C 中函數重載允許在同一類別中定義同名函數,但參數列表不同;函數重寫發生在子類別中定義一個與父類別同名且參數相同的函數,子類別函數將覆蓋父類別函數。在實戰範例中,重載函數用於針對不同資料類型執行加法運算,重寫函數用於覆蓋父類別中的虛擬函數,以計算不同形狀的面積。
C 函數重載與重寫:深入理解與實戰應用
函數重載
函數重載允許在同一類別中定義擁有相同函數名稱但參數清單不同的多個函數。
class MyClass { public: int add(int a, int b); double add(double a, double b); }; int MyClass::add(int a, int b) { return a + b; } double MyClass::add(double a, double b) { return a + b; }
函數重寫
函數重寫發生在子類別中定義一個與父類別同名且具有相同參數清單的函數時。子類別函數將覆蓋父類別函數。
class ParentClass { public: virtual int display() { return 10; } }; class ChildClass : public ParentClass { public: int display() { // 重写父类的 display() return 20; } };
實戰案例
重載函數範例:
#include <iostream> class Calculator { public: int add(int a, int b) { return a + b; } double add(double a, double b) { return a + b; } std::string add(std::string a, std::string b) { return a + b; } }; int main() { Calculator calc; std::cout << calc.add(1, 2) << std::endl; // 输出:3 std::cout << calc.add(1.5, 2.5) << std::endl; // 输出:4 std::cout << calc.add("Hello", "World") << std::endl; // 输出:HelloWorld return 0; }
重寫函數範例:
#include <iostream> class Shape { public: virtual double area() = 0; // 纯虚函数(强制子类实现 area()) }; class Rectangle : public Shape { public: Rectangle(double width, double height) : m_width(width), m_height(height) {} double area() override { return m_width * m_height; } private: double m_width; double m_height; }; class Circle : public Shape { public: Circle(double radius) : m_radius(radius) {} double area() override { return 3.14 * m_radius * m_radius; } private: double m_radius; }; int main() { Rectangle rect(5, 10); Circle circle(5); std::cout << "Rectangle area: " << rect.area() << std::endl; // 输出:50 std::cout << "Circle area: " << circle.area() << std::endl; // 输出:78.5 return 0; }
以上是C++ 函式重載和重寫的理解和使用的詳細內容。更多資訊請關注PHP中文網其他相關文章!
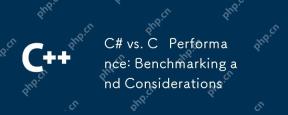
C#和C 在性能上的差異主要體現在執行速度和資源管理上:1)C 在數值計算和字符串操作上通常表現更好,因為它更接近硬件,沒有垃圾回收等額外開銷;2)C#在多線程編程上更為簡潔,但性能略遜於C ;3)選擇哪種語言應根據項目需求和團隊技術棧決定。
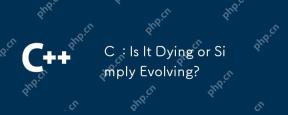
1)c relevantduetoItsAverity and效率和效果臨界。 2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
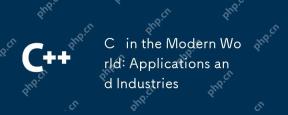
C 在現代世界中的應用廣泛且重要。 1)在遊戲開發中,C 因其高性能和多態性被廣泛使用,如UnrealEngine和Unity。 2)在金融交易系統中,C 的低延遲和高吞吐量使其成為首選,適用於高頻交易和實時數據分析。
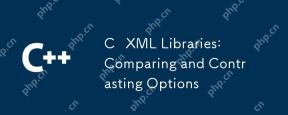
C 中有四種常用的XML庫:TinyXML-2、PugiXML、Xerces-C 和RapidXML。 1.TinyXML-2適合資源有限的環境,輕量但功能有限。 2.PugiXML快速且支持XPath查詢,適用於復雜XML結構。 3.Xerces-C 功能強大,支持DOM和SAX解析,適用於復雜處理。 4.RapidXML專注於性能,解析速度極快,但不支持XPath查詢。
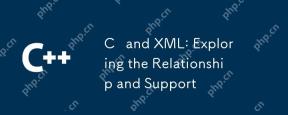
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
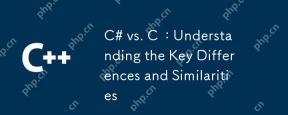
C#和C 的主要區別在於語法、性能和應用場景。 1)C#語法更簡潔,支持垃圾回收,適用於.NET框架開發。 2)C 性能更高,需手動管理內存,常用於系統編程和遊戲開發。
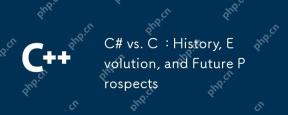
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
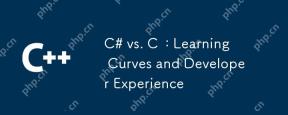
C#和C 的学习曲线和开发者体验有显著差异。1)C#的学习曲线较平缓,适合快速开发和企业级应用。2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
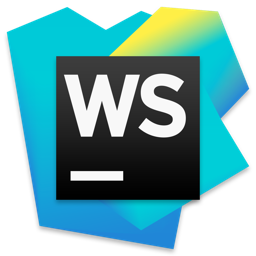
WebStorm Mac版
好用的JavaScript開發工具

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!
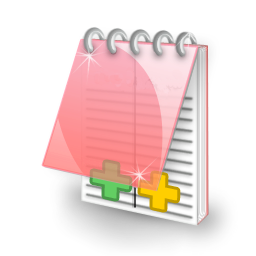
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能

記事本++7.3.1
好用且免費的程式碼編輯器