通过使用 Go 语言的内置测试框架,开发者可以轻松地为他们的代码编写和运行测试。测试文件以 _test.go 结尾,并包含 Test 开头的测试函数,其中 *testing.T 参数表示测试实例。错误信息使用 t.Error() 记录。可以通过运行 go test 命令来运行测试。子测试允许将测试函数分解成更小的部分,并通过 t.Run() 创建。实战案例包括针对 utils 包中 IsStringPalindrome() 函数编写的测试文件,该文件使用一系列输入字符串和预期输出来测试该函数的正确性。
如何在 Go 语言中测试包?
Go 语言提供了强大的内置测试框架,让开发者轻松地为其代码编写和运行测试。下面将介绍如何使用 Go 测试包对你的程序进行测试。
编写测试
在 Go 中,测试文件以 _test.go 结尾,并放在与要测试的包所在的目录中。测试文件包含一个或多个测试函数,它们以 Test
开头,后面跟要测试的功能。
以下是一个示例测试函数:
import "testing" func TestAdd(t *testing.T) { if Add(1, 2) != 3 { t.Error("Add(1, 2) returned an incorrect result") } }
*testing.T
参数表示测试实例。错误信息使用 t.Error()
记录。
运行测试
可以通过运行以下命令来运行测试:
go test
如果测试成功,将显示诸如 "PASS" 之类的消息。如果出现错误,将显示错误信息。
使用子测试
子测试允许将一个测试函数分解成更小的部分。这有助于组织测试代码并提高可读性。
以下是如何编写子测试:
func TestAdd(t *testing.T) { t.Run("PositiveNumbers", func(t *testing.T) { if Add(1, 2) != 3 { t.Error("Add(1, 2) returned an incorrect result") } }) t.Run("NegativeNumbers", func(t *testing.T) { if Add(-1, -2) != -3 { t.Error("Add(-1, -2) returned an incorrect result") } }) }
实战案例
假设我们有一个名为 utils
的包,里面包含一个 IsStringPalindrome()
函数,用于检查一个字符串是否是回文字符串。
下面是如何编写一个测试文件来测试这个函数:
package utils_test import ( "testing" "utils" ) func TestIsStringPalindrome(t *testing.T) { tests := []struct { input string expected bool }{ {"", true}, {"a", true}, {"bb", true}, {"racecar", true}, {"level", true}, {"hello", false}, {"world", false}, } for _, test := range tests { t.Run(test.input, func(t *testing.T) { if got := utils.IsStringPalindrome(test.input); got != test.expected { t.Errorf("IsStringPalindrome(%s) = %t; want %t", test.input, got, test.expected) } }) } }
在这个测试文件中:
-
tests
数组定义了一系列输入字符串和预期的输出。 -
for
循环遍历tests
数组,并使用t.Run()
创建子测试。 - 每个子测试调用
utils.IsStringPalindrome()
函数并将其结果与预期结果进行比较。如果结果不一致,它使用t.Errorf()
记录错误。
以上是如何在 Go 语言中测试包?的详细内容。更多信息请关注PHP中文网其他相关文章!
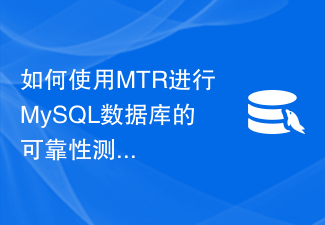
如何使用MTR进行MySQL数据库的可靠性测试?概述:MTR(MySQL测试运行器)是MySQL官方提供的一个测试工具,可以帮助开发人员进行MySQL数据库的功能和性能测试。在开发过程中,为了确保数据库的可靠性和稳定性,我们经常需要进行各种测试,而MTR提供了一种简单方便且可靠的方法来进行这些测试。步骤:安装MySQL测试运行器:首先,需要从MySQL官方网
