当然!针对高流量网站优化 PHP 需要一种涵盖代码质量、数据库管理、缓存、服务器配置等方面的综合方法。下面是针对高流量网站优化 PHP 的详细规则列表,并在适用的情况下提供了实践示例。
1. 使用操作码缓存
规则:启用 OPcache 缓存预编译的 PHP 代码。
示例:
; Enable OPcache in php.ini opcache.enable=1 opcache.memory_consumption=128 opcache.interned_strings_buffer=8 opcache.max_accelerated_files=10000 opcache.revalidate_freq=60
2. 优化数据库查询
规则:使用索引列并避免 SELECT 语句中不必要的列。
示例:
-- Instead of SELECT * SELECT id, name, price FROM products WHERE category_id = 1;
3. 实现数据缓存
规则: 使用 Memcached 缓存经常访问的数据。
示例:
$memcached = new Memcached(); $memcached->addServer('localhost', 11211); $key = 'products_list'; $products = $memcached->get($key); if ($products === FALSE) { $products = get_products_from_database(); // Fetch from DB $memcached->set($key, $products, 600); // Cache for 10 minutes }
4. 使用持久连接
规则:使用持久数据库连接来减少连接开销。
示例:
$pdo = new PDO('mysql:host=localhost;dbname=test', 'user', 'password', [ PDO::ATTR_PERSISTENT => true ]);
5. 减少文件 I/O 操作
规则:最小化文件系统读/写。
示例:
// Avoid repeated file reads $settings = include('config.php'); // Cache this in a variable if used multiple times
6. 优化PHP配置
规则:调整 php.ini 设置以获得更好的性能。
示例:
memory_limit=256M max_execution_time=30
7. 使用自动加载器
规则:使用 Composer 的自动加载器进行高效的类加载。
示例:
require 'vendor/autoload.php'; // Composer's autoloader // Use classes $object = new MyClass();
8. 实施负载均衡
规则:跨多个服务器分配流量。
示例:
- 配置 Nginx 进行负载均衡:
http { upstream backend { server backend1.example.com; server backend2.example.com; } server { location / { proxy_pass http://backend; } } }
9. 使用异步处理
规则: 将任务卸载到后台进程。
示例:
// Using a queue system like Redis $redis = new Redis(); $redis->connect('localhost'); $redis->rPush('email_queue', json_encode($emailData)); // Worker process to handle email sending $emailData = json_decode($redis->lPop('email_queue'), true); send_email($emailData);
10. 最小化依赖
规则:仅包含必要的库和依赖项。
示例:
composer install --no-dev // Install production dependencies only
11. 优化循环和算法
规则:避免低效的循环和算法。
示例:
// Instead of inefficient loops foreach ($items as $item) { // Process item } // Use optimized algorithms and data structures $items = array_map('processItem', $items);
12. 使用高效的数据结构
规则:根据您的需求选择适当的数据结构。
示例:
// Using associative arrays for quick lookups $data = ['key1' => 'value1', 'key2' => 'value2']; $value = $data['key1'];
13. 优化会话处理
规则:使用高效的会话存储。
示例:
; Use Redis for session storage session.save_handler = redis session.save_path = "tcp://localhost:6379"
14. 使用 HTTP/2
规则: 利用 HTTP/2 获得更好的性能。
示例:
- 在 Nginx 中配置 HTTP/2:
server { listen 443 ssl http2; # Other SSL configuration }
15. 实现 Gzip 压缩
规则:压缩响应以减少带宽。
示例:
- 在 Nginx 中启用 Gzip:
http { gzip on; gzip_types text/plain text/css application/json application/javascript; }
16. 最小化前端资产大小
规则: 优化 CSS、JavaScript 和图像文件。
示例:
# Minify CSS and JS files uglifyjs script.js -o script.min.js
17.使用内容分发网络(CDN)
规则: 将静态内容卸载到 CDN。
示例:
- 为静态资产配置CDN:
<link rel="stylesheet" href="https://cdn.example.com/styles.css"> <script src="https://cdn.example.com/scripts.js"></script>
18. 启用错误日志
规则:有效记录错误以进行调试。
示例:
; Log errors to a file error_log = /var/log/php_errors.log log_errors = On
19. 监控性能
规则:使用监控工具来跟踪性能。
示例:
- 安装并配置 New Relic:
# Install New Relic PHP agent sudo newrelic-install install # Configure New Relic in php.ini newrelic.enabled = true
20. 定期分析和基准测试
规则:不断分析和基准测试您的应用程序。
示例:
- 使用 Xdebug 分析 PHP 脚本:
# Install Xdebug sudo pecl install xdebug # Enable Xdebug profiling in php.ini xdebug.profiler_enable = 1 xdebug.profiler_output_dir = "/tmp/xdebug"
通过遵循这些规则并实现提供的示例,您可以显着增强基于 PHP 的高流量网站的性能和可扩展性。
以上是针对高流量网站优化 PHP 的规则的详细内容。更多信息请关注PHP中文网其他相关文章!
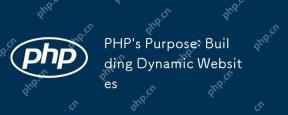
PHP用于构建动态网站,其核心功能包括:1.生成动态内容,通过与数据库对接实时生成网页;2.处理用户交互和表单提交,验证输入并响应操作;3.管理会话和用户认证,提供个性化体验;4.优化性能和遵循最佳实践,提升网站效率和安全性。

