多執行緒 C 例外處理指南提出了四種關鍵方法:使用互斥量或原子操作確保異常處理的執行緒安全性。利用執行緒局部儲存 (TLS) 為每個執行緒儲存異常資訊。透過 std::async 和 std::future 實現非同步任務和異常傳播。透過 TLS 和主執行緒收集異常訊息,實現多執行緒檔案下載中的異常處理。
C 並發程式設計:多執行緒異常處理實戰指南
在多執行緒環境中,異常處理特別關鍵,它能夠確保應用程式在發生意外情況時仍能正常運作。本文將介紹如何處理 C 中多執行緒環境下的異常,並透過一個實戰案例加以示範。
異常同步與執行緒安全
在多執行緒環境中,例外的拋出和處理需要同步,以確保不會出現資料競爭或死鎖。可以使用互斥量或原子操作來確保異常處理的執行緒安全。
// 使用互斥量实现线程安全异常处理 std::mutex m; void handle_error() { std::unique_lock<std::mutex> lock(m); // 处理异常 }
線程局部儲存(Thread-Local Storage)
線程局部儲存(TLS) 可以為每個執行緒提供獨自の儲存區域,用於儲存特定於該執行緒的數據,包括異常訊息。
// 使用 TLS 存储每个线程的异常信息 __thread std::exception_ptr exception_ptr; void set_exception(const std::exception& e) { exception_ptr = std::make_exception_ptr(e); }
異常傳播與處理
在多執行緒環境中,異常可以從一個執行緒傳播到另一個執行緒。可以使用 std::async
和 std::future
來非同步執行任務,並處理執行緒中拋出的例外。
// 在异步任务中处理异常 auto f = std::async(std::launch::async, []() { try { // 执行任务 } catch (const std::exception& e) { std::cout << "Exception caught in async task: " << e.what() << std::endl; } }); // 在主线程中检查异常 if (f.get()) { std::cout << "Async task completed successfully" << std::endl; } else { std::cout << "Async task failed with exception" << std::endl; }
實戰案例:多執行緒檔案下載
考慮一個多執行緒檔案下載應用程序,其中每個執行緒負責下載檔案的一部分。為了處理異常,我們可以使用 TLS 儲存下載失敗的異常訊息,並在主線程中收集這些資訊。
#include <thread> #include <vector> #include <iostream> #include <fstream> using namespace std; // TLS 存储下载失败的异常信息 __thread exception_ptr exception_ptr; // 下载文件的线程函数 void download_file(const string& url, const string& path) { try { ofstream file(path, ios::binary); // 略:从 URL 下载数据并写入文件 } catch (const exception& e) { exception_ptr = make_exception_ptr(e); } } // 主线程函数 int main() { // 创建下载线程 vector<thread> threads; for (const auto& url : urls) { string path = "file_" + to_string(i) + ".txt"; threads.emplace_back(download_file, url, path); } // 加入线程并收集异常信息 for (auto& thread : threads) { thread.join(); if (exception_ptr) { try { rethrow_exception(exception_ptr); } catch (const exception& e) { cerr << "File download failed: " << e.what() << endl; } } } return 0; }
透過這些方法,我們可以有效地處理 C 多執行緒環境下的異常,確保應用程式的健全性和穩定性。
以上是C++並發程式設計:如何處理多執行緒環境下的例外處理?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
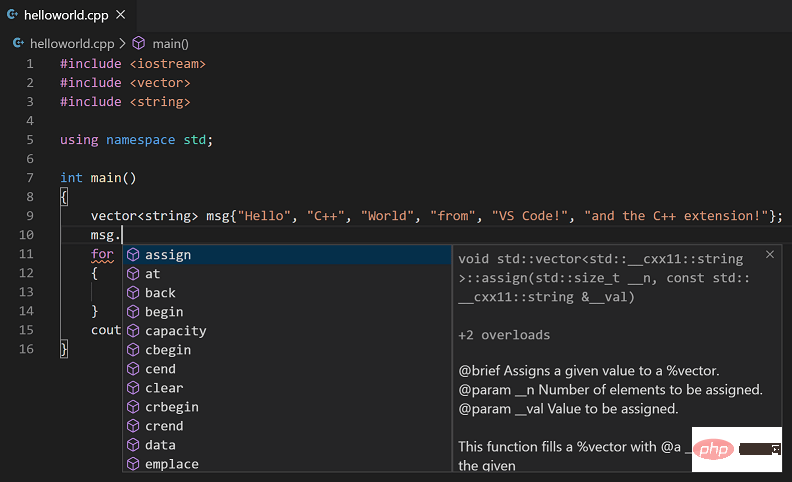
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
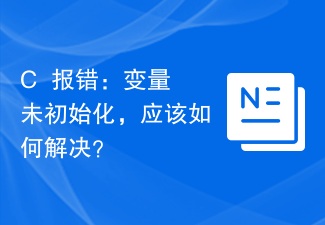
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
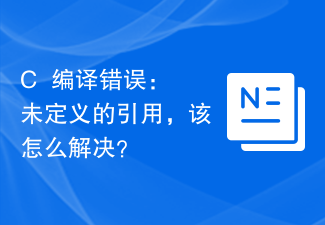
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
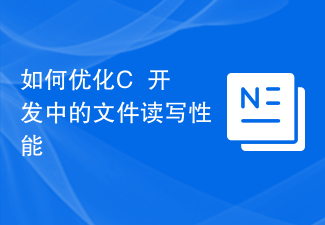
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
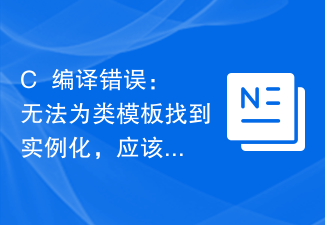
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
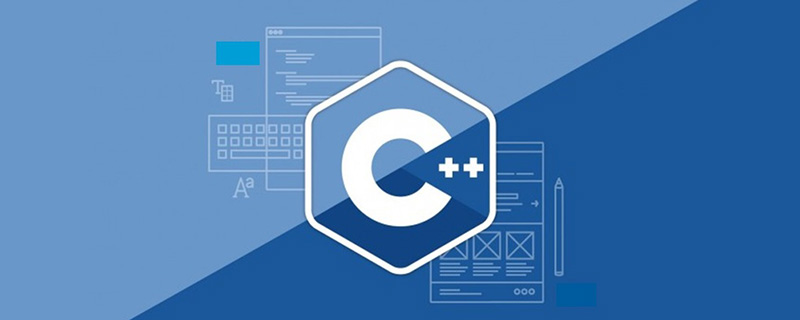
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
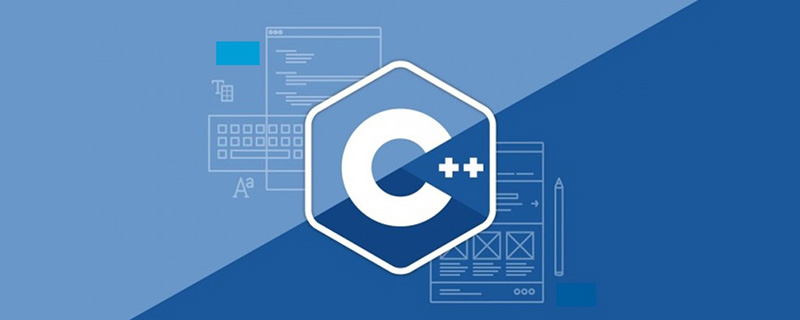
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
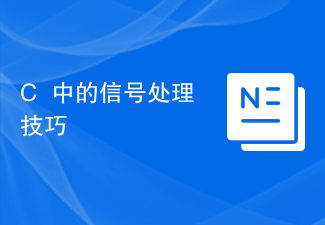
C++是一种流行的编程语言,它强大而灵活,适用于各种应用程序开发。在使用C++开发应用程序时,经常需要处理各种信号。本文将介绍C++中的信号处理技巧,以帮助开发人员更好地掌握这一方面。一、信号处理的基本概念信号是一种软件中断,用于通知应用程序内部或外部事件。当特定事件发生时,操作系统会向应用程序发送信号,应用程序可以选择忽略或响应此信号。在C++中,信号可以


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。
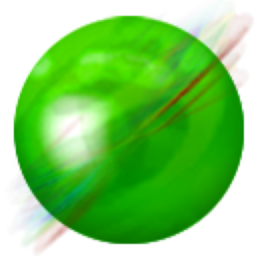
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

禪工作室 13.0.1
強大的PHP整合開發環境
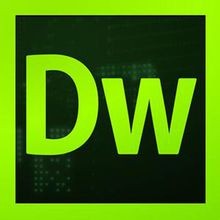
Dreamweaver CS6
視覺化網頁開發工具