異常處理最佳化可平衡錯誤處理與效率:僅在嚴重錯誤時使用異常。使用 noexcept 規範聲明不引發異常的函數。避免巢狀異常,將其放入 try-catch 區塊中。使用 exception_ptr 捕獲不能立即處理的異常。
C 函數異常效能最佳化:平衡錯誤處理與效率
簡介
#在C 中使用異常處理對於處理錯誤條件至關重要。然而,濫用異常可能會對效能產生重大影響。本文將探討優化異常處理以平衡錯誤處理和效率的技巧。
最佳化原則
- 僅在嚴重錯誤時使用例外:為可復原的錯誤使用錯誤代碼或日誌記錄。
- 使用 noexcept 規範:對於不引發異常的函數,使用 noexcept 規範,以告訴編譯器可以最佳化異常處理程式碼。
- 避免巢狀例外:巢狀例外會增加開銷,使得偵錯變得困難。
- 使用 try-catch 區塊:將例外處理程式碼放在 try-catch 區塊中,以便隔離處理程式碼。
- 使用 exception_ptr:在無法立即處理例外狀況時,使用 exception_ptr 來擷取並以後處理例外狀況。
實戰案例
未經最佳化的程式碼:
void process_file(const std::string& filename) { try { std::ifstream file(filename); // 代码过程... } catch (std::ifstream::failure& e) { std::cerr << "Error opening file: " << e.what() << std::endl; } }
使用nofail:
void process_file_nofail(const std::string& filename) { std::ifstream file(filename, std::ifstream::nofail); if (!file) { std::cerr << "Error opening file: " << file.rdstate() << std::endl; return; } // 代码过程... }
使用try-catch 區塊:
void process_file_try_catch(const std::string& filename) { std::ifstream file(filename); try { if (!file) { throw std::runtime_error("Error opening file"); } // 代码过程... } catch (const std::runtime_error& e) { std::cerr << "Error: " << e.what() << std::endl; } }
使用exception_ptr:
std::exception_ptr process_file_exception_ptr(const std::string& filename) { std::ifstream file(filename); try { if (!file) { throw std::runtime_error("Error opening file"); } // 代码过程... } catch (const std::runtime_error& e) { return std::make_exception_ptr(e); } return nullptr; }
以上是C++ 函數異常效能最佳化:平衡錯誤處理與效率的詳細內容。更多資訊請關注PHP中文網其他相關文章!
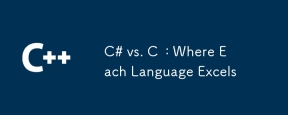
C#適合需要高開發效率和跨平台支持的項目,而C 適用於需要高性能和底層控制的應用。 1)C#簡化開發,提供垃圾回收和豐富類庫,適合企業級應用。 2)C 允許直接內存操作,適用於遊戲開發和高性能計算。
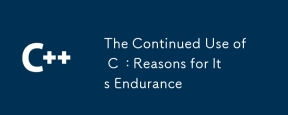
C 持續使用的理由包括其高性能、廣泛應用和不斷演進的特性。 1)高效性能:通過直接操作內存和硬件,C 在系統編程和高性能計算中表現出色。 2)廣泛應用:在遊戲開發、嵌入式系統等領域大放異彩。 3)不斷演進:自1983年發布以來,C 持續增加新特性,保持其競爭力。
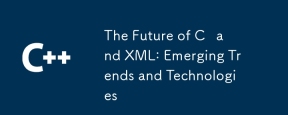
C 和XML的未來發展趨勢分別為:1)C 將通過C 20和C 23標準引入模塊、概念和協程等新特性,提升編程效率和安全性;2)XML將繼續在數據交換和配置文件中佔據重要地位,但會面臨JSON和YAML的挑戰,並朝著更簡潔和易解析的方向發展,如XMLSchema1.1和XPath3.1的改進。
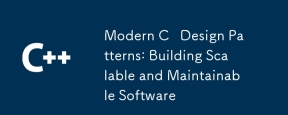
現代C 設計模式利用C 11及以後的新特性實現,幫助構建更靈活、高效的軟件。 1)使用lambda表達式和std::function簡化觀察者模式。 2)通過移動語義和完美轉發優化性能。 3)智能指針確保類型安全和資源管理。
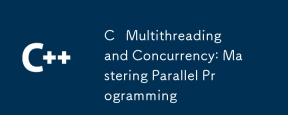
C 多線程和並發編程的核心概念包括線程的創建與管理、同步與互斥、條件變量、線程池、異步編程、常見錯誤與調試技巧以及性能優化與最佳實踐。 1)創建線程使用std::thread類,示例展示瞭如何創建並等待線程完成。 2)同步與互斥使用std::mutex和std::lock_guard保護共享資源,避免數據競爭。 3)條件變量通過std::condition_variable實現線程間的通信和同步。 4)線程池示例展示瞭如何使用ThreadPool類並行處理任務,提高效率。 5)異步編程使用std::as
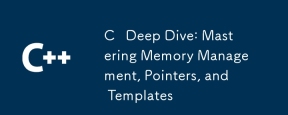
C 的內存管理、指針和模板是核心特性。 1.內存管理通過new和delete手動分配和釋放內存,需注意堆和棧的區別。 2.指針允許直接操作內存地址,使用需謹慎,智能指針可簡化管理。 3.模板實現泛型編程,提高代碼重用性和靈活性,需理解類型推導和特化。
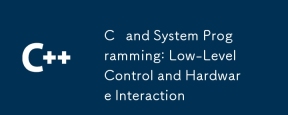
C 適合系統編程和硬件交互,因為它提供了接近硬件的控制能力和麵向對象編程的強大特性。 1)C 通過指針、內存管理和位操作等低級特性,實現高效的系統級操作。 2)硬件交互通過設備驅動程序實現,C 可以編寫這些驅動程序,處理與硬件設備的通信。
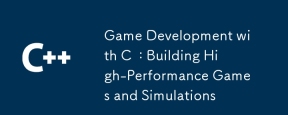
C 適合構建高性能遊戲和仿真係統,因為它提供接近硬件的控制和高效性能。 1)內存管理:手動控制減少碎片,提高性能。 2)編譯時優化:內聯函數和循環展開提昇運行速度。 3)低級操作:直接訪問硬件,優化圖形和物理計算。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

SublimeText3 Linux新版
SublimeText3 Linux最新版

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

Atom編輯器mac版下載
最受歡迎的的開源編輯器

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。