功能齐全的图像库,具有使用 PHP、HTML、jQuery、AJAX、JSON、Bootstrap、CSS 和 MySQL 上传功能。该项目包含详细的分步解决方案以及代码解释和文档,使其成为一个全面的学习教程。
主题:php、mysql、博客、ajax、bootstrap、jquery、css、图片库、文件上传
分步解决方案
1. 目录结构
simple-image-gallery/ │ ├── index.html ├── db/ │ └── database.sql ├── src/ │ ├── fetch-image.php │ └── upload.php ├── include/ │ ├── config.sample.php │ └── db.php ├── assets/ │ ├── css/ │ │ └── style.css │ ├── js/ │ │ └── script.js │ └── uploads/ │ │ └── (uploaded images will be stored here) ├── README.md └── .gitignore
2. 数据库架构
db/database.sql:
CREATE TABLE IF NOT EXISTS `images` ( `id` int(11) NOT NULL AUTO_INCREMENT, `file_name` varchar(255) NOT NULL, `uploaded_on` datetime NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
3. 配置文件
配置设置(include/config.sample.php)
<?php define('DB_HOST', 'localhost'); // Database host define('DB_NAME', 'image_gallery'); // Database name define('DB_USER', 'root'); // Change if necessary define('DB_PASS', ''); // Change if necessary define('UPLOAD_DIRECTORY', '/assets/uploads/'); // Change if necessary ?>
4. 配置数据库连接
建立数据库连接(include/db.php)
<?php include 'config.php'; // Database configuration $dsn = 'mysql:host='.DB_HOST.';dbname='.DB_NAME; $options = [ PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION, PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC, ]; // Create a new PDO instance try { $pdo = new PDO($dsn, DB_USER, DB_PASS, $options); $pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Set error mode to exception } catch (PDOException $e) { echo 'Connection failed: ' . $e->getMessage(); // Display error message if connection fails } ?>
5. HTML 和 PHP 结构
HTML 结构 (index.html)
<meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Image Gallery Upload</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> <link rel="stylesheet" href="assets/css/styles.css"> <div class="container"> <h1 id="Image-Gallery">Image Gallery</h1> <div class="row"> <div class="col-md-6 offset-md-3"> <form id="uploadImage" enctype="multipart/form-data"> <div class="form-group"> <label for="image">Choose Image</label> <input type="file" name="image" id="image" class="form-control" required> </div> <button type="submit" class="btn btn-primary">Upload</button> </form> <div id="upload-status"></div> </div> </div> <hr> <div class="row" id="gallery"> <!-- Images will be loaded here --> </div> </div> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script src="assets/js/script.js"></script> <!-- Custom JS -->
6. JavaScript 和 AJAX
AJAX 处理 (assets/js/script.js)
$(document).ready(function(){ // load gallery on page load loadGallery(); // Form submission for image upload $('#uploadImage').on('submit', function(e){ e.preventDefault(); // Prevent default form submission var file_data = $('#image').prop('files')[0]; var form_data = new FormData(); form_data.append('file', file_data); //hide upload section $('#uploadImage').hide(); $.ajax({ url: 'src/upload.php', // PHP script to process upload type: 'POST', dataType: 'text', // what to expect back from the server cache: false, data: new FormData(this), // Form data for upload processData: false, contentType: false, success: function(response){ let jsonData = JSON.parse(response); if(jsonData.status == 1){ $('#image').val(''); // Clear the file input loadGallery(); // Fetch and display updated images $('#upload-status').html('<div class="alert alert-success">'+jsonData.message+'</div>'); } else { $('#upload-status').html('<div class="alert alert-success">'+jsonData.message+'</div>'); // Display error message } // hide