Vue元件實戰:資料篩選元件開發
在Vue開發中,資料篩選是常用的功能之一。本文將帶您詳細了解Vue組件實戰:資料篩選組件的開發,透過具體的程式碼實例來示範其實現過程,幫助您深入理解Vue組件的使用方法。
首先,我們需要明確需求,就是開發一個資料篩選元件,可以在前端進行簡單的篩選操作,包括輸入框、多選框、日期選擇、範圍選擇等方式,滿足不同場景下的數據篩選需求。
根據需求,我們可以將元件拆分為以下幾個部分:
- 輸入框篩選
程式碼如下:
<template> <div class="input-filter"> <input type="text" v-model="value" placeholder="请输入关键词" @input="changeInput"> <button @click="search">搜索</button> </div> </template> <script> export default { data() { return { value: "" }; }, methods: { changeInput(event) { this.value = event.target.value; }, search() { this.$emit("search", this.value); } } }; </script> <style scoped> .input-filter { display: flex; margin-bottom: 10px; align-items: center; justify-content: center; } .input-filter input { margin-right: 10px; padding: 5px; border-radius: 4px; border: 1px solid #ccc; font-size: 14px; } .input-filter button { padding: 5px 10px; border-radius: 4px; background-color: #1989fa; color: #fff; border: none; font-size: 14px; } </style>
此元件包含一個輸入框和搜尋按鈕,使用者在輸入框中輸入關鍵字,點擊搜尋按鈕後將觸發search
事件,並將搜尋關鍵字傳遞給父元件。
- 多重選取框篩選
程式碼如下:
<template> <div class="checkbox-filter"> <div class="title">{{ title }}</div> <el-checkbox-group v-model="checkedList" @change="handleChange"> <el-checkbox v-for="item in options" :label="item.value" :key="item.value">{{ item.label }}</el-checkbox> </el-checkbox-group> </div> </template> <script> export default { props: { title: { type: String, default: "" }, options: { type: Array, default: () => [] } }, data() { return { checkedList: [] }; }, methods: { handleChange(checkedList) { this.$emit("change", checkedList); } } }; </script> <style scoped> .checkbox-filter { margin-bottom: 10px; } .checkbox-filter .title { font-size: 16px; font-weight: bold; margin-bottom: 5px; } </style>
此元件包含一個多重選取方塊和一個標題,使用者在多重選取方塊中選擇需要篩選的選項後,將觸發change
事件,並傳遞選取的選項給父元件。
- 日期選擇篩選
程式碼如下:
<template> <div class="date-filter"> <el-row :gutter="10"> <el-col :span="12"> <el-date-picker v-model="start" type="date" placeholder="开始日期" @change="handleChange" /> </el-col> <el-col :span="12"> <el-date-picker v-model="end" type="date" placeholder="结束日期" @change="handleChange" /> </el-col> </el-row> </div> </template> <script> export default { data() { return { start: "", end: "" }; }, methods: { handleChange() { this.$emit("change", { start: this.start, end: this.end }); } } }; </script> <style scoped> .date-filter { margin-bottom: 10px; } </style>
此元件包含兩個日期選擇器,使用者可以選擇起始日期和結束日期,選取後將觸發change
事件,並將選取的日期範圍傳遞給父元件。
- 範圍選擇篩選
程式碼如下:
<template> <div class="range-filter"> <el-row :gutter="10"> <el-col :span="12"> <el-input-number v-model.number="min" controls-position="right" :min="0" :step="1" @change="handleChange" /> </el-col> <el-col :span="12"> <el-input-number v-model.number="max" controls-position="right" :min="0" :step="1" @change="handleChange" /> </el-col> </el-row> </div> </template> <script> export default { data() { return { min: 0, max: 0 }; }, methods: { handleChange() { this.$emit("change", { min: this.min, max: this.max }); } } }; </script> <style scoped> .range-filter { margin-bottom: 10px; } </style>
此元件包含兩個數字輸入框,使用者可以選擇數值範圍,並在勾選後觸發change
事件,並將選取的範圍傳遞給父元件。
以上四個元件可以組合起來使用,實現多維度資料的篩選。在父元件中,我們可以將這些子元件結合起來,完成完整的資料篩選功能。
程式碼如下:
<template> <div class="filter-container"> <input-filter @search="onSearch" /> <checkbox-filter :title="title1" :options="options1" @change="onChange1" /> <date-filter @change="onChange2" /> <range-filter @change="onChange3" /> </div> </template> <script> import InputFilter from "./InputFilter.vue"; import CheckboxFilter from "./CheckboxFilter.vue"; import DateFilter from "./DateFilter.vue"; import RangeFilter from "./RangeFilter.vue"; export default { components: { InputFilter, CheckboxFilter, DateFilter, RangeFilter }, data() { return { title1: "多选框筛选", options1: [ { label: "选项1", value: 1 }, { label: "选项2", value: 2 }, { label: "选项3", value: 3 } ] }; }, methods: { onSearch(value) { console.log("搜索关键词:", value); }, onChange1(value) { console.log("多选框选中的值:", value); }, onChange2(value) { console.log("日期选择范围:", value); }, onChange3(value) { console.log("范围选择范围:", value); } } }; </script> <style scoped> .filter-container { margin: 20px; } </style>
這裡只是簡單示範了一些篩選元件的範例,您可以根據實際需求進行組合和擴展,豐富您的資料篩選能力。
總結
本文詳細介紹了Vue元件實戰:資料篩選元件的開發,並提供了多個具體的程式碼範例,讓讀者更能理解Vue元件的使用方法。在日常開發中,遇到資料篩選的需求,可以透過以上元件實現,提高開發效率和使用者體驗。
以上是Vue組件實戰:資料篩選組件開發的詳細內容。更多資訊請關注PHP中文網其他相關文章!

