在 Web 开发领域,了解请求生命周期对于优化性能、调试问题和构建强大的应用程序至关重要。在 Django(一种流行的 Python Web 框架)中,请求生命周期是一个明确定义的步骤序列,从服务器收到请求到将响应发送回客户端,该请求所经历的步骤。
这篇博客文章对 Django 请求生命周期进行了广泛的检查。我们将引导您完成该过程的每个阶段,为您提供代码示例,并为您提供有关如何调整和提高 Django 应用程序性能的提示和建议。通过本文的结束,您将对 Django 的请求和响应处理有一个全面的了解。
- Django 请求生命周期简介
在深入研究请求生命周期的细节之前,有必要了解 Web 开发背景下的请求是什么。请求是由客户端(通常是 Web 浏览器)发送到服务器的 HTTP 消息,请求特定资源或操作。服务器处理请求并发回 HTTP 响应,该响应可以是网页、图像或 JSON 格式的数据。
Django 是一个高级 Python Web 框架,抽象了处理 HTTP 请求和响应的大部分复杂性。然而,了解 Django 如何处理这些请求的底层机制对于想要充分利用该框架的全部功能的开发人员来说非常宝贵。
- Django 请求的剖析
Django 请求的核心是 HttpRequest 类的实例。当服务器收到请求时,Django 会创建一个 HttpRequest 对象,其中包含有关该请求的元数据,例如:
方法:使用的 HTTP 方法(GET、POST、PUT、DELETE 等)。
Path:请求的URL路径。
Headers:包含HTTP标头的字典,例如User-Agent、Host等
Body:请求的正文,可能包含表单数据、JSON负载等
这是在 Django 视图中访问其中一些属性的简单示例:
from django.http import HttpResponse def example_view(request): method = request.method path = request.path user_agent = request.headers.get('User-Agent', '') response_content = f"Method: {method}, Path: {path}, User-Agent: {user_agent}" return HttpResponse(response_content)
在此示例中,example_view 是一个基本的 Django 视图,它从请求中提取 HTTP 方法、路径和用户代理,并在响应中返回它们。
- Django 请求生命周期的逐步分解
让我们详细探讨 Django 请求生命周期的每一步:
第 1 步:URL 路由
当请求到达 Django 服务器时,第一步是 URL 路由。 Django 使用 URL 调度程序将传入请求的路径与 urls.py 文件中定义的预定义 URL 模式列表进行匹配。
# urls.py from django.urls import path from .views import example_view urlpatterns = [ path('example/', example_view, name='example'), ]
在此示例中,任何路径为 /example/ 的请求都将被路由到 example_view 函数。
如果 Django 找到匹配的 URL 模式,它会调用关联的视图函数。如果未找到匹配项,Django 将返回 404 Not Found 响应。
第 2 步:中间件处理
在视图执行之前,Django通过一系列中间件处理请求。中间件是允许开发人员全局处理请求和响应的钩子。它们可用于各种目的,例如身份验证、日志记录或修改请求/响应。
这是一个记录请求方法和路径的自定义中间件示例:
# middleware.py class LogRequestMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): # Process the request print(f"Request Method: {request.method}, Path: {request.path}") response = self.get_response(request) # Process the response return response
要使用此中间件,请将其添加到settings.py文件中的MIDDLEWARE列表中:
# settings.py MIDDLEWARE = [ 'django.middleware.security.SecurityMiddleware', 'django.contrib.sessions.middleware.SessionMiddleware', # Add your custom middleware here 'myapp.middleware.LogRequestMiddleware', 'django.middleware.common.CommonMiddleware', 'django.middleware.csrf.CsrfViewMiddleware', 'django.contrib.auth.middleware.AuthenticationMiddleware', 'django.contrib.messages.middleware.MessageMiddleware', 'django.middleware.clickjacking.XFrameOptionsMiddleware', ]
中间件按照中间件列表中列出的顺序进行处理。请求会经过列表中的每个中间件,直到到达视图。
第 3 步:查看执行情况
一旦请求通过了所有中间件,Django 就会调用与匹配的 URL 模式关联的视图。视图是应用程序的核心逻辑所在的地方。它负责处理请求、与模型和数据库交互并返回响应。
这是与数据库交互的 Django 视图示例:
# views.py from django.shortcuts import render from .models import Product def product_list(request): products = Product.objects.all() return render(request, 'product_list.html', {'products': products})
在此示例中,product_list 视图查询 Product 模型以从数据库中检索所有产品,并将它们传递到 Product_list.html 模板进行渲染。
第四步:模板渲染
如果视图直接返回 HttpResponse 对象,Django 会跳过模板渲染步骤。但是,如果视图返回上下文数据字典,Django 将使用模板引擎来呈现 HTML 响应。
这是一个简单的 Django 模板示例:
<!-- templates/product_list.html --> <title>Product List</title> <h1 id="Products">Products</h1>
-
{% for product in products %}
- {{ product.name }} - ${{ product.price }} {% endfor %}
在此示例中,product_list.html 模板循环遍历产品上下文变量,并在无序列表中呈现每个产品的名称和价格。
第 5 步:生成响应
After the view has processed the request and rendered the template (if applicable), Django generates an HttpResponse object. This object contains the HTTP status code, headers, and content of the response.
