When learning Java or any object-oriented programming (OOP) language, two essential concepts stand out—Encapsulation and Abstraction. These concepts are key pillars of OOP that promote code reusability, security, and maintainability. Although they are often used together, they serve distinct purposes.
In this post, we'll dive deep into the differences between encapsulation and abstraction, with clear definitions, examples, and code snippets to help you understand their role in Java programming. Let's break it down!
What is Encapsulation?
Encapsulation is the process of bundling data (variables) and methods that operate on the data into a single unit, typically a class. It hides the internal state of an object from the outside world, only allowing controlled access through public methods.
Key Features of Encapsulation:
- Data hiding: Internal object data is hidden from other classes.
- Access control: Only the allowed (public) methods can manipulate the hidden data.
- Improves security: Prevents external code from modifying internal data directly.
- Easy maintenance: If the internal implementation changes, only the methods need to be updated, not the external classes.
Example of Encapsulation in Java:
// Encapsulation in action public class Employee { // Private variables (data hiding) private String name; private int age; // Getter and setter methods (controlled access) public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } } // Using the encapsulated class public class Main { public static void main(String[] args) { Employee emp = new Employee(); emp.setName("John Doe"); emp.setAge(30); System.out.println("Employee Name: " + emp.getName()); System.out.println("Employee Age: " + emp.getAge()); } }
In this example, the Employee class hides its fields (name and age) by declaring them private. External classes like Main can only access these fields via getter and setter methods, which control and validate the input/output.
What is Abstraction?
Abstraction refers to the concept of hiding the complex implementation details of an object and exposing only the essential features. This simplifies the interaction with objects and makes the code more user-friendly.
Key Features of Abstraction:
- Hides complexity: Users only see what they need, and the underlying code is hidden.
- Focus on 'what' rather than 'how': Provides only the necessary details to the user while keeping implementation hidden.
- Helps in managing complexity: Useful for working with complex systems by providing simplified interfaces.
- Enforced via interfaces and abstract classes: These constructs provide a blueprint without exposing implementation.
Example of Abstraction in Java:
// Abstract class showcasing abstraction abstract class Animal { // Abstract method (no implementation) public abstract void sound(); // Concrete method public void sleep() { System.out.println("Sleeping..."); } } // Subclass providing implementation for abstract method class Dog extends Animal { public void sound() { System.out.println("Barks"); } } public class Main { public static void main(String[] args) { Animal dog = new Dog(); dog.sound(); // Calls the implementation of the Dog class dog.sleep(); // Calls the common method in the Animal class } }
Here, the abstract class Animal contains an abstract method sound() which must be implemented by its subclasses. The Dog class provides its own implementation for sound(). This way, the user doesn't need to worry about how the sound() method works internally—they just call it.
Encapsulation vs. Abstraction: Key Differences
Now that we’ve seen the definitions and examples, let’s highlight the key differences between encapsulation and abstraction in Java:
Feature | Encapsulation | Abstraction |
---|---|---|
Purpose | Data hiding and protecting internal state | Simplifying code by hiding complex details |
Focus | Controls access to data using getters/setters | Provides essential features and hides implementation |
Implementation | Achieved using classes with private fields | Achieved using abstract classes and interfaces |
Role in OOP | Increases security and maintains control over data | Simplifies interaction with complex systems |
Example | Private variables and public methods | Abstract methods and interfaces |
Java 的实际用例
何时使用封装:
- 当您需要保护数据时:例如,在银行系统中不应直接修改帐户余额。
- 当您想要控制数据的访问方式时:确保只有允许的方法才能修改或检索数据,从而添加一层安全性。
何时使用抽象:
- 在大型系统上工作时:在各种类和模块交互的大型项目中,抽象可以通过提供简化的接口来帮助管理复杂性。
- 开发 API 时:仅向用户公开必要的细节,同时隐藏实际的实现。
封装和抽象相结合的好处
尽管封装和抽象服务于不同的目的,但它们一起工作以在 Java 中构建健壮、安全且可维护的代码。
- 安全性和灵活性:通过将两者结合起来,您可以确保数据受到保护(封装),同时仍然允许用户以简单的方式与其交互(抽象)。
- 代码可维护性:抽象隐藏了复杂性,使系统更易于管理,而封装提供了对数据的受控访问。
- 可重用性:这两个概念都促进代码重用——通过隔离数据进行封装,通过允许抽象方法的不同实现来进行抽象。
结论:掌握 Java 中的封装和抽象
封装和抽象是面向对象编程中的两个强大概念,每个 Java 开发人员都应该掌握。 封装通过控制数据访问来帮助保护对象的内部状态,抽象隐藏了系统的复杂性并仅提供必要的细节。
通过理解和应用两者,您可以构建经得起时间考验的安全、可维护和可扩展的应用程序。
本指南是否帮助您阐明 Java 中的封装和抽象?在下面的评论中分享您的想法或问题!
标签:
- #Java
- #OOP
- #封装
- #抽象
- #Java编程
以上是Java 中的封装与抽象:终极指南的详细内容。更多信息请关注PHP中文网其他相关文章!
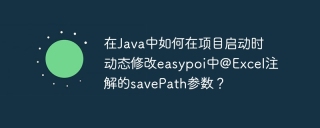
在Java中如何动态配置实体类注解的参数在开发过程中,我们经常会遇到需要根据不同环境动态配置注解参数的�...
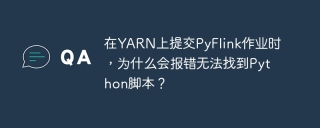
在YARN上提交PyFlink作业时报错无法找到Python脚本的原因分析当你尝试通过YARN提交一个PyFlink作业时,可能会遇到�...
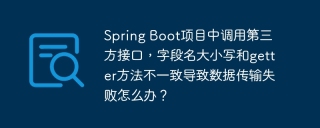
在SpringBoot项目中调用第三方接口传输数据时遇到的难题本文将针对一个Spring...
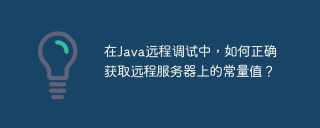
Java远程调试中常量获取的疑问解答在使用Java进行远程调试时,许多开发者可能会遇到一些难以理解的现象。其�...

探讨后端开发中的分层架构在后端开发中,分层架构是一种常见的设计模式,通常包括controller、service和dao三层�...


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。
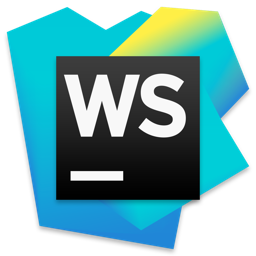
WebStorm Mac版
好用的JavaScript开发工具
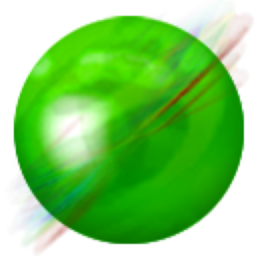
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。