Go語言程式設計指南:單鍊錶實作詳解
在Go語言中,單鍊錶是一種常見的資料結構,用於儲存一系列元素並按順序訪問。本文將詳細介紹單鍊錶的實作原理,並給出具體的Go語言程式碼範例。
單鍊錶的定義
單鍊錶是一種線性表的資料結構,其中的每個元素(節點)包含兩部分:資料域和指標域。資料域用於儲存元素的值,指標域則指向下一個節點。最後一個節點的指標域通常為空,表示鍊錶的結束。
單鍊錶的節點定義
首先,我們定義一個單鍊錶的節點類型:
type Node struct { data int next *Node }
其中,data
欄位儲存節點的值, next
欄位儲存指向下一個節點的指標。
單鍊錶的初始化
接下來,我們定義單鍊錶的初始化函數:
type LinkedList struct { head *Node } func NewLinkedList() *LinkedList { return &LinkedList{} }
在初始化函數中,我們建立一個空的鍊錶,並將頭節點指針初始化為空。
單鍊錶的插入操作
實作單鍊錶的插入操作可以分為兩種情況:在鍊錶頭部插入節點和在鍊錶尾部插入節點。
首先是在鍊錶頭部插入節點的函數:
func (list *LinkedList) InsertAtBeginning(value int) { newNode := &Node{data: value} newNode.next = list.head list.head = newNode }
在這個函數中,我們首先建立一個新節點並將其值初始化為傳入的數值。然後將新節點的指標指向鍊錶頭部,最後更新鍊錶的頭節點為新節點。
接下來是在鍊錶尾部插入節點的函數:
func (list *LinkedList) InsertAtEnd(value int) { newNode := &Node{data: value} if list.head == nil { list.head = newNode return } current := list.head for current.next != nil { current = current.next } current.next = newNode }
這個函數先建立一個新節點,並判斷鍊錶是否為空。如果為空,則直接將新節點設為頭節點;否則,遍歷鍊錶直到找到最後一個節點,然後將新節點插入到最後一個節點的後面。
單一鍊錶的刪除操作
刪除操作分為兩種情況:刪除頭節點和刪除指定數值的節點。
首先是刪除頭節點的函數:
func (list *LinkedList) DeleteAtBeginning() { if list.head == nil { return } list.head = list.head.next }
這個函數直接將頭節點指標指向下一個節點,因此刪除了頭節點。
接下來是刪除指定數值的節點的函數:
func (list *LinkedList) DeleteByValue(value int) { if list.head == nil { return } if list.head.data == value { list.head = list.head.next return } prev := list.head current := list.head.next for current != nil { if current.data == value { prev.next = current.next return } prev = current current = current.next } }
這個函數中,我們需要先判斷鍊錶是否為空。然後從頭節點開始遍歷鍊錶,找到目標數值所在的節點並刪除。
單一鍊錶的遍歷操作
最後是單鍊錶的遍歷操作:
func (list *LinkedList) Print() { current := list.head for current != nil { fmt.Print(current.data, " ") current = current.next } fmt.Println() }
這個函數從頭節點開始逐一列印節點的值,直到鍊錶結束。
範例程式碼
下面是一個完整的範例程式碼,示範如何使用單鍊錶:
package main import "fmt" type Node struct { data int next *Node } type LinkedList struct { head *Node } func NewLinkedList() *LinkedList { return &LinkedList{} } func (list *LinkedList) InsertAtBeginning(value int) { newNode := &Node{data: value} newNode.next = list.head list.head = newNode } func (list *LinkedList) InsertAtEnd(value int) { newNode := &Node{data: value} if list.head == nil { list.head = newNode return } current := list.head for current.next != nil { current = current.next } current.next = newNode } func (list *LinkedList) DeleteAtBeginning() { if list.head == nil { return } list.head = list.head.next } func (list *LinkedList) DeleteByValue(value int) { if list.head == nil { return } if list.head.data == value { list.head = list.head.next return } prev := list.head current := list.head.next for current != nil { if current.data == value { prev.next = current.next return } prev = current current = current.next } } func (list *LinkedList) Print() { current := list.head for current != nil { fmt.Print(current.data, " ") current = current.next } fmt.Println() } func main() { list := NewLinkedList() list.InsertAtEnd(1) list.InsertAtEnd(2) list.InsertAtEnd(3) list.Print() list.DeleteByValue(2) list.Print() list.DeleteAtBeginning() list.Print() }
以上就是使用Go語言實作單鍊錶的詳粵指南。希望透過本文的介紹和範例程式碼,讀者能夠更深入地理解單鍊錶的原理和實作方式。
以上是Go語言程式設計指南:單鍊錶實作詳解的詳細內容。更多資訊請關注PHP中文網其他相關文章!