Here's an example of manually creating an HttpResponse object:
from django.http import HttpResponse def custom_response_view(request): response = HttpResponse("Hello, Django!") response.status_code = 200 response['Content-Type'] = 'text/plain' return response
In this example, the custom_response_view function returns a plain text response with a status code of 200 (OK).
Step 6: Middleware Response Processing
Before the response is sent back to the client, it passes through the middleware again. This time, Django processes the response through any middleware that has a process_response method.
This is useful for tasks such as setting cookies, compressing content, or adding custom headers. Here’s an example of a middleware that adds a custom header to the response:
# middleware.py class CustomHeaderMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): response = self.get_response(request) response['X-Custom-Header'] = 'MyCustomHeaderValue' return response
Step 7: Sending the Response
Finally, after all middleware processing is complete, Django sends the HttpResponse object back to the client. The client receives the response and renders the content (if it’s a web page) or processes it further (if it’s an API response).
- Advanced Topics in Django Request Handling
Now that we’ve covered the basics of the Django request life cycle, let's explore some advanced topics:
4.1 Custom Middleware
Creating custom middleware allows you to hook into the request/response life cycle and add custom functionality globally. Here’s an example of a middleware that checks for a custom header and rejects requests that do not include it:
# middleware.py from django.http import HttpResponseForbidden class RequireCustomHeaderMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): if 'X-Required-Header' not in request.headers: return HttpResponseForbidden("Forbidden: Missing required header") response = self.get_response(request) return response
4.2 Request and Response Objects
Django's HttpRequest and HttpResponse objects are highly customizable. You can subclass these objects to add custom behavior. Here’s an example of a custom request class that adds a method for checking if the request is coming from a mobile device:
# custom_request.py from django.http import HttpRequest class CustomHttpRequest(HttpRequest): def is_mobile(self): user_agent = self.headers.get('User-Agent', '').lower() return 'mobile' in user_agent
To use this custom request class, you need to set it in the settings.py file:
# settings.py MIDDLEWARE = [ 'django.middleware.security.SecurityMiddleware', 'django.contrib.sessions.middleware.SessionMiddleware', 'django.middleware.common.Common Middleware', # Use your custom request class 'myapp.custom_request.CustomHttpRequest', 'django.middleware.csrf.CsrfViewMiddleware', 'django.contrib.auth.middleware.AuthenticationMiddleware', 'django.contrib.messages.middleware.MessageMiddleware', 'django.middleware.clickjacking.XFrameOptionsMiddleware', ]
4.3 Optimizing the Request Life Cycle
Optimizing the request life cycle can significantly improve your Django application's performance. Here are some tips:
Use Caching: Caching can drastically reduce the load on your server by storing frequently accessed data in memory. Django provides a robust caching framework that supports multiple backends, such as Memcached and Redis.
# views.py from django.views.decorators.cache import cache_page @cache_page(60 * 15) # Cache the view for 15 minutes def my_view(request): # View logic here return HttpResponse("Hello, Django!")
Minimize Database Queries: Use Django’s select_related and prefetch_related methods to minimize the number of database queries.
# views.py from django.shortcuts import render from .models import Author def author_list(request): # Use select_related to reduce database queries authors = Author.objects.select_related('profile').all() return render(request, 'author_list.html', {'authors': authors})
Leverage Middleware for Global Changes: Instead of modifying each view individually, use middleware to make global changes. This can include setting security headers, handling exceptions, or modifying the request/response.
Asynchronous Views: Starting with Django 3.1, you can write asynchronous views to handle requests asynchronously. This can improve performance for I/O-bound tasks such as making external API calls or processing large files.
# views.py from django.http import JsonResponse import asyncio async def async_view(request): await asyncio.sleep(1) # Simulate a long-running task return JsonResponse({'message': 'Hello, Django!'})
- Conclusion
Understanding the Django request life cycle is fundamental for any Django developer. By knowing how requests are processed, you can write more efficient, maintainable, and scalable applications. This guide has walked you through each step of the request life cycle, from URL routing to sending the response, and provided code examples and tips for optimizing your Django applications.
By leveraging the power of Django’s middleware, request and response objects, and caching framework, you can build robust web applications that perform well under load and provide a great user experience.
