常见问题:测试颗粒度过大:将测试分解为较小单元。测试速度慢:使用并行测试和数据驱动的测试。测试不稳定:使用 mocks 和测试夹具隔离测试。测试覆盖率不足:使用代码覆盖工具和 mutate 测试。
GoLang 框架自动化测试常见问题及其解决方案
简介
自动化测试对于确保软件质量至关重要。在 GoLang 中,有多种可用于自动化测试的框架。然而,在使用这些框架时经常会遇到一些常见问题。本文将探讨这些常见问题并提供解决方案。
问题 1:测试颗粒度过大
问题:测试案例过大,导致难以维护和调试。
解决方案:
-
将测试案例分解为较小的单元,每个单元测试应用程序的特定功能。
func TestAddNumbers(t *testing.T) { result := AddNumbers(1, 2) if result != 3 { t.Errorf("Expected 3, got %d", result) } }
问题 2:测试速度慢
问题:测试套件执行缓慢,阻碍了开发进度。
解决方案:
-
使用并行测试来同时运行多个测试案例。
import "testing" func TestAddNumbers(t *testing.T) { t.Parallel() result := AddNumbers(1, 2) if result != 3 { t.Errorf("Expected 3, got %d", result) } }
-
使用数据驱动的测试来减少代码重复。
type AddNumbersTestData struct { a int b int result int } func TestAddNumbers(t *testing.T) { tests := []AddNumbersTestData{ {1, 2, 3}, {3, 4, 7}, } for _, test := range tests { result := AddNumbers(test.a, test.b) if result != test.result { t.Errorf("For a=%d, b=%d, expected %d, got %d", test.a, test.b, test.result, result) } } }
问题 3:测试不稳定
问题:测试结果不一致,导致调试困难。
解决方案:
-
使用 mocks 和 stubs 隔离测试,避免外部依赖项的影响。
type NumberGenerator interface { Generate() int } type MockNumberGenerator struct { numbers []int } func (m *MockNumberGenerator) Generate() int { return m.numbers[0] } func TestAddNumbersWithMock(t *testing.T) { m := &MockNumberGenerator{[]int{1, 2}} result := AddNumbers(m, m) if result != 3 { t.Errorf("Expected 3, got %d", result) } }
-
使用测试夹具设置和拆除测试环境。
import "testing" type TestFixture struct { // Setup and teardown code } func TestAddNumbersWithFixture(t *testing.T) { fixture := &TestFixture{} t.Run("case 1", fixture.testFunc1) t.Run("case 2", fixture.testFunc2) } func (f *TestFixture) testFunc1(t *testing.T) { // ... } func (f *TestFixture) testFunc2(t *testing.T) { // ... }
问题 4:测试覆盖率不足
问题:测试没有覆盖应用程序的足够代码路径,导致潜在错误被忽视。
解决方案:
-
使用代码覆盖工具来识别未覆盖的代码。
import ( "testing" "github.com/stretchr/testify/assert" ) func TestAddNumbers(t *testing.T) { assert.Equal(t, 3, AddNumbers(1, 2)) } func TestCoverage(t *testing.T) { // Coverage report generation code }
-
使用mutate测试来生成程序的变异体,并执行测试来检测意外行为。
import ( "testing" "github.com/dvyukov/go-fuzz-corpus/fuzz" ) func FuzzAddNumbers(f *fuzz.Fuzz) { a := f.Intn(100) b := f.Intn(100) f.Check(AddNumbers(a, b)) }
以上是golang框架自动化测试常见问题及解决方案的详细内容。更多信息请关注PHP中文网其他相关文章!
