解决 Go 并发中的 Goroutine 死锁
在并发 Go 程序中,当 Goroutine 无限期地等待对方释放资源时,可能会出现死锁。要解决此类死锁,请考虑以下示例:
<code class="go">// Create a channel for communication between goroutines. ch := make(chan int) // Start producer goroutines that send values to the channel. go producer(ch, 100*time.Millisecond, 2) go producer(ch, 200*time.Millisecond, 5) // Indefinite loop to continuously receive values from the channel. for { fmt.Println(<p>此代码会导致死锁错误:“致命错误:所有 goroutine 都在睡眠 - 死锁!”发生这种情况是因为生产者的生命周期有限,最终会停止传输,而消费者 goroutine 则无休止地等待新值。为了避免这种僵局,可以采用两种主要策略:</p> <p><strong>1。通道终止:</strong></p> <p>由于通道只能关闭一次,因此生产者向消费者发出终止信号至关重要。协调员可以监控生产者的完成情况并相应地关闭通道。</p> <p><strong>2.协调同步:</strong></p> <p>使用像sync.WaitGroup这样的同步原语,生产者可以在完成时通知协调者,协调者可以在所有生产者完成后关闭通道。</p> <p> <strong>使用同步更新代码:</strong></p> <pre class="brush:php;toolbar:false"><code class="go">import ( "fmt" "sync" "time" ) func producer(ch chan int, d time.Duration, num int, wg *sync.WaitGroup) { defer wg.Done() for i := 0; i <p><strong>结论:</strong></p> <p>通过实现通道终止或协调同步,开发人员可以有效避免 goroutine 死锁,确保并发 Go 程序中的适当协调。</p></code>
以上是## 如何避免并发Go程序中的死锁?的详细内容。更多信息请关注PHP中文网其他相关文章!
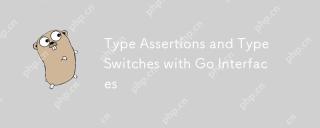
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
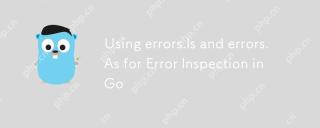
Go语言的错误处理通过errors.Is和errors.As函数变得更加灵活和可读。1.errors.Is用于检查错误是否与指定错误相同,适用于错误链的处理。2.errors.As不仅能检查错误类型,还能将错误转换为具体类型,方便提取错误信息。使用这些函数可以简化错误处理逻辑,但需注意错误链的正确传递和避免过度依赖以防代码复杂化。
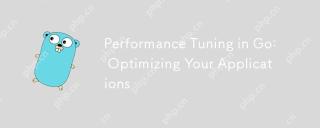
tomakegoapplicationsRunfasterandMorefly,useProflingTools,leverageConCurrency,andManageMoryfectily.1)usepprofforcpuorforcpuandmemoryproflingtoidentifybottlenecks.2)upitizegorizegoroutizegoroutinesandchannelstoparalletaparelalyizetasksandimproverperformance.3)
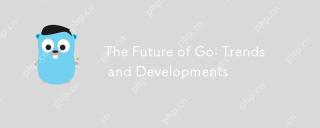
go'sfutureisbrightwithtrendslikeMprikeMprikeTooling,仿制药,云 - 纳蒂维德象,performanceEnhancements,andwebassemblyIntegration,butchallengeSinclainSinClainSinClainSiNgeNingsImpliCityInsImplicityAndimimprovingingRornhandRornrorlling。
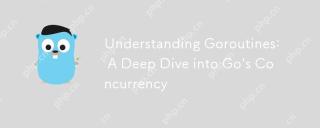
goroutinesarefunctionsormethodsthatruncurranceingo,启用效率和灯威量。1)shememanagedbodo'sruntimemultimusingmultiplexing,允许千sstorunonfewerosthreads.2)goroutinessimproverentimensImproutinesImproutinesImproveranceThroutinesImproveranceThrountinesimproveranceThroundinesImproveranceThroughEasySytaskParallowalizationAndeff
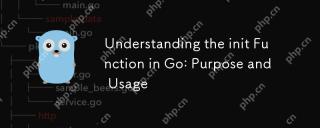
purposeoftheInitfunctionoIsistoInitializeVariables,setUpConfigurations,orperformneccesSetarySetupBeforEtheMainFunctionExeCutes.useInitby.UseInitby:1)placingitinyourcodetorunautoamenationally oneraty oneraty oneraty on inity in ofideShortAndAndAndAndForemain,2)keepitiTshortAntAndFocusedonSimImimpletasks,3)
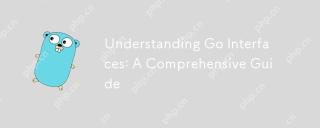
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
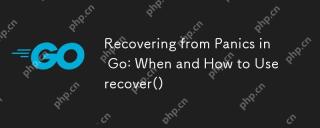
在Go中使用recover()函数可以从panic中恢复。具体方法是:1)在defer函数中使用recover()捕获panic,避免程序崩溃;2)记录详细的错误信息以便调试;3)根据具体情况决定是否恢复程序执行;4)谨慎使用,以免影响性能。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
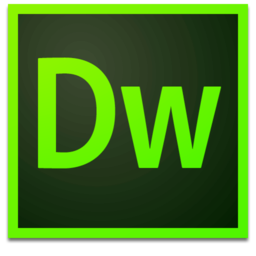
Dreamweaver Mac版
视觉化网页开发工具
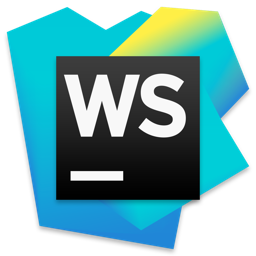
WebStorm Mac版
好用的JavaScript开发工具

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。
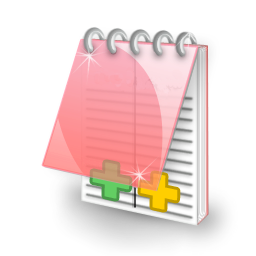
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。