在 Go 語言中傳送 POST 請求,可以按照以下步驟進行:匯入必要的套件。建立一個 http 客戶端。建立一個 http 請求,指定方法、URL 和請求正文。設定必要的請求標頭。執行請求並取得回應。處理響應正文。
有效率地執行 Go 語言中的 POST 請求
POST 請求在與 web 伺服器互動時非常有用,例如提交表單或建立新資源。在 Go 語言中,使用 net/http
套件輕鬆傳送 POST 請求。
1. 導入必要的套件
import "net/http"
2. 建立http
客戶端
建立一個http
用戶端來處理請求:
client := http.Client{}
3. 建立http
請求
使用http.NewRequest
建立一個新的http
請求,指定方法、URL 和請求正文(如果需要):
req, err := http.NewRequest("POST", "https://example.com", body) if err != nil { // 处理错误 }
4. 設定請求標頭
為請求設定任何必要的標頭,例如Content-Type
:
req.Header.Set("Content-Type", "application/json")
5. 執行請求
使用client.Do
執行請求並取得回應:
resp, err := client.Do(req) if err != nil { // 处理错误 }
6. 處理回應
使用resp.Body
讀取並處理回應正文:
defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { // 处理错误 } fmt.Println(string(body))
實戰案例:建立新使用者
考慮一個API,其中我們需要使用POST 請求建立新使用者:
const userURL = "https://example.com/api/v1/users" type User struct { Name string `json:"name"` } func main() { client := http.Client{} user := User{ Name: "My New User", } jsonBytes, err := json.Marshal(user) if err != nil { // 处理错误 } req, err := http.NewRequest("POST", userURL, bytes.NewReader(jsonBytes)) if err != nil { // 处理错误 } req.Header.Set("Content-Type", "application/json") resp, err := client.Do(req) if err != nil { // 处理错误 } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { // 处理错误 } fmt.Println(string(body)) }
在上面的範例中,我們首先建立了一個User
結構體來表示新使用者。然後,我們將使用者資料序列化為 JSON 並建立了一個新的 http.Request
。最後,我們執行請求並處理回應。
以上是有效率地執行 Go 語言中的 POST 請求的詳細內容。更多資訊請關注PHP中文網其他相關文章!
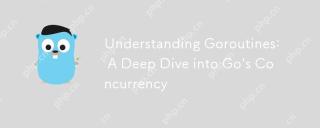
goroutinesarefunctionsormethodsthatruncurranceingo,啟用效率和燈威量。 1)shememanagedbodo'sruntimemultimusingmultiplexing,允許千sstorunonfewerosthreads.2)goroutinessimproverentimensImproutinesImproutinesImproveranceThroutinesImproveranceThrountinesimproveranceThroundinesImproveranceThroughEasySytaskParallowalizationAndeff
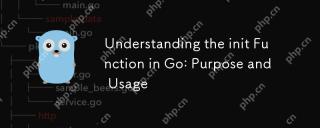
purposeoftheInitfunctionoIsistoInitializeVariables,setUpConfigurations,orperformneccesSetarySetupBeforEtheMainFunctionExeCutes.useInitby.UseInitby:1)placingitinyourcodetorunautoamenationally oneraty oneraty oneraty on inity in ofideShortAndAndAndAndForemain,2)keepitiTshortAntAndFocusedonSimImimpletasks,3)
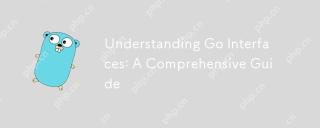
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
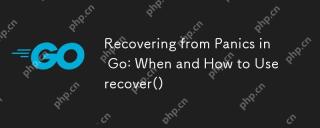
在Go中使用recover()函數可以從panic中恢復。具體方法是:1)在defer函數中使用recover()捕獲panic,避免程序崩潰;2)記錄詳細的錯誤信息以便調試;3)根據具體情況決定是否恢復程序執行;4)謹慎使用,以免影響性能。
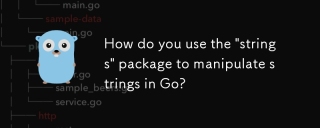
本文討論了使用GO的“字符串”軟件包進行字符串操作,詳細介紹了共同的功能和最佳實踐,以提高效率並有效地處理Unicode。
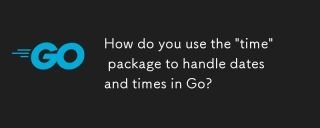
本文詳細介紹了GO的“時間”包用於處理日期,時間和時區,包括獲得當前時間,創建特定時間,解析字符串以及測量經過的時間。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具
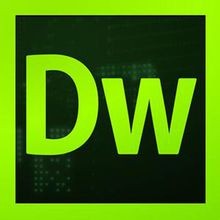
Dreamweaver CS6
視覺化網頁開發工具

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。