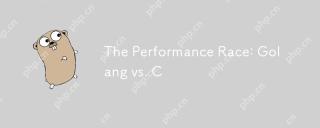
Golang和C 在性能竞赛中的表现各有优势:1)Golang适合高并发和快速开发,2)C 提供更高性能和细粒度控制。选择应基于项目需求和团队技术栈。
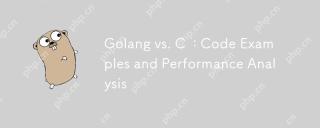
Golang适合快速开发和并发编程,而C 更适合需要极致性能和底层控制的项目。1)Golang的并发模型通过goroutine和channel简化并发编程。2)C 的模板编程提供泛型代码和性能优化。3)Golang的垃圾回收方便但可能影响性能,C 的内存管理复杂但控制精细。
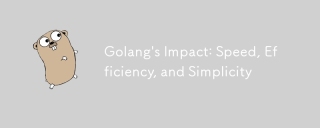
GoimpactsdevelopmentPositationalityThroughSpeed,效率和模拟性。1)速度:gocompilesquicklyandrunseff,ifealforlargeprojects.2)效率:效率:ITScomprehenSevestAndArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增强开发的简单性:3)SimpleflovelmentIcties:3)简单性。
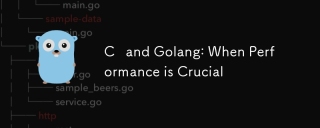
C 更适合需要直接控制硬件资源和高性能优化的场景,而Golang更适合需要快速开发和高并发处理的场景。1.C 的优势在于其接近硬件的特性和高度的优化能力,适合游戏开发等高性能需求。2.Golang的优势在于其简洁的语法和天然的并发支持,适合高并发服务开发。
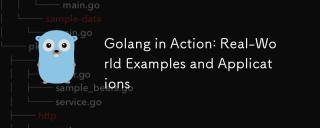
Golang在实际应用中表现出色,以简洁、高效和并发性着称。 1)通过Goroutines和Channels实现并发编程,2)利用接口和多态编写灵活代码,3)使用net/http包简化网络编程,4)构建高效并发爬虫,5)通过工具和最佳实践进行调试和优化。
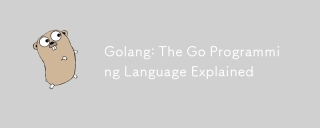
Go语言的核心特性包括垃圾回收、静态链接和并发支持。1.Go语言的并发模型通过goroutine和channel实现高效并发编程。2.接口和多态性通过实现接口方法,使得不同类型可以统一处理。3.基本用法展示了函数定义和调用的高效性。4.高级用法中,切片提供了动态调整大小的强大功能。5.常见错误如竞态条件可以通过gotest-race检测并解决。6.性能优化通过sync.Pool重用对象,减少垃圾回收压力。
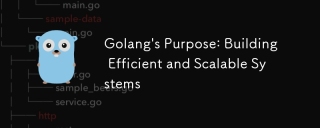
Go语言在构建高效且可扩展的系统中表现出色,其优势包括:1.高性能:编译成机器码,运行速度快;2.并发编程:通过goroutines和channels简化多任务处理;3.简洁性:语法简洁,降低学习和维护成本;4.跨平台:支持跨平台编译,方便部署。
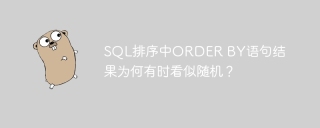
关于SQL查询结果排序的疑惑学习SQL的过程中,常常会遇到一些令人困惑的问题。最近,笔者在阅读《MICK-SQL基础�...


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具
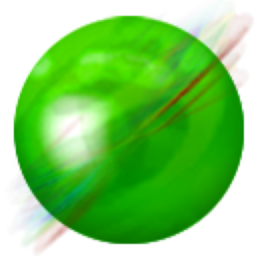
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具
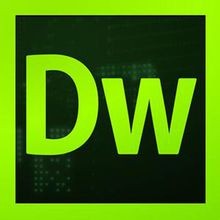
Dreamweaver CS6
视觉化网页开发工具
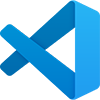
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器
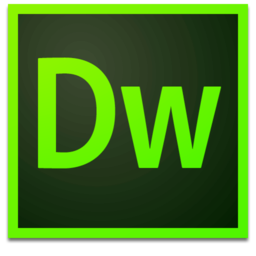
Dreamweaver Mac版
视觉化网页开发工具