在本文中,我们将解决 Perl 每周挑战 #288 中的两个任务:找到最接近的回文并确定矩阵中最大连续块的大小。这两种解决方案都将在 Perl 和 Go 中递归实现。
目录
- 最近的回文
- 连续块
- 结论
最近的回文
第一个任务是找到最接近的不包含自身的回文。
最接近的回文被定义为最小化两个整数之间的绝对差的回文。
如果有多个候选者,则应返回最小的一个。
任务描述
输入: 字符串 $str,代表整数。
输出: 最接近的回文字符串。
示例
输入:“123”
输出:“121”输入: "2"
输出:“1”
有两个最接近的回文:“1”和“3”。因此,我们返回最小的“1”。输入:“1400”
输出:“1441”输入:“1001”
输出:“999”
解决方案
Perl 实现
在此实现中,我们利用递归方法来查找不等于原始数字的最接近的回文。递归函数探索原始数字的下限和上限:
- 它检查当前候选(下级和上级)是否是有效的回文(并且不等于原始)。
- 如果两个候选都无效,该函数会递归地递减较低的候选并递增较高的候选,直到找到有效的回文。
这种递归策略有效地缩小了搜索空间,确保我们在遵守问题约束的同时识别最接近的回文。
sub is_palindrome { my ($num) = @_; return $num eq reverse($num); } sub find_closest { my ($lower, $upper, $original) = @_; return $lower if is_palindrome($lower) && $lower != $original; return $upper if is_palindrome($upper) && $upper != $original; return find_closest($lower - 1, $upper + 1, $original) if $lower > 0; return $upper + 1; } sub closest_palindrome { my ($str) = @_; my $num = int($str); return find_closest($num - 1, $num + 1, $num); }
实施
Go 实现遵循类似的递归策略。它还检查原始数字周围的候选数,使用递归来调整边界,直到找到有效的回文数。
package main import ( "strconv" ) func isPalindrome(num int) bool { reversed := 0 original := num for num > 0 { digit := num % 10 reversed = reversed*10 + digit num /= 10 } return original == reversed } func findClosest(lower, upper, original int) string { switch { case isPalindrome(lower) && lower != original: return strconv.Itoa(lower) case isPalindrome(upper) && upper != original: return strconv.Itoa(upper) case lower > 0: return findClosest(lower-1, upper+1, original) default: return strconv.Itoa(upper + 1) } } func closestPalindrome(str string) string { num, _ := strconv.Atoi(str) return findClosest(num-1, num+1, num) }
Hier ist die erweiterte Definition für den 连续块:
连续块
第二个任务是确定给定矩阵中最大连续块的大小,其中所有单元格都包含 x 或 o。
连续块由包含相同符号的元素组成,这些元素与块中的其他元素共享边缘(不仅仅是角),从而创建一个连接区域。
任务描述
输入: 包含 x 和 o 的矩形矩阵。
输出:最大连续块的大小。
示例
-
输入:
[ ['x', 'x', 'x', 'x', 'o'], ['x', 'o', 'o', 'o', 'o'], ['x', 'o', 'o', 'o', 'o'], ['x', 'x', 'x', 'o', 'o'], ]
输出: 11
有一个包含 x 的 9 个连续单元格的块和一个包含 o 的 11 个连续单元格的块。
-
输入:
[ ['x', 'x', 'x', 'x', 'x'], ['x', 'o', 'o', 'o', 'o'], ['x', 'x', 'x', 'x', 'o'], ['x', 'o', 'o', 'o', 'o'], ]
输出: 11
有一个包含 x 的 11 个连续单元格的块和一个包含 o 的 9 个连续单元格的块。
-
输入:
[ ['x', 'x', 'x', 'o', 'o'], ['o', 'o', 'o', 'x', 'x'], ['o', 'x', 'x', 'o', 'o'], ['o', 'o', 'o', 'x', 'x'], ]
输出: 7
有一个包含 o 的 7 个连续单元格块、另外两个包含 o 的 2 单元格块、三个包含 x 的 2 单元格块和一个包含 x 的 3 单元格块。
解决方案
Perl 实现
在此实现中,我们利用递归深度优先搜索(DFS)方法来确定矩阵中最大连续块的大小。主函数初始化一个访问矩阵来跟踪哪些单元已被探索。它迭代每个单元格,每当遇到未访问的单元格时调用递归 DFS 函数。
DFS 函数探索当前单元格的所有四个可能的方向(上、下、左、右)。它通过在共享相同符号且尚未被访问的相邻单元上递归调用自身来计算连续块的大小。这种递归方法有效地聚合了块的大小,同时确保每个单元仅被计数一次。
sub largest_contiguous_block { my ($matrix) = @_; my $rows = @$matrix; my $cols = @{$matrix->[0]}; my @visited = map { [(0) x $cols] } 1..$rows; my $max_size = 0; for my $r (0 .. $rows - 1) { for my $c (0 .. $cols - 1) { my $symbol = $matrix->[$r][$c]; my $size = dfs($matrix, \@visited, $r, $c, $symbol); $max_size = $size if $size > $max_size; } } return $max_size; } sub dfs { my ($matrix, $visited, $row, $col, $symbol) = @_; return 0 if $row = @$matrix || $col = @{$matrix->[0]} || $visited->[$row][$col] || $matrix->[$row][$col] ne $symbol; $visited->[$row][$col] = 1; my $count = 1; $count += dfs($matrix, $visited, $row + 1, $col, $symbol); $count += dfs($matrix, $visited, $row - 1, $col, $symbol); $count += dfs($matrix, $visited, $row, $col + 1, $symbol); $count += dfs($matrix, $visited, $row, $col - 1, $symbol); return $count; }
实施
Go 实现反映了这种递归 DFS 策略。它类似地遍历矩阵并使用递归来探索具有相同符号的连续单元格。
package main func largestContiguousBlock(matrix [][]rune) int { rows := len(matrix) if rows == 0 { return 0 } cols := len(matrix[0]) visited := make([][]bool, rows) for i := range visited { visited[i] = make([]bool, cols) } maxSize := 0 for r := 0; r maxSize { maxSize = size } } } return maxSize } func dfs(matrix [][]rune, visited [][]bool, row, col int, symbol rune) int { if row = len(matrix) || col = len(matrix[0]) || visited[row][col] || matrix[row][col] != symbol { return 0 } visited[row][col] = true count := 1 count += dfs(matrix, visited, row+1, col, symbol) count += dfs(matrix, visited, row-1, col, symbol) count += dfs(matrix, visited, row, col+1, symbol) count += dfs(matrix, visited, row, col-1, symbol) return count }
Conclusion
In this article, we explored two intriguing challenges from the Perl Weekly Challenge #288: finding the closest palindrome and determining the size of the largest contiguous block in a matrix.