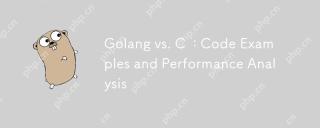
Golang適合快速開發和並發編程,而C 更適合需要極致性能和底層控制的項目。 1)Golang的並發模型通過goroutine和channel簡化並發編程。 2)C 的模板編程提供泛型代碼和性能優化。 3)Golang的垃圾回收方便但可能影響性能,C 的內存管理複雜但控制精細。
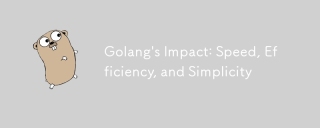
goimpactsdevelopmentpositationality throughspeed,效率和模擬性。 1)速度:gocompilesquicklyandrunseff,IdealforlargeProjects.2)效率:效率:ITScomprehenSevestAndardArdardArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增強的Depleflovelmentimency.3)簡單性。
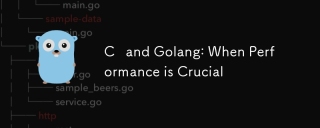
C 更適合需要直接控制硬件資源和高性能優化的場景,而Golang更適合需要快速開發和高並發處理的場景。 1.C 的優勢在於其接近硬件的特性和高度的優化能力,適合遊戲開發等高性能需求。 2.Golang的優勢在於其簡潔的語法和天然的並發支持,適合高並發服務開發。
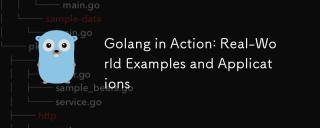
Golang在实际应用中表现出色,以简洁、高效和并发性著称。1)通过Goroutines和Channels实现并发编程,2)利用接口和多态编写灵活代码,3)使用net/http包简化网络编程,4)构建高效并发爬虫,5)通过工具和最佳实践进行调试和优化。
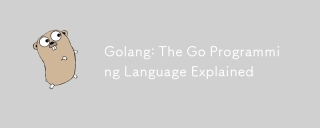
Go語言的核心特性包括垃圾回收、靜態鏈接和並發支持。 1.Go語言的並發模型通過goroutine和channel實現高效並發編程。 2.接口和多態性通過實現接口方法,使得不同類型可以統一處理。 3.基本用法展示了函數定義和調用的高效性。 4.高級用法中,切片提供了動態調整大小的強大功能。 5.常見錯誤如競態條件可以通過gotest-race檢測並解決。 6.性能優化通過sync.Pool重用對象,減少垃圾回收壓力。
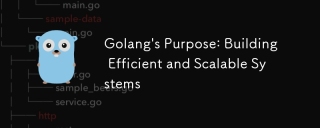
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
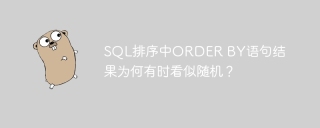
關於SQL查詢結果排序的疑惑學習SQL的過程中,常常會遇到一些令人困惑的問題。最近,筆者在閱讀《MICK-SQL基礎�...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
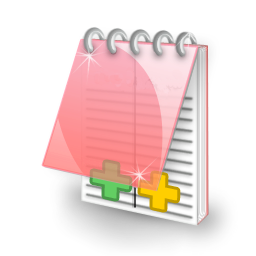
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能
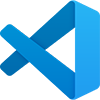
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

SublimeText3 Linux新版
SublimeText3 Linux最新版

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中