React資料流管理指南:如何優雅地處理前端資料流
#引言:
React是一種非常流行的前端開發框架,它提供了一種組件化的開發方式,使得前端開發更加模組化、可維護性更高。然而,在開發複雜的應用程式時,管理資料流動變得非常重要。這篇文章將介紹一些React中優雅處理資料流動的方法,並示範具體的程式碼範例。
一、單向資料流
React倡議使用單向資料流來管理資料流。單向資料流的概念很簡單:資料只能從父元件流向子元件,子元件不能直接修改父元件傳遞過來的資料。這種資料流動的模式使得資料的流向更加清晰,便於調試和維護。
下面是一個簡單的範例,說明了單向資料流的使用:
class ParentComponent extends React.Component { constructor() { super(); this.state = { count: 0 }; } incrementCount() { this.setState(prevState => ({ count: prevState.count + 1 })); } render() { return ( <div> <ChildComponent count={this.state.count} /> <button onClick={() => this.incrementCount()}>增加计数</button> </div> ); } } class ChildComponent extends React.Component { render() { return ( <div> 当前计数:{this.props.count} </div> ); } }
在這個範例中,父元件ParentComponent的state中的count變數被傳遞給了子元件ChildComponent 。當點擊增加計數按鈕時,父元件會呼叫incrementCount方法來更新state中的count變數。然後,父元件重新渲染,同時將更新後的count傳遞給子元件。子元件根據新的props值進行重新渲染,並顯示最新的計數。
二、使用狀態管理工具
當應用程式變得複雜時,僅使用父子元件的props傳遞資料可能不夠靈活。這時可以考慮使用狀態管理工具來更好地管理資料流動。
Redux是一個非常流行的狀態管理工具,它提供了強大的資料流管理功能。以下是一個使用Redux的範例:
// store.js import { createStore } from 'redux'; const initialState = { count: 0 }; const reducer = (state = initialState, action) => { switch (action.type) { case 'INCREMENT': return { ...state, count: state.count + 1 }; default: return state; } }; const store = createStore(reducer); export default store;
// index.js import React from 'react'; import ReactDOM from 'react-dom'; import { Provider } from 'react-redux'; import store from './store'; import App from './App'; ReactDOM.render( <Provider store={store}> <App /> </Provider>, document.getElementById('root') );
// App.js import React from 'react'; import { connect } from 'react-redux'; class App extends React.Component { render() { return ( <div> 当前计数:{this.props.count} <button onClick={this.props.increment}>增加计数</button> </div> ); } } const mapStateToProps = state => ({ count: state.count }); const mapDispatchToProps = dispatch => ({ increment: () => dispatch({ type: 'INCREMENT' }) }); export default connect(mapStateToProps, mapDispatchToProps)(App);
在這個範例中,我們使用createStore函數建立了一個Redux store,並使用Provider元件將其傳遞給應用程式的根元件。根元件中透過connect函數將store中的狀態對應到應用程式中的元件,同時將dispatch函數對應到元件的props中,以實現狀態的更新。
這種方式使得資料的管理更加靈活,能夠輕鬆處理複雜的資料流動情況。
結論:
在React中優雅地處理資料流動是非常重要的,它能夠使你的應用程式更易於維護和擴展。本文介紹了使用單向資料流和狀態管理工具Redux來處理資料流動的方法,並提供了具體的程式碼範例。希望能夠對你在React專案中的資料管理有所幫助!
以上是React資料流管理指南:如何優雅地處理前端資料流動的詳細內容。更多資訊請關注PHP中文網其他相關文章!
