為 C 函式進行基準測試,可採取下列步驟:使用計時工具(如 std::chrono 函式庫)測量執行時間。編寫基準測試函數以執行程式碼並傳回執行時間。利用基準測試庫取得進階功能,如統計收集和比較。
如何對C 函數效能進行基準測試
基準測試是測量程式碼效能並比較不同實作的重要技術。在C 中,我們可以透過以下方法對函數效能進行基準測試:
1. 使用計時工具
C 提供了std::chrono
庫,其中包含用於衡量時間的類別。我們可以使用std::chrono::high_resolution_clock
來取得高精確度計時:
#include <chrono> using namespace std::chrono; auto start = high_resolution_clock::now(); // 待测试代码 auto end = high_resolution_clock::now();
2. 寫基準測試函數
#寫一個函數來執行要測試的程式碼並傳回執行時間:
#include <chrono> using namespace std::chrono; double benchmark(int n) { auto start = high_resolution_clock::now(); // 待测试代码 auto end = high_resolution_clock::now(); return duration_cast<duration<double>>(end - start).count(); }
3. 使用基準測試函式庫
還有各種C 基準測試函式庫可供使用,它們提供更高級的功能,如統計收集和比較。以下是一些受歡迎的函式庫:
- [benchmark](https://github.com/google/benchmark)
- [boost::benchmark](https://www .boost.org/doc/libs/1_65_1/libs/benchmark/doc/html/index.html)
- #[google-benchmark](https://github.com/google/benchmark)
- [Catch2](https://github.com/catchorg/Catch2)
#實戰案例:
假設我們要基準測試一個查找給定數組中元素的函數find_element()
:
#include <chrono> #include <vector> using namespace std::chrono; double find_element_benchmark(size_t n) { // 生成一个包含 n 个元素的数组 std::vector<int> arr(n, 0); // 查找一个不存在的元素 auto start = high_resolution_clock::now(); auto pos = std::find(arr.begin(), arr.end(), -1); auto end = high_resolution_clock::now(); if (pos != arr.end()) return -1; // 仅在元素找到时返回 -1 return duration_cast<duration<double>>(end - start).count(); } int main() { // 多次测试不同数组大小 for (size_t n = 1000; n <= 1000000; n *= 10) { // 运行基准测试 double time = find_element_benchmark(n); // 打印结果 std::cout << "数组大小: " << n << "\t执行时间: " << time << " 秒" << std::endl; } return 0; }
以上是如何對 C++ 函數效能進行基準測試?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
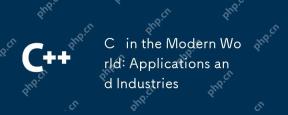
C 在現代世界中的應用廣泛且重要。 1)在遊戲開發中,C 因其高性能和多態性被廣泛使用,如UnrealEngine和Unity。 2)在金融交易系統中,C 的低延遲和高吞吐量使其成為首選,適用於高頻交易和實時數據分析。
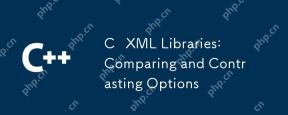
C 中有四種常用的XML庫:TinyXML-2、PugiXML、Xerces-C 和RapidXML。 1.TinyXML-2適合資源有限的環境,輕量但功能有限。 2.PugiXML快速且支持XPath查詢,適用於復雜XML結構。 3.Xerces-C 功能強大,支持DOM和SAX解析,適用於復雜處理。 4.RapidXML專注於性能,解析速度極快,但不支持XPath查詢。
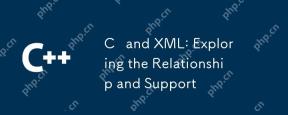
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
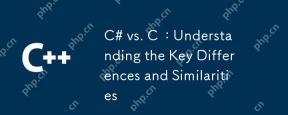
C#和C 的主要區別在於語法、性能和應用場景。 1)C#語法更簡潔,支持垃圾回收,適用於.NET框架開發。 2)C 性能更高,需手動管理內存,常用於系統編程和遊戲開發。
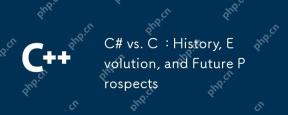
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
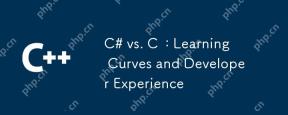
C#和C 的学习曲线和开发者体验有显著差异。1)C#的学习曲线较平缓,适合快速开发和企业级应用。2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
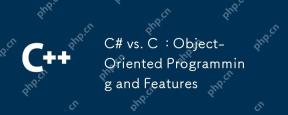
C#和C 在面向对象编程(OOP)中的实现方式和特性上有显著差异。1)C#的类定义和语法更为简洁,支持如LINQ等高级特性。2)C 提供更细粒度的控制,适用于系统编程和高性能需求。两者各有优势,选择应基于具体应用场景。
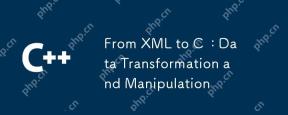
從XML轉換到C 並進行數據操作可以通過以下步驟實現:1)使用tinyxml2庫解析XML文件,2)將數據映射到C 的數據結構中,3)使用C 標準庫如std::vector進行數據操作。通過這些步驟,可以高效地處理和操作從XML轉換過來的數據。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

SublimeText3漢化版
中文版,非常好用

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),