JavaScript 是一种支持多种编程范例的通用语言。了解这些范例可以帮助开发人员选择解决不同问题的最佳方法。主要的编程范例包括:
- 命令式:专注于如何执行任务(一步一步)。
- 过程式:类似于命令式,但具有可重用的过程。
- 面向对象:将代码组织成可重用的对象。
- 声明式:重点关注程序应该完成的任务。
- 函数式:将计算视为数学函数(我们今天的明星!)。
在本文中,我们将探索 JavaScript 中的函数式编程,这是一种强调纯函数、高阶函数和不变性的强大范例。
1. 纯函数
纯函数是一种输出值仅由其输入值决定的函数,没有可观察到的副作用。
确定性:对于相同的输入,函数总是产生相同的输出。
无副作用:该函数不会修改任何外部状态(例如全局变量、输入参数)。
示例:
// Pure function function add(a, b) { return a + b; } // Impure function let count = 0; function increment() { count += 1; return count; }
在上面的示例中,add 是一个纯函数,因为它对于相同的输入始终返回相同的结果,并且不会修改任何外部状态。相反,increment是一个不纯函数,因为它修改了外部变量count。
2. 高阶函数
高阶函数是一个可以将其他函数作为参数和/或返回函数作为结果的函数。
函数作为参数:可以接受函数作为输入参数。
函数作为返回值:可以返回函数作为输出。
示例:
// Higher-order function function applyOperation(a, b, operation) { return operation(a, b); } // Function to be used as an argument function multiply(x, y) { return x * y; } // Using the higher-order function const result = applyOperation(5, 3, multiply); // Output: 15
在此示例中,applyOperation 是一个高阶函数,因为它采用函数(操作)作为参数。
3. 不变性
不变性是指数据一旦创建就无法更改的概念。不是修改现有的数据结构,而是创建新的数据结构。
无突变:数据结构在创建后不会更改。
复制和修改:操作创建具有所需更改的新数据结构。
示例:
// Mutable object let user = { name: 'Alice', age: 25 }; user.age = 26; // Mutation // Immutable object using Object.assign const newUser = Object.assign({}, user, { age: 26 }); console.log(newUser); // Output: { name: 'Alice', age: 26 }
在此示例中,我们没有直接修改用户对象,而是使用更新后的年龄创建了一个新对象 newUser。
函数式编程有什么大不了的?
现在,想象一下您正在编写一些代码(请耐心等待,我们将在这里进行完整的比喻)。命令式编程就像做一顿饭,给出一步一步的指令:“切洋葱,然后炒它们,然后添加大蒜......”另一方面,函数式编程就像拥有一个专业厨师团队,每一个都完善了菜肴的一部分。你只需告诉他们你想要什么,瞧!烹饪魔法发生了。
是否曾经感觉你的代码是一团混乱的 for 循环和 if 语句?好吧,系好安全带,因为我们即将踏上 JavaScript 函数式编程 (FP) 世界的神奇之旅。这就像将您的意大利面条代码变成一顿美食! ➡️?
让我们通过一些美味的代码示例来看看这个厨房魔法的实际效果!
为了了解函数式编程的好处,让我们将其与更传统的命令式风格进行比较:
数组转换:开胃菜
命令式(老式厨房):
const veggies = ['carrot', 'broccoli', 'cauliflower']; const cookedVeggies = []; for (let i = 0; i <p>功能风格(现代厨房):<br> </p> <pre class="brush:php;toolbar:false">const veggies = ['carrot', 'broccoli', 'cauliflower']; const cookedVeggies = veggies.map(veggie => `cooked ${veggie}`);
看看我们如何将笨重的 for 循环变成流畅的单行代码?这就是 FP 的美妙之处——就像有一个副主厨(地图)为你做所有重复的工作!
煎饼堆栈逆转:翻转早餐塔
想象一下,您是一名煎饼艺术家,您刚刚制作了一堆高耸的煎饼,每个煎饼上都写着字母。现在您想要翻转整个堆栈以从下到上读取消息。让我们看看如何使用代码来做到这一点!
命令式(老式煎饼脚蹼):
function flipPancakeStack(stack) { let flippedStack = ''; for (let i = stack.length - 1; i >= 0; i--) { flippedStack += stack[i]; } return flippedStack; } const originalStack = "PANCAKE"; const flippedStack = flipPancakeStack(originalStack); console.log(flippedStack); // "EKACNAP"
在这种方法中,我们手动将每个煎饼从顶部到底部逐个翻转。它确实有效,但是有点费力,不是吗?想象一下这样翻转一大堆!
功能型(光滑的煎饼机):
const flipPancakeStack = str => str.split('').reduce((reversed, char) => char + reversed, ''); const originalStack = "PANCAKE"; const flippedStack = flipPancakeStack(originalStack); console.log(flippedStack); // "EKACNAP"
Wow! Look at that smooth operator! ? We've turned our string into an array of characters, then used the reduce function to flip our pancake in one sweeping motion. Here's what's happening:
- split('') turns our string into an array of characters.
