請考慮下表以了解不同公司的資格標準 -
CGPA | 的中文翻譯為:績點平均成績 |
符合條件的公司 |
---|---|---|
#大於或等於8 |
#Google、微軟、亞馬遜、戴爾、英特爾、Wipro |
|
大於或等於7 |
#教學點、accenture、Infosys、Emicon、Rellins |
|
大於或等於6 |
#rtCamp、Cybertech、Skybags、Killer、Raymond |
|
#大於或等於5 |
#Patronics、鞋子、NoBrokers |
#讓我們進入 java 程式來檢查 tpp 學生參加面試的資格。
方法1:使用if else if條件
通常,當我們必須檢查多個條件時,我們會使用 if else if 語句。它遵循自上而下的方法。
文法
if(condition 1) { // code will be executed only when condition 1 is true } else if(condition 2) { // code will be executed only when condition 2 is true } else { // code will be executed when all of the above condition is false }
範例
public class Eligible { public static void main(String[] args) { int regd = 12109659; double CGPA = 8.08; if( CGPA >= 8 ) { System.out.println(regd + " is eligible for companies: Google, Microsoft, Amazon, Capgemini, Dell, Intel, Wipro"); } else if(CGPA >= 7) { System.out.println(regd + " is eligible for companies: Tutorials point, accenture, Infosys, Emicon, Rellins"); } else if(CGPA >= 6) { System.out.println(regd + " is eligible for companies: rtCamp, Cybertech, Skybags, Killer, Raymond"); } else if( CGPA >= 5 ) { System.out.println(regd + " is eligible for companies: Patronics, Bata, Nobroker"); } else { System.out.println("Improve yourself!"); } } }
輸出
12109659 is eligible for companies: Google, Microsoft, Amazon, Capgemini, Dell, Intel, Wiproe
在上面的程式碼中,我們宣告並初始化了兩個名為「regd」和「CGPA」的變數。當我們執行此程式碼時,編譯器將檢查第一個 if 條件,並且對於給定的「CGPA」值,它是 true。因此,它執行了第一個 if 區塊內的程式碼。
使用Switch語句的方法
switch 語句僅適用於 int、short、byte 和 char 資料型別。它不支援十進制值。它首先評估表達式,如果有任何情況匹配,它將執行該程式碼區塊。如果沒有任何情況匹配,則執行預設情況。
文法
// expression and value must be of same datatype switch(expression) { case value: // code will be executed only when the expression and case value matched break; case value: // code will be executed only when the expression and case value matched break; . . . case value n: // n is required number of value default: // If none of the case matched then it will be executed }
範例
public class Main { public static void main(String[] args){ int regd = 12109659; double CGPA = 6.55; int GPA = (int) CGPA; // typecasting double to integer type switch(GPA){ // here GPA = 6 case 10: case 9: case 8: System.out.println(regd + " is eligible for companies: Google, Microsoft, Amazon, Capgemini, Dell, Intel, Wipro"); break; case 7: System.out.println(regd + " is eligible for companies: Tutorials point, accenture, Infosys, Emicon, Rellins"); break; case 6: System.out.println(regd + " is eligible for companies: rtCamp, Cybertech, Skybags, Killer, Raymond"); break; case 5: System.out.println(regd + " is eligible for companies: Patronics, Bata, Nobroker"); break; default: System.out.println("Improve yourself!"); } } }
輸出
12109659 is eligible for companies: rtCamp, Cybertech, Skybags, Killer, Raymond
在上面的程式碼中,我們再次採用了相同的變數。由於 switch 與 double 變數不相容,我們將其類型轉換為名為「GPA」的整數類型變數。對於給定的“GPA”值,情況 6 與表達式相符。因此,編譯器執行了 case 6 程式碼。
方法三:使用使用者自訂方法
方法是可以多次重複使用以執行單一操作的程式碼區塊。它節省了我們的時間,也減少了程式碼的大小。
文法
accessSpecifier nonAccessModifier return_Type method_Name(Parameters){ //Body of the method }
accessSpecifier - 用於設定方法的可存取性。它可以是公共的、受保護的、預設的和私有的。
nonAccessModifier - 它顯示方法的附加功能或行為,例如靜態和最終。