message box autoHide('#upload-status'); //show upload section autoShow('#uploadImage'); } }); }); }); // Function to load the gallery from server function loadGallery() { $.ajax({ url: 'src/fetch-images.php', // PHP script to fetch images type: 'GET', success: function(response){ let jsonData = JSON.parse(response); $('#gallery').html(''); // Clear previous images if(jsonData.status == 1){ $.each(jsonData.data, function(index, image){ $('#gallery').append('<div class="col-md-3"><img class="img-responsive img-thumbnail lazy" src="/static/imghwm/default1.png" data-src="assets/uploads/'+image.file_name+'" alt="PHP 速成课程:简单图片库" ></div>'); // Display each image }); } else { $('#gallery').html('<p>No images found...</p>'); // No images found message } } }); } //Auto Hide Div function autoHide(idORClass) { setTimeout(function () { $(idORClass).fadeOut('fast'); }, 1000); } //Auto Show Element function autoShow(idORClass) { setTimeout(function () { $(idORClass).fadeIn('fast'); }, 1000); }
7. 后端 PHP 脚本
获取图像 (src/fetch-images.php)
<?php include '../include/db.php'; // Include database configuration $response = array('status' => 0, 'message' => 'No images found.'); // Fetching images from the database $query = $pdo->prepare("SELECT * FROM images ORDER BY uploaded_on DESC"); $query->execute(); $images = $query->fetchAll(PDO::FETCH_ASSOC); if(count($images) > 0){ $response['status'] = 1; $response['data'] = $images; // Returning images data } // Return response echo json_encode($response); ?> ?>
图片上传 (src/upload.php)
<?php include '../include/db.php'; // Include database configuration $response = array('status' => 0, 'message' => 'Form submission failed, please try again.'); if(isset($_FILES['image']['name'])){ // Directory where files will be uploaded $targetDir = UPLOAD_DIRECTORY; $fileName = date('Ymd').'-'.str_replace(' ', '-', basename($_FILES['image']['name'])); $targetFilePath = $targetDir . $fileName; $fileType = pathinfo($targetFilePath, PATHINFO_EXTENSION); // Allowed file types $allowTypes = array('jpg','png','jpeg','gif'); if(in_array($fileType, $allowTypes)){ // Upload file to server if(move_uploaded_file($_FILES['image']['tmp_name'], $targetFilePath)){ // Insert file name into database $insert = $pdo->prepare("INSERT INTO images (file_name, uploaded_on) VALUES (:file_name, NOW())"); $insert->bindParam(':file_name', $fileName); $insert->execute(); if($insert){ $response['status'] = 1; $response['message'] = 'Image uploaded successfully!'; }else{ $response['message'] = 'Image upload failed, please try again.'; } }else{ $response['message'] = 'Sorry, there was an error uploading your file.'; } }else{ $response['message'] = 'Sorry, only JPG, JPEG, PNG, & GIF files are allowed to upload.'; } } // Return response echo json_encode($response); ?>
6.CSS样式
CSS 样式 (assets/css/style.css)
body { background-color: #f8f9fa; } #gallery .col-md-4 { margin-bottom: 20px; } #gallery img { display: block; width: 200px; height: auto; margin: 10px; }
文档和评论
- config.php:包含数据库配置并使用PDO连接到MySQL。
- index.php:具有 HTML 结构的主页,包括用于样式设置的 Bootstrap 以及用于添加/编辑帖子的模式。
- assets/js/script.js: 处理加载和保存帖子的 AJAX 请求。使用 jQuery 进行 DOM 操作和 AJAX。
- src/fetch-images.php: 从数据库中获取帖子并将其作为 JSON 返回。
- src/upload.php: 根据 ID 的存在处理帖子创建和更新。
该解决方案包括设置项目、数据库配置、HTML 结构、CSS 样式、使用 AJAX 处理图像上传以及使用 PHP PDO 将图像数据存储在数据库中。每行代码都带有注释以解释其目的和功能,使其成为构建具有上传功能的图片库的综合教程。
连接链接
如果您发现本系列有帮助,请考虑在 GitHub 上给 存储库 一个星号或在您最喜欢的社交网络上分享该帖子?。您的支持对我来说意义重大!
如果您想要更多类似的有用内容,请随时关注我:
- 领英
- GitHub
源代码
以上是PHP 速成课程:简单图片库的详细内容。更多信息请关注PHP中文网其他相关文章!