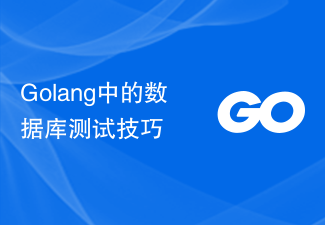
Golang中的数据库测试技巧引言:在开发应用程序时,数据库测试是一个非常重要的环节。合适的测试方法可以帮助我们发现潜在的问题并确保数据库操作的正确性。本文将介绍Golang中的一些常用数据库测试技巧,并提供相应的代码示例。一、使用内存数据库进行测试在编写数据库相关的测试时,我们通常会面临一个问题:如何在不依赖外部数据库的情况下进行测试?这里我们可以使用内存
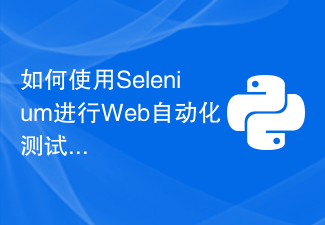
如何使用Selenium进行Web自动化测试概述:Web自动化测试是现代软件开发过程中至关重要的一环。Selenium是一个强大的自动化测试工具,可以模拟用户在Web浏览器中的操作,实现自动化的测试流程。本文将介绍如何使用Selenium进行Web自动化测试,并附带代码示例,帮助读者快速上手。环境准备在开始之前,需要安装Selenium库和Web浏览器驱动程
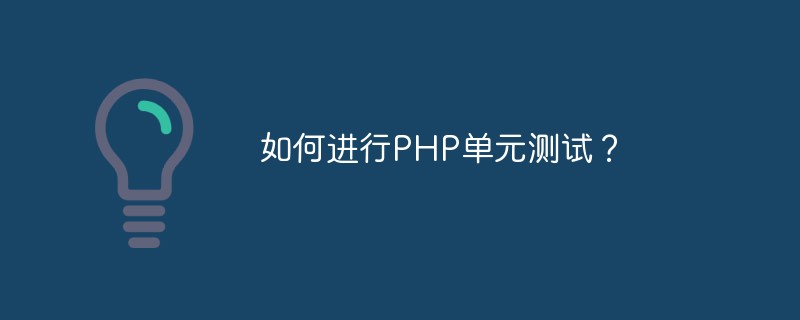
在Web开发中,PHP是一种流行的语言,因此对于任何人来说,对PHP进行单元测试是一个必须掌握的技能。本文将介绍什么是PHP单元测试以及如何进行PHP单元测试。一、什么是PHP单元测试?PHP单元测试是指测试一个PHP应用程序的最小组成部分,也称为代码单元。这些代码单元可以是方法、类或一组类。PHP单元测试旨在确认每个代码单元都能按预期工作,并且能否正确地与
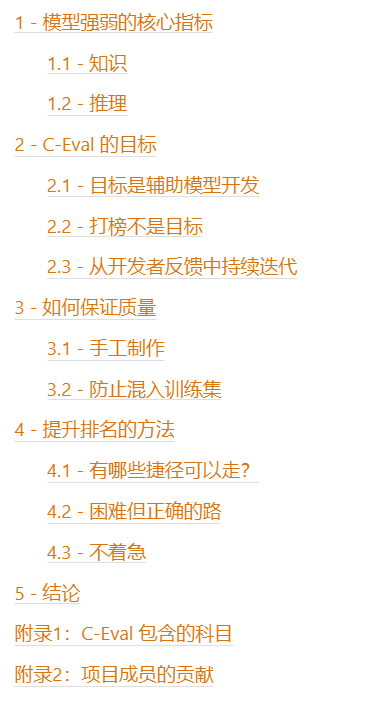
ChatGPT的出现,使中文社区意识到与国际领先水平的差距。近期,中文大模型研发如火如荼,但中文评价基准却很少。在OpenAIGPT系列/GooglePaLM系列/DeepMindChinchilla系列/AnthropicClaude系列的研发过程中,MMLU/MATH/BBH这三个数据集发挥了至关重要的作用,因为它们比较全面地覆盖了模型各个维度的能力。最值得注意的是MMLU这个数据集,它考虑了57个学科,从人文到社科到理工多个大类的综合知识能力。DeepMind的Gopher和Chinchi
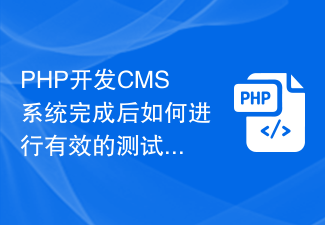
在日益发展的互联网时代中,CMS系统已经成为了网络建设中的一项重要工具。其中PHP语言开发的CMS系统因其简单易用,自由度高,成为了经典的CMS系统之一。然而,PHP开发CMS系统过程中的测试工作也是至关重要的。只有经过完善、系统的测试工作,我们才可以保证开发出的CMS系统在使用中更加稳定、可靠。那么,如何进行有效的PHP开发CMS系统测试呢?一、测试流程的
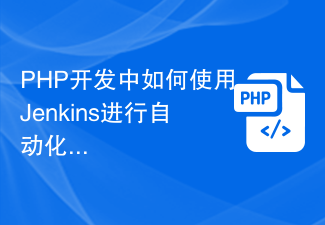
随着Web应用程序规模的不断扩大,PHP语言的应用也越来越广泛。在软件开发过程中,自动化测试可以大大提高开发效率和软件质量。而Jenkins是一个可扩展的开源自动化服务器,它能够自动执行软件构建、测试、部署等操作,今天我们来看一下在PHP开发中如何使用Jenkins进行自动化测试。一、Jenkins的安装和配置首先,我们需要在服务器上安
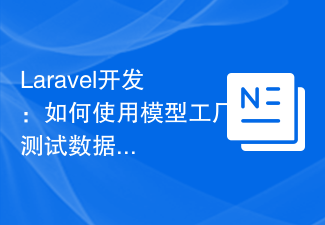
Laravel是一个流行的PHPWeb开发框架,以其简洁易用的API设计,丰富的函数库和强大的生态系统而著名。在使用Laravel进行项目开发时,测试是非常重要的一个环节。Laravel提供了多种测试工具和技术,其中模型工厂是其中的重要组成部分。本文将介绍如何在Laravel项目中使用模型工厂来测试数据库。一、模型工厂的作用在Laravel中,模型工厂是用


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中

SublimeText3 英文版
推荐:为Win版本,支持代码提示!

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。
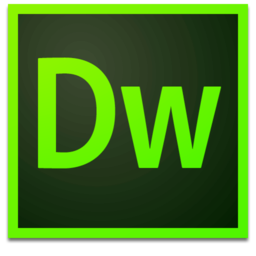
Dreamweaver Mac版
视觉化网页开发工具