For the first task, both the Perl and Go implementations effectively utilized recursion to navigate around the original number, ensuring the closest palindrome was found efficiently.
In the second task, the recursive depth-first search approach in both languages allowed for a thorough exploration of the matrix, resulting in an accurate count of the largest contiguous block of identical symbols.
These challenges highlight the versatility of recursion as a powerful tool in solving algorithmic problems, showcasing its effectiveness in both Perl and Go. If you're interested in further exploration or have any questions, feel free to reach out!
You can find the complete code, including tests, on GitHub.
以上是深入研究:回文和连续块的递归解决方案的详细内容。更多信息请关注PHP中文网其他相关文章!
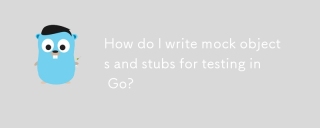
本文演示了创建模拟和存根进行单元测试。 它强调使用接口,提供模拟实现的示例,并讨论最佳实践,例如保持模拟集中并使用断言库。 文章
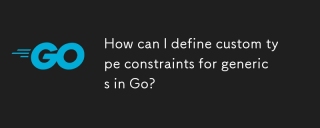
本文探讨了GO的仿制药自定义类型约束。 它详细介绍了界面如何定义通用功能的最低类型要求,从而改善了类型的安全性和代码可重复使用性。 本文还讨论了局限性和最佳实践
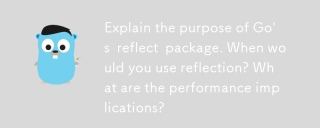
本文讨论了GO的反思软件包,用于运行时操作代码,对序列化,通用编程等有益。它警告性能成本,例如较慢的执行和更高的内存使用,建议明智的使用和最佳
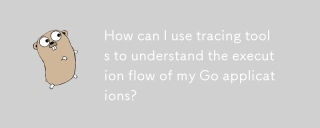
本文使用跟踪工具探讨了GO应用程序执行流。 它讨论了手册和自动仪器技术,比较诸如Jaeger,Zipkin和Opentelemetry之类的工具,并突出显示有效的数据可视化
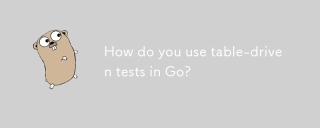
本文讨论了GO中使用表驱动的测试,该方法使用测试用例表来测试具有多个输入和结果的功能。它突出了诸如提高的可读性,降低重复,可伸缩性,一致性和A
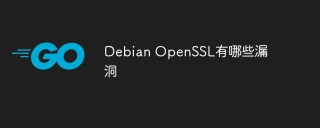
OpenSSL,作为广泛应用于安全通信的开源库,提供了加密算法、密钥和证书管理等功能。然而,其历史版本中存在一些已知安全漏洞,其中一些危害极大。本文将重点介绍Debian系统中OpenSSL的常见漏洞及应对措施。DebianOpenSSL已知漏洞:OpenSSL曾出现过多个严重漏洞,例如:心脏出血漏洞(CVE-2014-0160):该漏洞影响OpenSSL1.0.1至1.0.1f以及1.0.2至1.0.2beta版本。攻击者可利用此漏洞未经授权读取服务器上的敏感信息,包括加密密钥等。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

SublimeText3 英文版
推荐:为Win版本,支持代码提示!
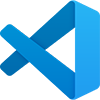
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。
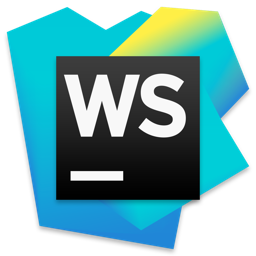
WebStorm Mac版
好用的JavaScript开发工具
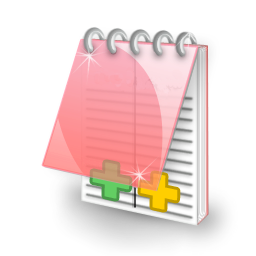
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能