当启动一个将使用 JavaScript 的新 Web 项目时,我们做的第一件事通常是设置构建和开发人员工具。比如现在很流行的Vite。您可能不知道并非所有 JavaScript(Web)项目都需要复杂的构建工具。事实上,正如我将在本文中展示的那样,现在比以往任何时候都更容易摆脱困境。
使用index.html 文件创建一个新项目。
<p>Hello world</p>
如果您使用的是 VS Code,请安装实时预览扩展。运行它。这是一个具有实时重新加载功能的简单文件服务器。您可以使用任何文件服务器,Python 内置了一个:
python3 -m http.server
我喜欢实时预览,因为它会在更改文件后自动刷新页面。
您现在应该能够从浏览器访问您的index.html 文件并看到“Hello world”。
接下来,创建一个index.js文件:
console.log("Hello world"); export {};
将其包含在您的index.html中:
<script type="module" src="./index.js"></script>
在浏览器中打开开发者控制台。如果您看到“Hello world”,则说明它正在正确加载。
浏览器现在支持 ECMAScript 模块。您可以导入其他文件以消除其副作用:
import "./some-other-script.js";
或用于出口
import { add, multiply } "./my-math-lib.js";
很酷吧?请参阅上面的 MDN 指南了解更多信息。
套餐
您可能不想重新发明轮子,因此您的项目可能会使用一些第三方包。这并不意味着您现在需要开始使用包管理器。
假设我们想使用超级结构进行数据验证。我们不仅可以从我们自己的(本地)文件服务器加载模块,还可以从任何 URL 加载模块。 esm.sh 方便地为 npm 上可用的几乎所有包提供模块。
当您访问 https://esm.sh/superstruct 时,您可以看到您被重定向到最新版本。您可以在代码中包含此包,如下所示:
import { assert } from "https://esm.sh/superstruct";
如果您想安全起见,您可以固定版本。
类型
我不了解你,但 TypeScript 宠坏了我(并且让我变得懒惰)。在没有类型检查器帮助的情况下编写纯 JavaScript 感觉就像走钢丝一样。幸运的是,我们也不必放弃类型检查。
是时候淘汰 npm 了(尽管我们不会发布它提供的任何代码)。
npm init --yes npm install typescript
您可以在 JavaScript 代码上使用 TypeScript 编译器!它有一流的支持。创建 jsconfig.json:
{ "compilerOptions": { "strict": true, "checkJs": true, "allowJs": true, "noImplicitAny": true, "lib": ["ES2022", "DOM"], "module": "ES2022", "target": "ES2022" }, "include": ["**/*.js"], "exclude": ["node_modules"] }
现在运行
npm run tsc --watch -p jsconfig.json
并在代码中犯一个类型错误。 TypeScript 编译器应该抱怨:
/** @type {number} **/ const num = "hello";
顺便说一下,你上面看到的评论是 JSDoc。您可以通过这种方式用类型注释您的 JavaScript。虽然它比使用 TypeScript 更冗长一些,但你很快就会习惯它。它也非常强大,只要您不编写疯狂的类型(对于大多数项目来说您不应该这样做),您应该没问题。
如果您确实需要复杂的类型(助手),您可以随时在 .d.ts 文件中添加一些 TypeScript。
JSDoc 是否只是那些陷入大型 JavaScript 项目的人们能够逐步迁移到 TypeScript 的垫脚石?我不这么认为! TypeScript 团队还继续向 JSDoc + TypeScript 添加出色的功能,例如即将发布的 TypeScript 版本。自动完成功能在 VS Code 中也非常有效。
导入地图
我们学习了如何在不使用构建工具的情况下将外部包添加到我们的项目中。但是,如果您将代码拆分为许多模块,则一遍又一遍地写出完整的 URL 可能会有点冗长。
我们可以将导入映射添加到我们的index.html的head部分:
<script type="importmap"> { "imports": { "superstruct": "https://esm.sh/superstruct@1.0.4" } } </script>
现在我们可以简单地使用
导入这个包
import {} from "superstruct"
就像一个“正常”项目。另一个好处是,如果您在本地安装该软件包,类型的完成和识别将按预期工作。
npm install --save-dev superstruct
请注意,node_modules 目录中的版本将不会被使用。您可以将其删除,您的项目将继续运行。
我喜欢使用的一个技巧是添加:
"cdn/": "https://esm.sh/",
到我的导入地图。然后,通过 esm.sh 提供的任何项目都可以通过简单地导入来使用。例如:
import Peer from "cdn/peerjs";
如果您也想从node_modules中提取类型以进行此类导入的开发,则需要将以下内容添加到jsconfig.json的compilerOptions中:
"paths": { "cdn/*": ["./node_modules/*", "./node_modules/@types/*"] },
部署
要部署您的项目,请将所有文件复制到静态文件主机,然后就完成了!如果您曾经参与过旧版 JavaScript 项目,您就会知道更新不到 1-2 年的构建工具的痛苦。通过此项目设置,您将不会遭受同样的命运。
Testing
If your JavaScript does not depend on browser APIs, you could just use the test runner that comes bundled with Node.js. But why not write your own test runner that runs right in the browser?
/** @type {[string, () => Promise<void> | void][]} */ const tests = []; /** * * @param {string} description * @param {() => Promise<void> | void} testFunc */ export async function test(description, testFunc) { tests.push([description, testFunc]); } export async function runAllTests() { const main = document.querySelector("main"); if (!(main instanceof HTMLElement)) throw new Error(); main.innerHTML = ""; for (const [description, testFunc] of tests) { const newSpan = document.createElement("p"); try { await testFunc(); newSpan.textContent = `✅ ${description}`; } catch (err) { const errorMessage = err instanceof Error && err.message ? ` - ${err.message}` : ""; newSpan.textContent = `❌ ${description}${errorMessage}`; } main.appendChild(newSpan); } } /** * @param {any} val */ export function assert(val, message = "") { if (!val) throw new Error(message); } </void></void>
Now create a file example.test.js.
import { test, assert } from "@/test.js"; test("1+1", () => { assert(1 + 1 === 2); });
And a file where you import all your tests:
import "./example.test.js"; console.log("This should only show up when running tests");
Run this on page load:
await import("@/test/index.js"); // file that imports all tests (await import("@/test.js")).runAllTests();
And you got a perfect TDD setup. To run only a section of the tests you can comment out a few .test.js import, but test execution speed should only start to become a problem when you have accumulated a lot of tests.
Benefits
Why would you do this? Well, using fewer layers of abstraction makes your project easier to debug. There is also the credo to "use the platform". The skills you learn will transfer better to other projects. Another advantage is, when you return to a project built like this in 10 years, it will still just work and you don't need to do archeology to try to revive a build tool that has been defunct for 8 years. An experience many web developers that worked on legacy projects will be familiar with.
See plainvanillaweb.com for some more ideas.
以上是无需(构建)工具的 Web 开发的详细内容。更多信息请关注PHP中文网其他相关文章!
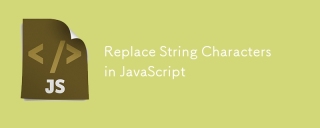
JavaScript字符串替换方法详解及常见问题解答 本文将探讨两种在JavaScript中替换字符串字符的方法:在JavaScript代码内部替换和在网页HTML内部替换。 在JavaScript代码内部替换字符串 最直接的方法是使用replace()方法: str = str.replace("find","replace"); 该方法仅替换第一个匹配项。要替换所有匹配项,需使用正则表达式并添加全局标志g: str = str.replace(/fi
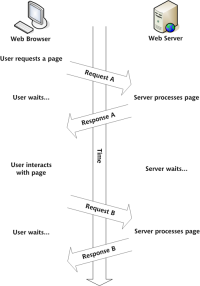
因此,在这里,您准备好了解所有称为Ajax的东西。但是,到底是什么? AJAX一词是指用于创建动态,交互式Web内容的一系列宽松的技术。 Ajax一词,最初由Jesse J创造

