Graal 编译器是动态即时 (JIT) 编译技术的根本性飞跃。 JIT 编译在 Java 虚拟机 (JVM) 架构中的作用和功能被誉为 Java 令人印象深刻的性能背后的一个重要因素,由于其复杂且相当不透明的性质,常常让许多从业者感到困惑。
什么是 JIT 编译器?
当您执行 javac 命令或使用 IDE 时,您的 Java 程序将从 Java 源代码转换为 JVM 字节码。这个
该过程创建 Java 程序的二进制表示 - 一种比原始源代码更简单、更紧凑的格式。
但是,计算机或服务器中的经典处理器无法直接执行 JVM 字节码。这需要 JVM 来解释字节码。
图 1 – 即时 (JIT) 编译器的工作原理
与在实际处理器上运行的本机代码相比,解释器的性能通常较差,这促使 JVM 在运行时调用另一个编译器 - JIT 编译器。 JIT 编译器将字节码转换为处理器可以直接运行的机器代码。这个复杂的编译器执行一系列高级优化来生成高质量的机器代码。
该字节码充当中间层,使 Java 应用程序能够在具有不同处理器架构的各种操作系统上运行。 JVM本身就是一个软件程序,它逐条指令地解释这个字节码。
Graal JIT 编译器——用 Java 编写
JVM 的 OpenJDK 实现包含两个传统的 JIT 编译器 - 客户端编译器 (C1) 和服务器编译器(C2 或 Opto)。客户端编译器针对更快的操作和较少优化的代码输出进行了优化,使其成为桌面应用程序的理想选择,因为长时间的 JIT 编译暂停可能会中断用户体验。相反,服务器编译器旨在花费更多时间来生成高度优化的代码,使其适合长时间运行的服务器应用程序。
两个编译器可以通过“分层编译”串联使用。最初,代码通过 C1 编译,如果执行频率证明额外的编译时间合理,则随后通过 C2 编译。
C2 采用 C++ 开发,尽管具有高性能特性,但也有固有的缺点。 C++是一种不安全的语言;因此,C2 模块中的错误可能会导致整个虚拟机崩溃。继承的C++代码的复杂性和刚性也导致其维护性和可扩展性成为重大挑战。
Graal 独有的,这个 JIT 编译器是用 Java 开发的。编译器的主要要求是接受 JVM 字节码并输出机器代码 - 一种不需要 C 或 C++ 等系统级语言的高级操作。
用 Java 编写的 Graal 有几个优点:
提高安全性:Java 的垃圾收集和托管内存方法消除了 JIT 编译器本身与内存相关的崩溃风险。
更容易维护和扩展:Java 代码库更易于开发人员贡献和扩展 JIT 编译器的功能。
可移植性:Java 的平台独立性意味着 Graal JIT 编译器可以在任何具有 Java 虚拟机的平台上工作。
JVM 编译器接口 (JVMCI)
JVM 编译器接口 (JVMCI) 是 JVM 中的一项创新功能和新接口 (JEP 243:https://openjdk.org/jeps/243)。
与 Java 注释处理 API 非常相似,JVMCI 也允许集成自定义 Java JIT 编译器。
JVMCI 接口包含一个从 byte 到 byte[] 的纯函数:
interface JVMCICompiler { byte[] compileMethod(byte[] bytecode); }
这并没有捕捉到现实生活场景的全部复杂性。
在实际应用中,我们经常需要额外的信息,例如局部变量的数量、堆栈大小以及从解释器中分析收集的数据,以更好地了解代码的执行情况。因此,该界面需要更复杂的输入。它不仅仅接受字节码,还接受 CompilationRequest:
public interface JVMCICompiler { int INVOCATION_ENTRY_BCI = -1; CompilationRequestResult compileMethod(CompilationRequest request); }
JVMCICompiler.java
CompilationRequest 封装了更全面的信息,例如哪个 JavaMethod 用于编译,以及编译器可能需要的更多数据。
CompilationRequest.java
This approach has the benefit of providing all necessary details to the custom JIT-compiler in a more organized and contextual manner. To create a new JIT-compiler for the JVM, one must implement the JVMCICompiler interface.
Ideal Graph
An aspect where Graal truly shines in terms of performing sophisticated code optimization is in its use of a unique data structure: the program-dependence-graph, or colloquially, an "Ideal Graph".
The program-dependence-graph is a directed graph that presents a visual representation of the dependencies between individual operations, essentially laying out the matrix of dependencies between different parts of your Java code.
Let's illustrate this concept with a simple example of adding two local variables, x and y. The program-dependence-graph for this operation in Graal's context would involve three nodes and two edges:
-
Nodes:
- Load(x) and Load(y): These nodes represent the operations of loading the values of variables x and y from memory into registers within the processor.
- Add: This node embodies the operation of adding the values loaded from x and y.
-
Edges:
- Two edges would be drawn from the Load(x) and Load(y) nodes to the Add node. These directional paths convey the data flow. They signify that the values loaded from x and y are the inputs to the addition operation.
+--------->+--------->+ | Load(x) | Load(y) | +--------->+--------->+ | v +-----+ | Add | +-----+
In this illustration, the arrows represent the data flow between the nodes. The Load(x) and Load(y) nodes feed their loaded values into the Add node, which performs the addition operation. This visual representation helps Graal identify potential optimizations based on the dependencies between these operations.
This graph-based architecture provides the Graal compiler with a clear visible landscape of dependencies and scheduling in the code it compiles. The program-dependence-graph not only maps the flow of data and relationships between operations but also offers a canvas for Gaal to manipulate these relationships. Each node on the graph is a clear candidate for specific optimizations, while the edges indicate where alterations would propagate changes elsewhere in the code - both aspects influence how Graal optimizes your program's performance.
Visualizing and analyzing this graph can be achieved through a tool called the IdealGraphVisualizer, or IGV. This tool is invaluable in understanding the intricacies of Graal's code optimization capabilities. It allows you to pinpoint how specific parts of your code are being analyzed, modified, and optimized, providing valuable insights for further code enhancements.
Let's consider a simple Java program that performs a complex operation in a loop:
public class Demo { public static void main(String[] args) { for (int i = 0; i <p>When compiled with Graal, the Ideal Graph for this program would look something like this(<strong>Figure 2</strong>).</p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172509339871886.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="Exploring Graal: Next-Generation JIT Compilation for Java"></p> <p><em>Figure 2 – Graal Graphs</em></p> <p>Therefore, along with its method level optimizations and overall code performance improvements, this graph-based representation constitutes the key to understanding the power of the Graal compiler in optimizing your Java applications</p> <h2> In Conclusion </h2> <p>The Graal JIT compiler represents a significant leap forward in Java performance optimization. Its unique characteristic of being written in Java itself offers a compelling alternative to traditional C-based compilers. This not only enhances safety and maintainability but also paves the way for a more dynamic and adaptable JIT compilation landscape.</p> <p>The introduction of the JVM Compiler Interface (JVMCI) further amplifies this potential. By allowing the development of custom JIT compilers in Java, JVMCI opens doors for further experimentation and innovation. This could lead to the creation of specialized compilers targeting specific needs or architectures, ultimately pushing the boundaries of Java performance optimization.</p> <p>In essence, Graal and JVMCI represent a paradigm shift in JIT compilation within the Java ecosystem. They lay the foundation for a future where JIT compilation can be customized, extended, and continuously improved, leading to even more performant and versatile Java applications.</p>
以上是探索 Graal:下一代 Java JIT 编译的详细内容。更多信息请关注PHP中文网其他相关文章!
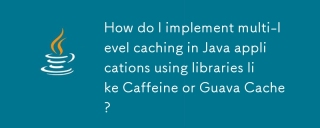
本文讨论了使用咖啡因和Guava缓存在Java中实施多层缓存以提高应用程序性能。它涵盖设置,集成和绩效优势,以及配置和驱逐政策管理最佳PRA
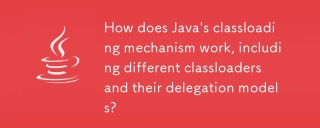
Java的类上载涉及使用带有引导,扩展程序和应用程序类负载器的分层系统加载,链接和初始化类。父代授权模型确保首先加载核心类别,从而影响自定义类LOA
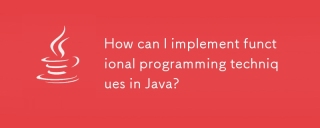
本文使用lambda表达式,流API,方法参考和可选探索将功能编程集成到Java中。 它突出显示了通过简洁性和不变性改善代码可读性和可维护性等好处