- reduce goes through each character, adding it to the front of our accumulating result.
- We start with an empty string '' and build it up, character by character.
It's like having a fancy pancake-flipping robot that assembles the pancake in reverse as it goes along. No manual flipping required!
The Beauty of Functional Flipping
Notice how our functional approach doesn't use any loops or temporary variables. It's a single expression that flows from left to right. This makes it:
- More readable: Once you're used to reduce, this reads almost like English.
- Immutable: We're not changing any existing data, just creating new strings.
- Shorter: We've reduced our function to a single, powerful line.
Remember, in the kitchen of code, it's not just about getting the job done – it's about style, efficiency, and leaving a clean workspace. Our functional pancake flipper does all three!
Main Course: Curry Transformation Feast
Now, let's spice things up with some Indian cuisine! Imagine we're running a bustling Indian restaurant, and we need to transform our thali menu. We want to adjust spice levels, filter out dishes based on dietary preferences, and format the names for our trendy menu board.
Imperative Style (The frazzled curry chef):
const thaliMenu = [ { name: 'Butter Chicken', spiceLevel: 2, vegetarian: false, available: true }, { name: 'Palak Paneer', spiceLevel: 1, vegetarian: true, available: true }, { name: 'Lamb Vindaloo', spiceLevel: 4, vegetarian: false, available: false }, { name: 'Dal Makhani', spiceLevel: 1, vegetarian: true, available: true }, { name: 'Chicken Tikka Masala', spiceLevel: 3, vegetarian: false, available: true } ]; const veggieSpicyMenu = []; for (let i = 0; i 5) dish.spiceLevel = 5; veggieSpicyMenu.push(dish); } }
Functional Style (The Michelin-star tandoor master):
const thaliMenu = [ { name: 'Butter Chicken', spiceLevel: 2, vegetarian: false, available: true }, { name: 'Palak Paneer', spiceLevel: 1, vegetarian: true, available: true }, { name: 'Lamb Vindaloo', spiceLevel: 4, vegetarian: false, available: false }, { name: 'Dal Makhani', spiceLevel: 1, vegetarian: true, available: true }, { name: 'Chicken Tikka Masala', spiceLevel: 3, vegetarian: false, available: true } ]; const veggieSpicyMenu = thaliMenu .filter(dish => dish.vegetarian && dish.available) .map(dish => ({ name: dish.name.toUpperCase().replace(/ /g, '_'), spiceLevel: Math.min(dish.spiceLevel + 1, 5) }));
?✨ We've just transformed our thali menu with the grace of a yoga master. The functional approach reads like a recipe from a classic Indian cookbook: "Filter the vegetarian and available dishes, then map them to new objects with formatted names and increased spice levels." It's a recipe for code that's as aromatic and delightful as the dishes it describes!
Dessert: Async Chai Brewing Symphony
For our final course, let's steep ourselves in the art of asynchronous chai brewing. Imagine we're creating a smart chai maker that needs to check tea leaves, heat water, and blend spices, all in perfect harmony.
Imperative Style (The flustered chai wallah):
function brewChai(teaType, callback) { checkTeaLeaves(teaType) .then(leaves => { if (leaves.quality === 'good') { heatWater(leaves.requiredTemperature) .then(water => { blendSpices(teaType) .then(spices => { const chai = mixChaiIngredients(leaves, water, spices); callback(null, chai); }) .catch(error => callback(error)); }) .catch(error => callback(error)); } else { callback(new Error('Tea leaves are not of good quality')); } }) .catch(error => callback(error)); }
Functional Style (The serene chai master):
const brewChai = teaType => checkTeaLeaves(teaType) .then(leaves => leaves.quality === 'good' ? Promise.all([ Promise.resolve(leaves), heatWater(leaves.requiredTemperature), blendSpices(teaType) ]) : Promise.reject(new Error('Tea leaves are not of good quality')) ) .then(([leaves, water, spices]) => mixChaiIngredients(leaves, water, spices));
Wah, what a beautiful symphony! ?? We've just orchestrated a complex chai brewing process into a smooth, promise-based operation. It's like watching a graceful kathak dance – each step flows seamlessly into the next, creating a perfect blend of flavors and aromas.
The Secret Masala: Why FP is the Chef's Kiss ???
- Readability: FP code often reads like a story. "Filter this, map that, reduce those." It's like writing a recipe for your future self (or your poor colleague who has to maintain your code).
- Predictability: Pure functions always return the same output for a given input. No surprises, no "it worked on my machine" mysteries.
- Testability: Since FP emphasizes pure functions, testing becomes a breeze. It's like being able to taste each ingredient separately before combining them.
- Conciseness: As we've seen, FP can often express complex operations in just a few lines. Less code means fewer bugs and easier maintenance.
