在C 中,trie是一種高階資料結構,用於儲存樹的集合。單字trie來自檢索一詞,它被稱為數字樹或前綴樹。
讓我們透過給定的字串清單來舉出一個所有可能的聯結的例子。
我們將字串輸入定義為 {“tutor”, “true”, “tuo”}
我們可以觀察到不同的字串與單一字串相連。所以這裡的t和u是連接所有可能字串的字元列表。
在本文中,我們將使用trie資料結構來解決一個字串清單中所有可能的連接。
文法
struct name_of_structure{ data_type var_name; // data member or field of the structure. }
參數
struct − 這個關鍵字用來表示結構資料型態。
name_of_structure − 我們為結構提供任何名稱。
結構是將各種相關變數集中在一個地方的集合。
treetrie* alpha[alphabet]
alpha是指向名為treetrie的結構指標/資料成員的變數的名稱。 alphabet是設定字元總數值的宏,以整數形式表示。
演算法
我們首先使用一個名為‘bits/stdc .h’的頭文件,該文件包含了C 的所有標準模板庫。
我們正在定義兩個宏,分別是'alphabet'和'max',它們定義了字母表中的總字元數和字元的最大值。
我們正在建立一個名為‘tree node’的結構,並定義一個指向‘tree_node’的指標來儲存字母的位址。使用bool資料類型定義變數‘end_word’,該變數將用於字串的結束字元。
我們正在使用一個指標來連接表示trie建構的樹的新節點,定義一個名為‘buildNode’的函數。
為了插入字串,我們創建了一個名為'ins_recursive_of_string'的遞歸函數,它接受三個參數- itm,str(要插入的字串),i(表示正在處理的目前字元)。
函數ins()在程式碼中被定義為ins_recursive_of_str()的包裝函數。它接受兩個參數:tree_trie* itm(一個tree_trie物件)和string str(要插入的字串)。它使用當前節點、要插入的字串和起始索引0來呼叫遞歸函數。
接下來,我們正在建立一個名為 LeafNode() 的函數,它接受一個 tree_trie 物件作為參數,並檢查它是否是葉節點,即它是否沒有子節點。
函數display_joint() 在程式碼中定義,並接受四個參數:tree_trie* root, tree_trie* itm(目前正在處理的節點),char str[](一個字元陣列str,用於儲存從根節點到目前節點形成的路徑字串),以及一個int level(表示目前節點深度的整數層級)。
程式碼定義了displayJ()函數,它是display_joint()的包裝函數。它接受一個tree_trie物件作為參數,並使用根節點、一個空字元陣列和起始層級為0作為參數呼叫display_joint()函數。
該程式碼定義了main()函數,它產生一個新的tree_trie物件作為Trie根節點。它產生一個包含要插入到Trie中的字串列表的向量s。然後,它會呼叫ins()函數將每個字串插入到Trie中。
最後,它會列印一條訊息來指示輸出的開始,並呼叫 displayJ() 函數來顯示所有的 Trie 連接點。
範例
在這個程式中,我們將列印由給定字串清單建構的trie的所有可能連接點。
#include <bits/stdc++.h> using namespace std; #define alphabet 26 #define max 200 // creating a structure for trie node struct tree_trie { tree_trie* alpha[alphabet]; bool end_word; }; tree_trie* buildNode(){ tree_trie* temp = new tree_trie(); temp->end_word = false; for (int i = 0; i < alphabet; i++) { temp->alpha[i] = NULL; } return temp; } // We will insert the string using trie recursively void ins_recursive_of_str(tree_trie* itm, string str, int i){ if (i < str.length()) { int idx = str[i] - 'a'; if (itm->alpha[idx] == NULL) { // We are creating a new node itm->alpha[idx] = buildNode(); } // calling recursion function for inserting a string ins_recursive_of_str(itm->alpha[idx], str, i + 1); } else { // We make the end_word true which represents the end of string itm->end_word = true; } } // By using function call we are inserting a tree void ins(tree_trie* itm, string str){ // The necessary argument required for function call ins_recursive_of_str(itm, str, 0); } // Using function we check whether the node is a leaf or not bool isLeafNode(tree_trie* root){ return root->end_word != false; } // This function is an important part of the program to display the joints of trie void display_joint(tree_trie* root, tree_trie* itm, char str[], int level){ //Using this variable we are counting the current child int current_alpha = 0; for (int i = 0; i < alphabet; i++){ if (itm->alpha[i]) { str[level] = i + 'a'; display_joint(root, itm->alpha[i], str, level + 1); current_alpha++; } } // We are printing the character if it has more than 1 character if (current_alpha > 1 && itm != root) { cout << str[level - 1] << endl; } } // By using this function call we are diplaying the joint of trie. void displayJ(tree_trie* root){ int level = 0; char str[max]; display_joint(root, root, str, level); } // main function int main(){ tree_trie* root = buildNode(); vector<string> s = { "tutor", "true", "tuo"}; for (string str : s) { ins(root, str); } cout<<"All possible joint of trie using the given list of string"<<endl; displayJ(root); return 0; }
輸出
All possible joint of trie using the given list of string u t
結論
我們探討了trie資料結構的概念,其中我們從給定的字串列表中建構了所有可能的trie連接點。我們在輸出中看到,字元u和t透過使用諸如tutor、true和tuo等字串連接了trie的所有可能連接點。因此,透過給出可能的連接點,樹可以減少其節點。
以上是列印由給定字串清單建構的Trie的所有可能節點的詳細內容。更多資訊請關注PHP中文網其他相關文章!
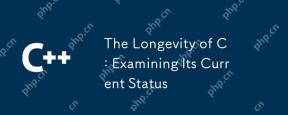
C 在現代編程中依然重要,因其高效、靈活和強大的特性。 1)C 支持面向對象編程,適用於系統編程、遊戲開發和嵌入式系統。 2)多態性是C 的亮點,允許通過基類指針或引用調用派生類方法,增強代碼的靈活性和可擴展性。
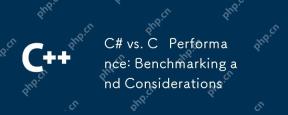
C#和C 在性能上的差異主要體現在執行速度和資源管理上:1)C 在數值計算和字符串操作上通常表現更好,因為它更接近硬件,沒有垃圾回收等額外開銷;2)C#在多線程編程上更為簡潔,但性能略遜於C ;3)選擇哪種語言應根據項目需求和團隊技術棧決定。
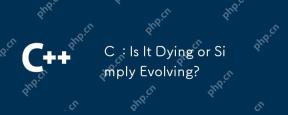
1)c relevantduetoItsAverity and效率和效果臨界。 2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
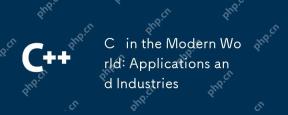
C 在現代世界中的應用廣泛且重要。 1)在遊戲開發中,C 因其高性能和多態性被廣泛使用,如UnrealEngine和Unity。 2)在金融交易系統中,C 的低延遲和高吞吐量使其成為首選,適用於高頻交易和實時數據分析。
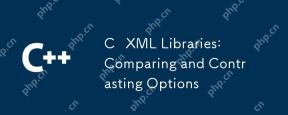
C 中有四種常用的XML庫:TinyXML-2、PugiXML、Xerces-C 和RapidXML。 1.TinyXML-2適合資源有限的環境,輕量但功能有限。 2.PugiXML快速且支持XPath查詢,適用於復雜XML結構。 3.Xerces-C 功能強大,支持DOM和SAX解析,適用於復雜處理。 4.RapidXML專注於性能,解析速度極快,但不支持XPath查詢。
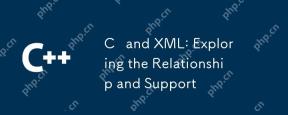
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
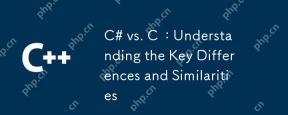
C#和C 的主要區別在於語法、性能和應用場景。 1)C#語法更簡潔,支持垃圾回收,適用於.NET框架開發。 2)C 性能更高,需手動管理內存,常用於系統編程和遊戲開發。
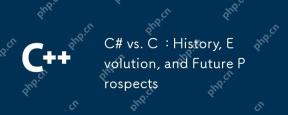
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

Atom編輯器mac版下載
最受歡迎的的開源編輯器
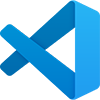
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

SublimeText3 Linux新版
SublimeText3 Linux最新版

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中