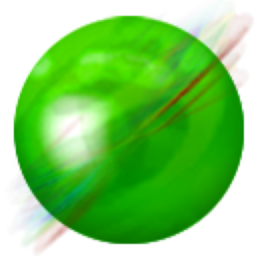
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。
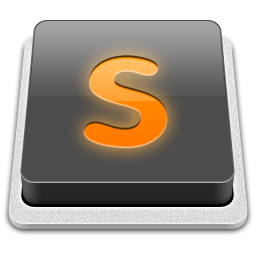
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)