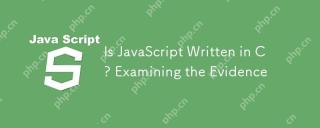
是的,JavaScript的引擎核心是用C語言編寫的。 1)C語言提供了高效性能和底層控制,適合JavaScript引擎的開發。 2)以V8引擎為例,其核心用C 編寫,結合了C的效率和麵向對象特性。 3)JavaScript引擎的工作原理包括解析、編譯和執行,C語言在這些過程中發揮關鍵作用。
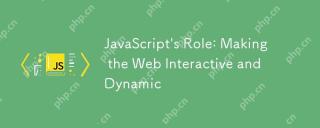
JavaScript是現代網站的核心,因為它增強了網頁的交互性和動態性。 1)它允許在不刷新頁面的情況下改變內容,2)通過DOMAPI操作網頁,3)支持複雜的交互效果如動畫和拖放,4)優化性能和最佳實踐提高用戶體驗。
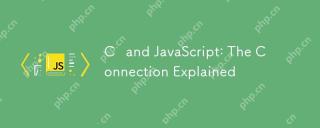
C 和JavaScript通過WebAssembly實現互操作性。 1)C 代碼編譯成WebAssembly模塊,引入到JavaScript環境中,增強計算能力。 2)在遊戲開發中,C 處理物理引擎和圖形渲染,JavaScript負責遊戲邏輯和用戶界面。
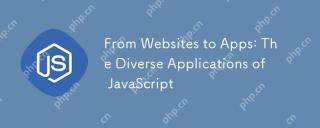
JavaScript在網站、移動應用、桌面應用和服務器端編程中均有廣泛應用。 1)在網站開發中,JavaScript與HTML、CSS一起操作DOM,實現動態效果,並支持如jQuery、React等框架。 2)通過ReactNative和Ionic,JavaScript用於開發跨平台移動應用。 3)Electron框架使JavaScript能構建桌面應用。 4)Node.js讓JavaScript在服務器端運行,支持高並發請求。
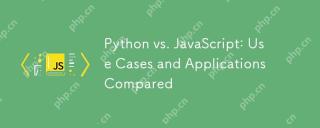
Python更適合數據科學和自動化,JavaScript更適合前端和全棧開發。 1.Python在數據科學和機器學習中表現出色,使用NumPy、Pandas等庫進行數據處理和建模。 2.Python在自動化和腳本編寫方面簡潔高效。 3.JavaScript在前端開發中不可或缺,用於構建動態網頁和單頁面應用。 4.JavaScript通過Node.js在後端開發中發揮作用,支持全棧開發。
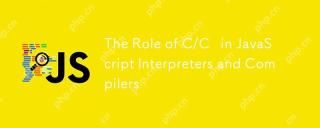
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。1)C 用于解析JavaScript源码并生成抽象语法树。2)C 负责生成和执行字节码。3)C 实现JIT编译器,在运行时优化和编译热点代码,显著提高JavaScript的执行效率。
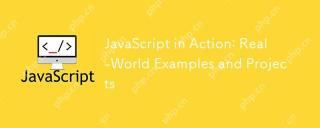
JavaScript在現實世界中的應用包括前端和後端開發。 1)通過構建TODO列表應用展示前端應用,涉及DOM操作和事件處理。 2)通過Node.js和Express構建RESTfulAPI展示後端應用。
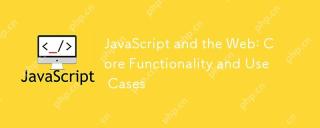
JavaScript在Web開發中的主要用途包括客戶端交互、表單驗證和異步通信。 1)通過DOM操作實現動態內容更新和用戶交互;2)在用戶提交數據前進行客戶端驗證,提高用戶體驗;3)通過AJAX技術實現與服務器的無刷新通信。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
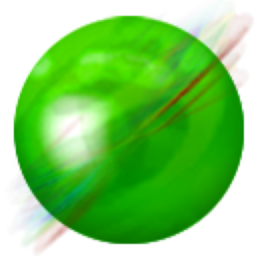
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

記事本++7.3.1
好用且免費的程式碼編輯器

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。
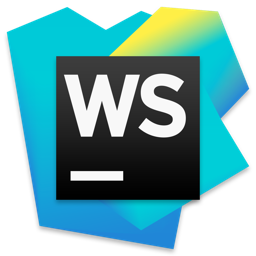
WebStorm Mac版
好用的JavaScript開發工具