- Composition: You can combine simple functions to create complex behaviors, like stacking Lego bricks to build a castle. ?
Wrapping Up Our Functional Feast
There you have it, folks! We've transformed our code from a fast-food joint to a Michelin-star restaurant. Functional programming in JavaScript isn't just about writing less code; it's about writing code that's easier to understand, test, and maintain.
Remember, you don't have to go full Gordon Ramsay and remake your entire codebase overnight. Start small – try using map instead of a for-loop, or break a complex function into smaller, pure functions. Before you know it, you'll be whipping up functional programming delicacies that would make any code chef proud!
Now, go forth and func-tionalize! May your code be pure, your functions be high-order, and your bugs be few.
Happy coding, and may the func be with you! ??
以上是JavaScript - 函数式编程有什么大不了的?的详细内容。更多信息请关注PHP中文网其他相关文章!
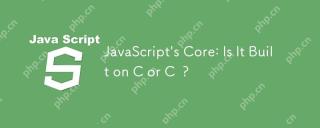
javascriptisnotbuiltoncorc; saninterpretedlanguagethatrunsonenginesoftenwritteninc.1)javascriptwasdesignedAsalightweight,解释edganguageforwebbrowsers.2)Enginesevolvedfromsimpleterterterpretpreterterterpretertestojitcompilerers,典型地提示。
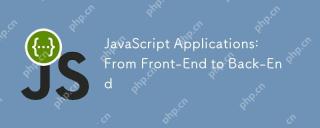
JavaScript可用于前端和后端开发。前端通过DOM操作增强用户体验,后端通过Node.js处理服务器任务。1.前端示例:改变网页文本内容。2.后端示例:创建Node.js服务器。
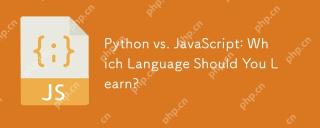
选择Python还是JavaScript应基于职业发展、学习曲线和生态系统:1)职业发展:Python适合数据科学和后端开发,JavaScript适合前端和全栈开发。2)学习曲线:Python语法简洁,适合初学者;JavaScript语法灵活。3)生态系统:Python有丰富的科学计算库,JavaScript有强大的前端框架。
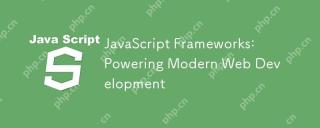
JavaScript框架的强大之处在于简化开发、提升用户体验和应用性能。选择框架时应考虑:1.项目规模和复杂度,2.团队经验,3.生态系统和社区支持。
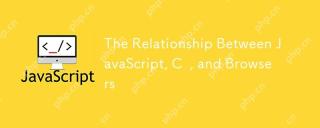
引言我知道你可能会觉得奇怪,JavaScript、C 和浏览器之间到底有什么关系?它们之间看似毫无关联,但实际上,它们在现代网络开发中扮演着非常重要的角色。今天我们就来深入探讨一下这三者之间的紧密联系。通过这篇文章,你将了解到JavaScript如何在浏览器中运行,C 在浏览器引擎中的作用,以及它们如何共同推动网页的渲染和交互。JavaScript与浏览器的关系我们都知道,JavaScript是前端开发的核心语言,它直接在浏览器中运行,让网页变得生动有趣。你是否曾经想过,为什么JavaScr
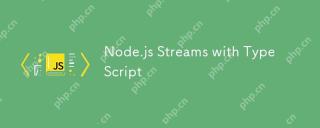
Node.js擅长于高效I/O,这在很大程度上要归功于流。 流媒体汇总处理数据,避免内存过载 - 大型文件,网络任务和实时应用程序的理想。将流与打字稿的类型安全结合起来创建POWE
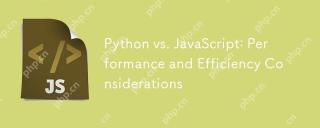
Python和JavaScript在性能和效率方面的差异主要体现在:1)Python作为解释型语言,运行速度较慢,但开发效率高,适合快速原型开发;2)JavaScript在浏览器中受限于单线程,但在Node.js中可利用多线程和异步I/O提升性能,两者在实际项目中各有优势。
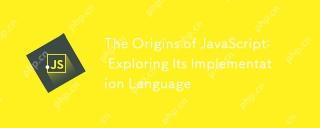
JavaScript起源于1995年,由布兰登·艾克创造,实现语言为C语言。1.C语言为JavaScript提供了高性能和系统级编程能力。2.JavaScript的内存管理和性能优化依赖于C语言。3.C语言的跨平台特性帮助JavaScript在不同操作系统上高效运行。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SublimeText3 Linux新版
SublimeText3 Linux最新版

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。

记事本++7.3.1
好用且免费的代码编辑器

禅工作室 13.0.1
功能强大的PHP集成开发环境

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中