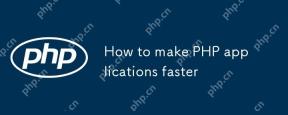
tomakephpapplicationsfaster,关注台词:1)useopcodeCachingLikeLikeLikeLikeLikePachetoStorePreciledScompiledScriptbyTecode.2)MinimimiedAtabaseSqueriSegrieSqueriSegeriSybysequeryCachingandeffeftExting.3)Leveragephp7 leveragephp7 leveragephp7 leveragephpphp7功能forbettercodeefficy.4)
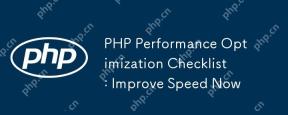
到ImprovephPapplicationspeed,关注台词:1)启用opcodeCachingwithapCutoredUcescriptexecutiontime.2)实现databasequerycachingusingpdotominiminimizedatabasehits.3)usehttp/2tomultiplexrequlexrequestsandredececonnection.4 limitsclection.4.4
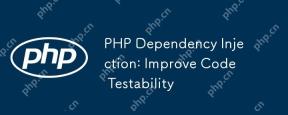
依赖注入(DI)通过显式传递依赖关系,显着提升了PHP代码的可测试性。 1)DI解耦类与具体实现,使测试和维护更灵活。 2)三种类型中,构造函数注入明确表达依赖,保持状态一致。 3)使用DI容器管理复杂依赖,提升代码质量和开发效率。
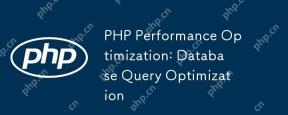
databasequeryOptimizationinphpinvolVolVOLVESEVERSEVERSTRATEMIESOENHANCEPERANCE.1)SELECTONLYNLYNESSERSAYCOLUMNSTORMONTOUMTOUNSOUDSATATATATATATATATATATRANSFER.3)
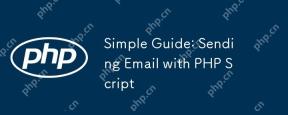
phpisusedforsenderemailsduetoitsbuilt-inmail()函数andsupportiveLibrariesLikePhpMailerandSwiftMailer.1)usethemail()functionforbasicemails,butithasimails.2)butithasimimitations.2)
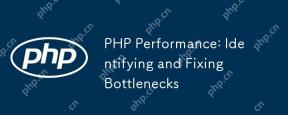
PHP性能瓶颈可以通过以下步骤解决:1)使用Xdebug或Blackfire进行性能分析,找出问题所在;2)优化数据库查询并使用缓存,如APCu;3)使用array_filter等高效函数优化数组操作;4)配置OPcache进行字节码缓存;5)优化前端,如减少HTTP请求和优化图片;6)持续监控和优化性能。通过这些方法,可以显着提升PHP应用的性能。
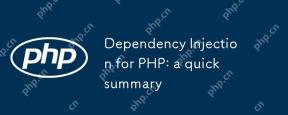
依赖性注射(DI)InphpisadesignPatternthatManages和ReducesClassDeptions,增强量产生性,可验证性和Maintainability.itallowspasspassingDepentenciesLikEdenceSeconnectionSeconnectionStoclasseconnectionStoclasseSasasasasareTers,interitationApertatingAeseritatingEaseTestingEasingEaseTeStingEasingAndScalability。
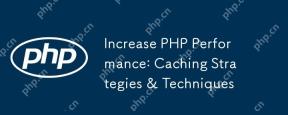
cachingimprovesphpermenceByStorcyResultSofComputationsorqucrouctationsorquctationsorquickretrieval,reducingServerLoadAndenHancingResponsetimes.feftectivestrategiesinclude:1)opcodecaching,whereStoresCompiledSinmememorytssinmemorytoskipcompliation; 2)datacaching datacachingsingMemccachingmcachingmcachings


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SublimeText3汉化版
中文版,非常好用

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。