References
Django Documentation: https://docs.djangoproject.com/en/stable/
Django Middleware: https://docs.djangoproject.com/en/stable/topics/http/middleware/
Django Views: https://docs.djangoproject.com/en/stable/topics/http/views/
Django Templates: https://docs.djangoproject.com/en/stable/topics/templates/
Django Caching: https://docs.djangoproject.com/en/stable/topics/cache/
以上是Django 请求生命周期解释的详细内容。更多信息请关注PHP中文网其他相关文章!
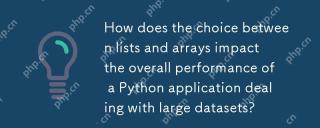
ForhandlinglargedatasetsinPython,useNumPyarraysforbetterperformance.1)NumPyarraysarememory-efficientandfasterfornumericaloperations.2)Avoidunnecessarytypeconversions.3)Leveragevectorizationforreducedtimecomplexity.4)Managememoryusagewithefficientdata
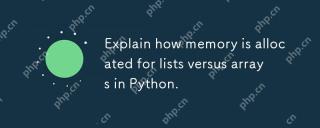
Inpython,ListSusedynamicMemoryAllocationWithOver-Asalose,而alenumpyArraySallaySallocateFixedMemory.1)listssallocatemoremoremoremorythanneededinentientary上,respizeTized.2)numpyarsallaysallaysallocateAllocateAllocateAlcocateExactMemoryForements,OfferingPrediCtableSageButlessemageButlesseflextlessibility。
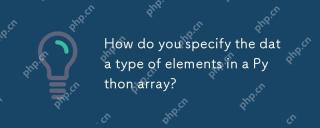
Inpython,YouCansspecthedatatAtatatPeyFelemereModeRernSpant.1)Usenpynernrump.1)Usenpynyp.dloatp.dloatp.ploatm64,formor professisconsiscontrolatatypes。
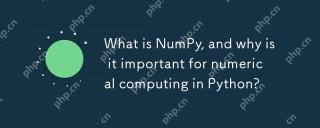
NumPyisessentialfornumericalcomputinginPythonduetoitsspeed,memoryefficiency,andcomprehensivemathematicalfunctions.1)It'sfastbecauseitperformsoperationsinC.2)NumPyarraysaremorememory-efficientthanPythonlists.3)Itoffersawiderangeofmathematicaloperation
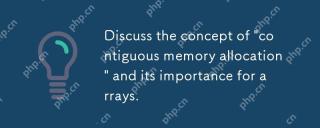
Contiguousmemoryallocationiscrucialforarraysbecauseitallowsforefficientandfastelementaccess.1)Itenablesconstanttimeaccess,O(1),duetodirectaddresscalculation.2)Itimprovescacheefficiencybyallowingmultipleelementfetchespercacheline.3)Itsimplifiesmemorym
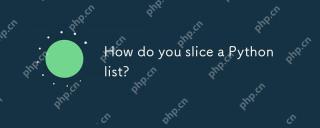
SlicingaPythonlistisdoneusingthesyntaxlist[start:stop:step].Here'showitworks:1)Startistheindexofthefirstelementtoinclude.2)Stopistheindexofthefirstelementtoexclude.3)Stepistheincrementbetweenelements.It'susefulforextractingportionsoflistsandcanuseneg

numpyallowsforvariousoperationsonArrays:1)basicarithmeticlikeaddition,减法,乘法和division; 2)evationAperationssuchasmatrixmultiplication; 3)element-wiseOperations wiseOperationswithOutexpliitloops; 4)
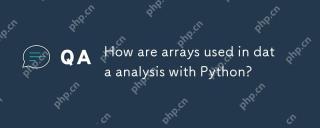
Arresinpython,尤其是Throughnumpyandpandas,weessentialFordataAnalysis,offeringSpeedAndeffied.1)NumpyArseNable efflaysenable efficefliceHandlingAtaSetSetSetSetSetSetSetSetSetSetSetsetSetSetSetSetsopplexoperationslikemovingaverages.2)


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SublimeText3 英文版
推荐:为Win版本,支持代码提示!
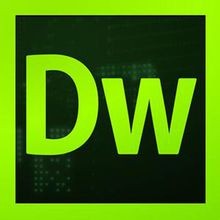
Dreamweaver CS6
视觉化网页开发工具
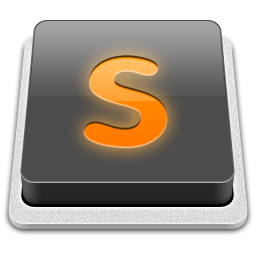
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

SublimeText3 Linux新版
SublimeText3 Linux最新版
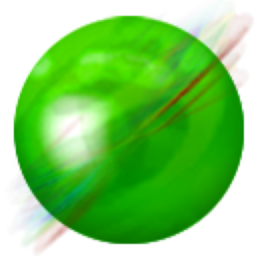
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境