本文讨论了在浏览器中优化JavaScript性能的策略,重点是减少执行时间并最大程度地减少对页面负载速度的影响。
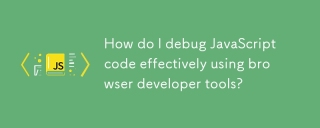
本文讨论了使用浏览器开发人员工具的有效JavaScript调试,专注于设置断点,使用控制台和分析性能。
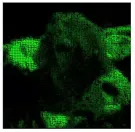
将矩阵电影特效带入你的网页!这是一个基于著名电影《黑客帝国》的酷炫jQuery插件。该插件模拟了电影中经典的绿色字符特效,只需选择一张图片,插件就会将其转换为充满数字字符的矩阵风格画面。快来试试吧,非常有趣! 工作原理 插件将图片加载到画布上,读取像素和颜色值: data = ctx.getImageData(x, y, settings.grainSize, settings.grainSize).data 插件巧妙地读取图片的矩形区域,并利用jQuery计算每个区域的平均颜色。然后,使用
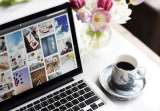
本文将引导您使用jQuery库创建一个简单的图片轮播。我们将使用bxSlider库,它基于jQuery构建,并提供许多配置选项来设置轮播。 如今,图片轮播已成为网站必备功能——一图胜千言! 决定使用图片轮播后,下一个问题是如何创建它。首先,您需要收集高质量、高分辨率的图片。 接下来,您需要使用HTML和一些JavaScript代码来创建图片轮播。网络上有很多库可以帮助您以不同的方式创建轮播。我们将使用开源的bxSlider库。 bxSlider库支持响应式设计,因此使用此库构建的轮播可以适应任何
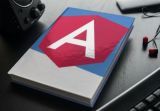
数据集对于构建API模型和各种业务流程至关重要。这就是为什么导入和导出CSV是经常需要的功能。在本教程中,您将学习如何在Angular中下载和导入CSV文件


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

SublimeText3汉化版
中文版,非常好用

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。

Atom编辑器mac版下载
最流行的的开源编辑器

记事本++7.3.1
好用且免费的代码编辑器

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),