本文讨论了使用JPA进行对象相关映射,并具有高级功能,例如缓存和懒惰加载。它涵盖了设置,实体映射和优化性能的最佳实践,同时突出潜在的陷阱。[159个字符]

本文讨论了使用Maven和Gradle进行Java项目管理,构建自动化和依赖性解决方案,以比较其方法和优化策略。

本文使用选择器和频道使用单个线程有效地处理多个连接的Java的NIO API,用于非阻滞I/O。 它详细介绍了过程,好处(可伸缩性,性能)和潜在的陷阱(复杂性,
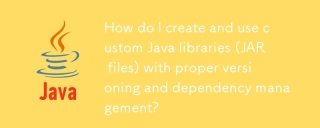
本文使用Maven和Gradle之类的工具讨论了具有适当的版本控制和依赖关系管理的自定义Java库(JAR文件)的创建和使用。
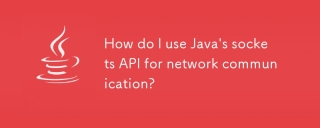
本文详细介绍了用于网络通信的Java的套接字API,涵盖了客户服务器设置,数据处理和关键考虑因素,例如资源管理,错误处理和安全性。 它还探索了性能优化技术,我


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

SublimeText3汉化版
中文版,非常好用

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。

记事本++7.3.1
好用且免费的代码编辑器

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),