使用正则表达式从字符串中提取双精度浮点值
本文将探讨编程中经常遇到的一个问题:如何提取双精度浮点值使用正则表达式的 Python re 模块从文本字符串中获取数字。
双精度的正则表达式模式
要匹配双精度浮点值,我们可以使用捕获的正则表达式可选符号、整数或小数部分以及可选指数。以下模式是 Perl 文档中的示例:
<code class="python">re_float = re.compile("""(?x) ^ [+-]?\ * # optional sign and space ( # integer or fractional mantissa: \d+ # start out with digits... ( \.\d* # mantissa of the form a.b or a. )? # ? for integers of the form a |\.\d+ # mantissa of the form .b ) ([eE][+-]?\d+)? # optional exponent $""")</code>
匹配和提取
要将双精度值与此模式匹配,我们可以在已编译的正则表达式上使用 match 方法object:
<code class="python">m = re_float.match("4.5") print(m.group(0)) # prints 4.5</code>
这会提取字符串中与模式匹配的部分,在本例中为整个字符串。
提取多个值
如果我们有一个包含多个双精度值的较大字符串,我们可以使用 findall 方法提取所有匹配值:
<code class="python">s = """4.5 abc -4.5 abc - 4.5 abc + .1e10 abc . abc 1.01e-2 abc 1.01e-.2 abc 123 abc .123""" print(re.findall(r"[+-]? *(?:\d+(?:\.\d*)?|\.\d+)(?:[eE][+-]?\d+)?", s)) # prints ['4.5', '-4.5', '- 4.5', '+ .1e10', ' 1.01e-2', # ' 1.01', '-.2', ' 123', ' .123']</code>
此模式匹配任何双精度浮点值,无论空格或周围文本如何,并将其提取作为字符串列表。
以上是如何使用正则表达式从字符串中提取双精度浮点值?的详细内容。更多信息请关注PHP中文网其他相关文章!
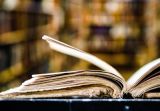
本教程演示如何使用Python处理Zipf定律这一统计概念,并展示Python在处理该定律时读取和排序大型文本文件的效率。 您可能想知道Zipf分布这个术语是什么意思。要理解这个术语,我们首先需要定义Zipf定律。别担心,我会尽量简化说明。 Zipf定律 Zipf定律简单来说就是:在一个大型自然语言语料库中,最频繁出现的词的出现频率大约是第二频繁词的两倍,是第三频繁词的三倍,是第四频繁词的四倍,以此类推。 让我们来看一个例子。如果您查看美国英语的Brown语料库,您会注意到最频繁出现的词是“th
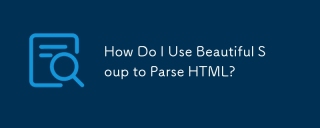
本文解释了如何使用美丽的汤库来解析html。 它详细介绍了常见方法,例如find(),find_all(),select()和get_text(),以用于数据提取,处理不同的HTML结构和错误以及替代方案(SEL)
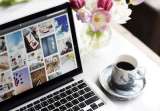
处理嘈杂的图像是一个常见的问题,尤其是手机或低分辨率摄像头照片。 本教程使用OpenCV探索Python中的图像过滤技术来解决此问题。 图像过滤:功能强大的工具 图像过滤器
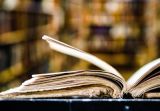
PDF 文件因其跨平台兼容性而广受欢迎,内容和布局在不同操作系统、阅读设备和软件上保持一致。然而,与 Python 处理纯文本文件不同,PDF 文件是二进制文件,结构更复杂,包含字体、颜色和图像等元素。 幸运的是,借助 Python 的外部模块,处理 PDF 文件并非难事。本文将使用 PyPDF2 模块演示如何打开 PDF 文件、打印页面和提取文本。关于 PDF 文件的创建和编辑,请参考我的另一篇教程。 准备工作 核心在于使用外部模块 PyPDF2。首先,使用 pip 安装它: pip 是 P

本教程演示了如何利用Redis缓存以提高Python应用程序的性能,特别是在Django框架内。 我们将介绍REDIS安装,Django配置和性能比较,以突出显示BENE
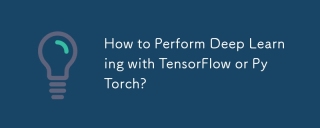
本文比较了Tensorflow和Pytorch的深度学习。 它详细介绍了所涉及的步骤:数据准备,模型构建,培训,评估和部署。 框架之间的关键差异,特别是关于计算刻度的
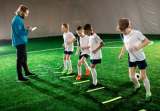
Python是数据科学和处理的最爱,为高性能计算提供了丰富的生态系统。但是,Python中的并行编程提出了独特的挑战。本教程探讨了这些挑战,重点是全球解释
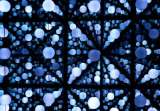
本教程演示了在Python 3中创建自定义管道数据结构,利用类和操作员超载以增强功能。 管道的灵活性在于它能够将一系列函数应用于数据集的能力,GE


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具
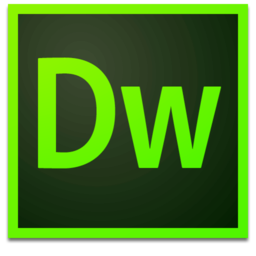
Dreamweaver Mac版
视觉化网页开发工具

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。