在物件導向程式設計中測試 GoLang 函數的策略有:單元測試:隔離測試單一函數及其相依性。表驅動測試:使用表格資料簡化測試案例定義。整合測試:測試多個函數的組合及其相依性。基準測試:衡量函數的效能並優化瓶頸。
GoLang 函數在物件導向程式設計中的測試策略實戰
在物件導向程式設計中,測試函數是確保程式碼可靠性和準確性的關鍵。 GoLang 提供了多種策略來測試函數,這有助於提高程式碼覆蓋率並防止錯誤。
單元測試
單元測試是測試單一函數及其相依性的隔離方法。它們使用testing
套件,如下所示:
import "testing" func TestAdd(t *testing.T) { tests := []struct { a, b, expected int }{ {1, 2, 3}, {-1, 0, -1}, } for _, tt := range tests { t.Run(fmt.Sprintf("%d + %d", tt.a, tt.b), func(t *testing.T) { actual := Add(tt.a, tt.b) if actual != tt.expected { t.Errorf("Add(%d, %d) = %d, want %d", tt.a, tt.b, actual, tt.expected) } }) } }
表格驅動測試
表格驅動測試是單元測試的變體,使用表格形式的測試數據。這簡化了測試案例定義並提高了可讀性:
import "testing" func TestAdd(t *testing.T) { tests := []struct { a, b, expected int }{ {1, 2, 3}, {-1, 0, -1}, } for _, tt := range tests { actual := Add(tt.a, tt.b) if actual != tt.expected { t.Errorf("Add(%d, %d) = %d, want %d", tt.a, tt.b, actual, tt.expected) } } }
整合測試
#整合測試測試多個函數的組合,包括它們的依賴項。它們模擬現實世界的使用場景,如下所示:
import ( "testing" "net/http" "net/http/httptest" ) func TestHandleAdd(t *testing.T) { req, _ := http.NewRequest("GET", "/add?a=1&b=2", nil) rr := httptest.NewRecorder() HandleAdd(rr, req) expected := "3" if rr.Body.String() != expected { t.Errorf("HandleAdd() = %s, want %s", rr.Body.String(), expected) } }
基準測試
基準測試衡量函數的效能,識別效能瓶頸並進行最佳化。它們使用 testing/benchmark
套件,如下所示:
import "testing" func BenchmarkAdd(b *testing.B) { for i := 0; i < b.N; i++ { Add(1, 2) } }
透過應用這些測試策略,開發者可以確保 GoLang 函數在物件導向程式設計中平穩運行並產生準確的結果。
以上是golang函數在物件導向程式設計中的測試策略的詳細內容。更多資訊請關注PHP中文網其他相關文章!
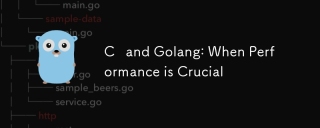
C 更適合需要直接控制硬件資源和高性能優化的場景,而Golang更適合需要快速開發和高並發處理的場景。 1.C 的優勢在於其接近硬件的特性和高度的優化能力,適合遊戲開發等高性能需求。 2.Golang的優勢在於其簡潔的語法和天然的並發支持,適合高並發服務開發。
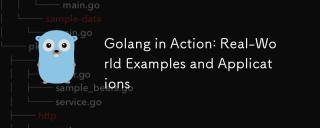
Golang在实际应用中表现出色,以简洁、高效和并发性著称。1)通过Goroutines和Channels实现并发编程,2)利用接口和多态编写灵活代码,3)使用net/http包简化网络编程,4)构建高效并发爬虫,5)通过工具和最佳实践进行调试和优化。
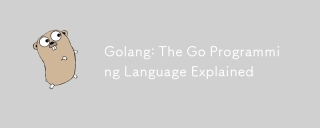
Go語言的核心特性包括垃圾回收、靜態鏈接和並發支持。 1.Go語言的並發模型通過goroutine和channel實現高效並發編程。 2.接口和多態性通過實現接口方法,使得不同類型可以統一處理。 3.基本用法展示了函數定義和調用的高效性。 4.高級用法中,切片提供了動態調整大小的強大功能。 5.常見錯誤如競態條件可以通過gotest-race檢測並解決。 6.性能優化通過sync.Pool重用對象,減少垃圾回收壓力。
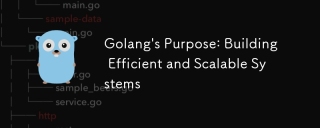
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
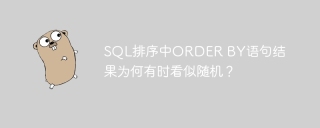
關於SQL查詢結果排序的疑惑學習SQL的過程中,常常會遇到一些令人困惑的問題。最近,筆者在閱讀《MICK-SQL基礎�...
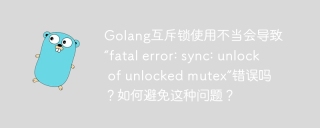
golang ...
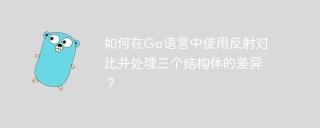
Go語言中如何對比並處理三個結構體在Go語言編程中,有時需要對比兩個結構體的差異,並將這些差異應用到第�...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

記事本++7.3.1
好用且免費的程式碼編輯器

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具

SublimeText3漢化版
中文版,非常好用