return_Type − 方法將傳回的資料類型。當方法不回傳任何內容時,我們使用void關鍵字。
method_Name - 方法的名稱。
參數 - 它包含變數名稱,後面跟著資料類型。
範例
public class Main { public static void eligible(int regd, double CGPA){ if(CGPA >= 8){ System.out.println(regd + " is eligible for companies: Google, Microsoft, Amazon, Capgemini, Dell, Intel, Wipro"); } else if(CGPA >= 7){ System.out.println(regd + " is eligible for companies: Tutorials point, accenture, Infosys, Emicon, Rellins"); } else if(CGPA >= 6){ System.out.println(regd + " is eligible for companies: rtCamp, Cybertech, Skybags, Killer, Raymond"); } else if(CGPA >= 5){ System.out.println(regd + " is eligible for companies: Patronics, Bata, Nobroker"); } else { System.out.println("Improve yourself!"); } } public static void main(String[] args){ eligible(12109659, 7.89); } }
輸出
12109659 is eligible for companies: Tutorials point, accenture, Infosys, Emicon, Rellins
上述程序的邏輯與我們在本文中討論的第一個程序相同。主要區別在於我們創建了一個名為“eligible()”的使用者定義方法,帶有兩個參數“regd”和“CGPA”,並且我們在主方法中使用兩個參數呼叫了該方法。
結論
在本文中,我們討論了三種用於檢查tpp學生是否有資格參加面試的java程式方法。我們看到了使用if else if條件和switch語句的用法。我們還為給定的問題創建了一個用戶定義的方法。
以上是Java程式用於檢查TPP學生是否有資格參加面試的詳細內容。更多資訊請關注PHP中文網其他相關文章!
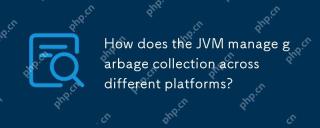
JVMmanagesgarbagecollectionacrossplatformseffectivelybyusingagenerationalapproachandadaptingtoOSandhardwaredifferences.ItemploysvariouscollectorslikeSerial,Parallel,CMS,andG1,eachsuitedfordifferentscenarios.Performancecanbetunedwithflagslike-XX:NewRa
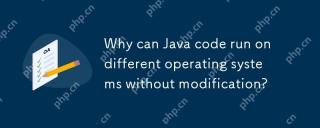
Java代碼可以在不同操作系統上無需修改即可運行,這是因為Java的“一次編寫,到處運行”哲學,由Java虛擬機(JVM)實現。 JVM作為編譯後的Java字節碼與操作系統之間的中介,將字節碼翻譯成特定機器指令,確保程序在任何安裝了JVM的平台上都能獨立運行。
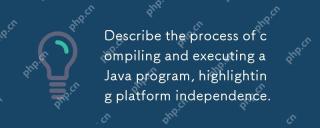
Java程序的編譯和執行通過字節碼和JVM實現平台獨立性。 1)編寫Java源碼並編譯成字節碼。 2)使用JVM在任何平台上執行字節碼,確保代碼的跨平台運行。
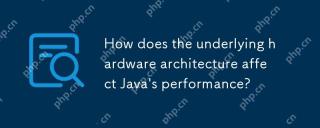
Java性能与硬件架构密切相关,理解这种关系可以显著提升编程能力。1)JVM通过JIT编译将Java字节码转换为机器指令,受CPU架构影响。2)内存管理和垃圾回收受RAM和内存总线速度影响。3)缓存和分支预测优化Java代码执行。4)多线程和并行处理在多核系统上提升性能。
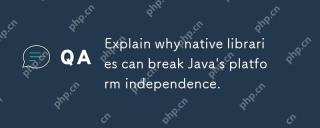
使用原生庫會破壞Java的平台獨立性,因為這些庫需要為每個操作系統單獨編譯。 1)原生庫通過JNI與Java交互,提供Java無法直接實現的功能。 2)使用原生庫增加了項目複雜性,需要為不同平台管理庫文件。 3)雖然原生庫能提高性能,但應謹慎使用並進行跨平台測試。
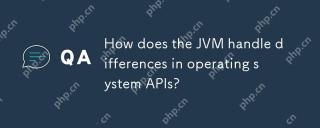
JVM通過JavaNativeInterface(JNI)和Java標準庫處理操作系統API差異:1.JNI允許Java代碼調用本地代碼,直接與操作系統API交互。 2.Java標準庫提供統一API,內部映射到不同操作系統API,確保代碼跨平台運行。
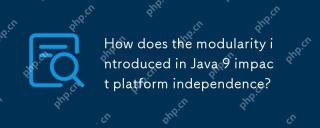
modularitydoesnotdirectlyaffectJava'splatformindependence.Java'splatformindependenceismaintainedbytheJVM,butmodularityinfluencesapplicationstructureandmanagement,indirectlyimpactingplatformindependence.1)Deploymentanddistributionbecomemoreefficientwi
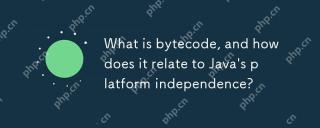
BytecodeinJavaistheintermediaterepresentationthatenablesplatformindependence.1)Javacodeiscompiledintobytecodestoredin.classfiles.2)TheJVMinterpretsorcompilesthisbytecodeintomachinecodeatruntime,allowingthesamebytecodetorunonanydevicewithaJVM,thusfulf


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

Atom編輯器mac版下載
最受歡迎的的開源編輯器
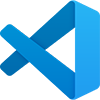
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

記事本++7.3.1
好用且免費的程式碼編輯器
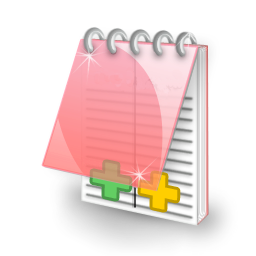
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能