PHP在数据库操作和服务器端逻辑处理中使用MySQLi和PDO扩展进行数据库交互,并通过会话管理等功能处理服务器端逻辑。1)使用MySQLi或PDO连接数据库,执行SQL查询。2)通过会话管理等功能处理HTTP请求和用户状态。3)使用事务确保数据库操作的原子性。4)防止SQL注入,使用异常处理和关闭连接来调试。5)通过索引和缓存优化性能,编写可读性高的代码并进行错误处理。
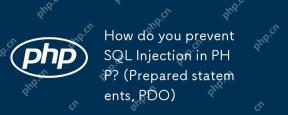
在PHP中使用预处理语句和PDO可以有效防范SQL注入攻击。1)使用PDO连接数据库并设置错误模式。2)通过prepare方法创建预处理语句,使用占位符和execute方法传递数据。3)处理查询结果并确保代码的安全性和性能。
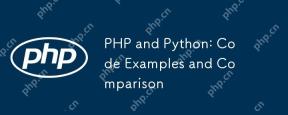
PHP和Python各有优劣,选择取决于项目需求和个人偏好。1.PHP适合快速开发和维护大型Web应用。2.Python在数据科学和机器学习领域占据主导地位。
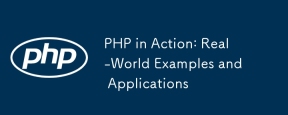
PHP在电子商务、内容管理系统和API开发中广泛应用。1)电子商务:用于购物车功能和支付处理。2)内容管理系统:用于动态内容生成和用户管理。3)API开发:用于RESTfulAPI开发和API安全性。通过性能优化和最佳实践,PHP应用的效率和可维护性得以提升。
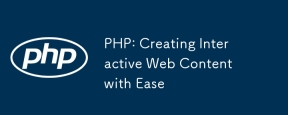
PHP可以轻松创建互动网页内容。1)通过嵌入HTML动态生成内容,根据用户输入或数据库数据实时展示。2)处理表单提交并生成动态输出,确保使用htmlspecialchars防XSS。3)结合MySQL创建用户注册系统,使用password_hash和预处理语句增强安全性。掌握这些技巧将提升Web开发效率。
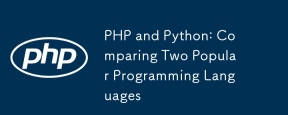
PHP和Python各有优势,选择依据项目需求。1.PHP适合web开发,尤其快速开发和维护网站。2.Python适用于数据科学、机器学习和人工智能,语法简洁,适合初学者。
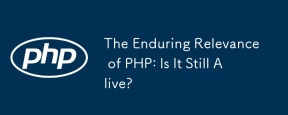
PHP仍然具有活力,其在现代编程领域中依然占据重要地位。1)PHP的简单易学和强大社区支持使其在Web开发中广泛应用;2)其灵活性和稳定性使其在处理Web表单、数据库操作和文件处理等方面表现出色;3)PHP不断进化和优化,适用于初学者和经验丰富的开发者。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。

禅工作室 13.0.1
功能强大的PHP集成开发环境

SublimeText3 英文版
推荐:为Win版本,支持代码提示!

SublimeText3汉化版
中文版,非常好用
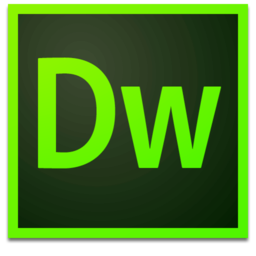
Dreamweaver Mac版
视觉化网页开发工具