Vue.js受歡迎的原因包括簡單易學、靈活性高和高效性能。 1)其漸進式框架設計適合初學者逐步學習。 2)組件化開發提高了代碼可維護性和團隊協作效率。 3)響應式系統和虛擬DOM提升了渲染性能。

Vue.js更易用且學習曲線較平緩,適合初學者;React學習曲線較陡峭,但靈活性強,適合有經驗的開發者。 1.Vue.js通過簡單的數據綁定和漸進式設計易於上手。 2.React需要理解虛擬DOM和JSX,但提供更高的靈活性和性能優勢。

Vue.js適合快速開發和小型項目,而React更適合大型和復雜的項目。 1.Vue.js簡單易學,適用於快速開發和小型項目。 2.React功能強大,適合大型和復雜的項目。 3.Vue.js的漸進式特性適合逐步引入功能。 4.React的組件化和虛擬DOM在處理複雜UI和數據密集型應用時表現出色。

Vue.js和React各有優缺點,選擇時需綜合考慮團隊技能、項目規模和性能需求。 1)Vue.js適合快速開發和小型項目,學習曲線低,但深層嵌套對象可能導致性能問題。 2)React適用於大型和復雜應用,生態系統豐富,但頻繁更新可能導致性能瓶頸。

Vue.js適合小型到中型項目,React適合大型項目和復雜應用場景。 1)Vue.js易於上手,適用於快速原型開發和小型應用。 2)React在處理複雜狀態管理和性能優化方面更有優勢,適合大型項目。

Vue.js和React各有優勢:Vue.js適用於小型應用和快速開發,React適合大型應用和復雜狀態管理。 1.Vue.js通過響應式系統實現自動更新,適用於小型應用。 2.React使用虛擬DOM和diff算法,適合大型和復雜應用。選擇框架時需考慮項目需求和團隊技術棧。

Vue.js和React各有優勢,選擇應基於項目需求和團隊技術棧。 1.Vue.js社區友好,提供豐富學習資源,生態系統包括VueRouter等官方工具,支持由官方團隊和社區提供。 2.React社區偏向企業應用,生態系統強大,支持由Facebook及其社區提供,更新頻繁。

Netflix使用React來提升用戶體驗。 1)React的組件化特性幫助Netflix將復雜UI拆分成可管理模塊。 2)虛擬DOM優化了UI更新,提高了性能。 3)結合Redux和GraphQL,Netflix高效管理應用狀態和數據流動。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

SublimeText3漢化版
中文版,非常好用

記事本++7.3.1
好用且免費的程式碼編輯器
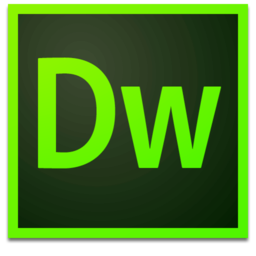
Dreamweaver Mac版
視覺化網頁開發工具