使用标准输入测试 Go 应用程序
在 Go 中,测试从标准输入读取的应用程序可能具有挑战性。考虑一个将 stdin 输入回显到 stdout 的简单应用程序。虽然看起来很简单,但编写验证输出的测试用例可能会带来困难。
尝试失败
最初的方法是使用管道模拟 stdin 和 stdout并手动写入标准输入管道。但是,这可能会导致竞争条件和意外失败。
解决方案:提取逻辑并测试独立函数
而不是使用 stdin 和 在 main 函数中执行所有操作stdout,创建一个单独的函数,接受 io.Reader 和 io.Writer 作为参数。这种方法允许主函数调用该函数,而测试函数直接测试它。
重构代码
<code class="go">package main import ( "bufio" "fmt" "io" ) // Echo takes an io.Reader and an io.Writer and echoes input to output. func Echo(r io.Reader, w io.Writer) { reader := bufio.NewReader(r) for { fmt.Print("> ") bytes, _, _ := reader.ReadLine() if bytes == nil { break } fmt.Fprintln(w, string(bytes)) } } func main() { Echo(os.Stdin, os.Stdout) }</code>
更新测试用例
<code class="go">package main import ( "bufio" "bytes" "io" "os" "testing" ) func TestEcho(t *testing.T) { input := "abc\n" reader := bytes.NewBufferString(input) writer := &bytes.Buffer{} Echo(reader, writer) actual := writer.String() if actual != input { t.Errorf("Wanted: %v, Got: %v", input, actual) } }</code>
这个测试用例通过直接调用 Echo 函数来模拟 main 函数,并使用一个用于 stdin 输入的缓冲区和一个用于捕获输出的缓冲区。然后将捕获的输出与预期输入进行比较,确保函数正确回显输入。
以上是如何测试从 Stdin 读取的 Go 应用程序?的详细内容。更多信息请关注PHP中文网其他相关文章!
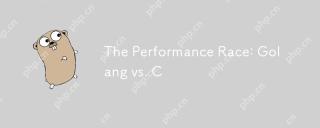
Golang和C 在性能竞赛中的表现各有优势:1)Golang适合高并发和快速开发,2)C 提供更高性能和细粒度控制。选择应基于项目需求和团队技术栈。
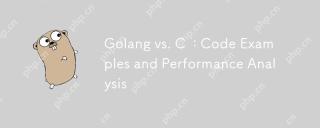
Golang适合快速开发和并发编程,而C 更适合需要极致性能和底层控制的项目。1)Golang的并发模型通过goroutine和channel简化并发编程。2)C 的模板编程提供泛型代码和性能优化。3)Golang的垃圾回收方便但可能影响性能,C 的内存管理复杂但控制精细。
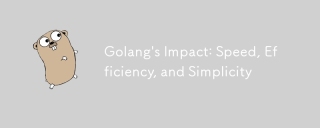
GoimpactsdevelopmentPositationalityThroughSpeed,效率和模拟性。1)速度:gocompilesquicklyandrunseff,ifealforlargeprojects.2)效率:效率:ITScomprehenSevestAndArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增强开发的简单性:3)SimpleflovelmentIcties:3)简单性。
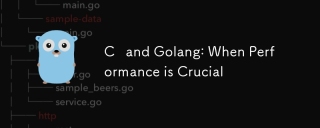
C 更适合需要直接控制硬件资源和高性能优化的场景,而Golang更适合需要快速开发和高并发处理的场景。1.C 的优势在于其接近硬件的特性和高度的优化能力,适合游戏开发等高性能需求。2.Golang的优势在于其简洁的语法和天然的并发支持,适合高并发服务开发。
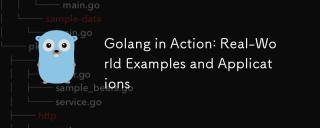
Golang在实际应用中表现出色,以简洁、高效和并发性着称。 1)通过Goroutines和Channels实现并发编程,2)利用接口和多态编写灵活代码,3)使用net/http包简化网络编程,4)构建高效并发爬虫,5)通过工具和最佳实践进行调试和优化。
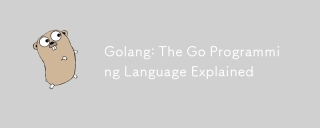
Go语言的核心特性包括垃圾回收、静态链接和并发支持。1.Go语言的并发模型通过goroutine和channel实现高效并发编程。2.接口和多态性通过实现接口方法,使得不同类型可以统一处理。3.基本用法展示了函数定义和调用的高效性。4.高级用法中,切片提供了动态调整大小的强大功能。5.常见错误如竞态条件可以通过gotest-race检测并解决。6.性能优化通过sync.Pool重用对象,减少垃圾回收压力。
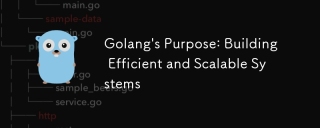
Go语言在构建高效且可扩展的系统中表现出色,其优势包括:1.高性能:编译成机器码,运行速度快;2.并发编程:通过goroutines和channels简化多任务处理;3.简洁性:语法简洁,降低学习和维护成本;4.跨平台:支持跨平台编译,方便部署。
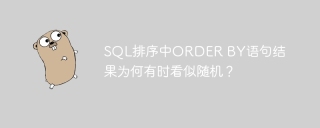
关于SQL查询结果排序的疑惑学习SQL的过程中,常常会遇到一些令人困惑的问题。最近,笔者在阅读《MICK-SQL基础�...


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具
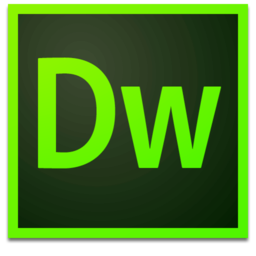
Dreamweaver Mac版
视觉化网页开发工具
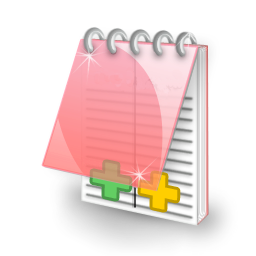
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

Atom编辑器mac版下载
最流行的的开源编辑器
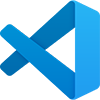
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器
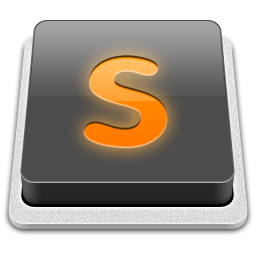
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)