The challenge of finding the largest number not exceeding a given number N and not containing any of the digits in a string S is a problem that involves string manipulation and number theory. The goal isblem that involves string manipulation and number theory. The goal is to determine the greatest possible number that is less than or equal to N while also excluding all of the digits found in the string S.
例如,考慮一個情景,其中N等於1000,S等於"42"。在這種情況下,最大的不超過N且不包含S中任何數字的數是999。這是因為999是使用數字0、1、3、5、6、7、8和9組成的最大可能數,而不包括字串S中的數字4和2。
Different approaches can be used to solve this problem, such as iterating through all numbers up to N and verifying if their digits are not present in S, or by utilizing more complex method
Algorithm步驟 1 − 我們將在main()函數中宣告兩個名為‘N’和‘S’的字串變數。
第二步 - 我們將這兩個變數當作參數傳遞給LargestNumberFinder()函數。
Step 3 − We will convert the string number N and S into integer implicitly to do mathematical operations such as comparision.
步驟 4 - 我們將手動刪除儲存在 N 中的數字中的前導 0,或透過建立一個每次都會執行相同操作的函數來刪除它們。
Step 5 − Then, we will start comparing the digits of the both the strings and finding out which is the largest number formed not more than 'N' that doesn't contain any digit from string ' S'.
Approach 1: - Naïve Approach使用另一個字串中的所有數字來尋找給定字串中最大的數字的基本方法如下。主函數宣告變數並呼叫LargestNumberFinder函數。函數以兩個字串作為輸入,檢查每個小於N的值,該值在字串S中具有所有的數字。如果滿足條件,則以字串格式傳回該值。 attendance函數用於確定儲存在'i'中的值是否為字串S的一部分,同時將S轉換為整數資料類型。輸入字串被轉換為整數,並使用循環來評估條件。程式碼輸出在給定字串中具有所有數字的最大數值,該數值在另一個字串中也存在。
Example
的翻譯為:
範例該程式碼是一個解決方案,它找到比N(輸入字串轉換為整數)小的最大數字,該數字由字串S中的數字組成。該程式碼利用兩個函數,'attendance'和'LargestNumberFinder'來確定並返回最大數字。 attendance函數以整數'i'和字串's'作為輸入,檢查儲存在'i'中的值是否為字串's'的一部分,並將's'轉換為整數資料型別。 LargestNumberFinder函數以兩個字串'x'和's'作為輸入,將'x'轉換為整數,然後使用attendance函數檢查所有小於N且所有數字都在's'中的值。主函數宣告變數並呼叫LargestNumberFinder函數,該函數將最大數字作為字串傳回。
#include <iostream> #include <string> #include <vector> // function to check whether value stored in ‘i’ is part of string S while also converting S into integer data type. bool attendance(int i, std::string s) { while (i) { int first_digit = i % 10; i /= 10; int t = std::stoi(s); bool found = false; while (t) { int second_digit = t % 10; t /= 10; if (second_digit == first_digit) { found = true; break; } } if (!found) return false; } return true; } // function to input two strings and check for each value less than N with all digits present in S. std::string LargestNumberFinder(std::string x, std::string s) { int N = std::stoi(x); for (int i = N; i >= 1; i--) { if (attendance(i, s)) { return std::to_string(i); } } return "-1"; } // main function to declare the variables and call the function. int main() { std::string N = "100709"; std::string S = "70"; std::cout << LargestNumberFinder(N, S); }Output
#
77777
方法2:高效方法對於問題2的解決方案,即透過將給定數字字串N的數字替換為給定字串S的數字,得到最大可能的數字,這是一種高效的方法。此方法首先檢查S中是否存在N的每個數字,並以S中不在N中的最大數字取代第一個在S中找到的數字。然後,其餘的數字將被替換為S中不在N中的最大數字。然後去掉前導零,並將結果作為最大可能的數字回傳。這種方法比之前的方法更有效率,因為它不需要對字串進行排序。
Example
的翻譯為:
範例The code solves a problem of finding the greatest number that can be formed from a given string "N" by replacing a digit with the highest digit not present in the string "S". The code utilizes highest digit not present in the string "S"。 problem. The LargestNumberFinder function takes two string inputs, "num" and "s", and returns the largest possible number. The vector "vis_s" is utilized to store the values of string "s". The code first identifies the first diifies the first git string "num" that is part of string "s". Then it swaps that digit with the highest digit not present in string "s". The code then finds the highest digit not found in string "s" and replaces the rest of theplaces the rest of the digits in string "num" with that digit. The leading zeros are removed from the final string, and if the string is empty, the function returns "0". The code outputs the result by calling the function with inputs "N" and " S".
#include <iostream> #include <string> #include <vector> using namespace std; // function to check for all values of String N with String S and replacing the digit if found same with the largest possible digit not present in S. string LargestNumberFinder(string num, string s) { vector<bool> vis_s(10, false); for (int i = 0; i < (int)s.size(); i++) { vis_s[int(s[i]) - 48] = true; } int n = num.size(); int in = -1; for (int i = 0; i < n; i++) { if (vis_s[(int)num[i] - '0']) { in = i; break; } } if (in == -1) { return num; } for (char dig = num[in]; dig >= '0'; dig--) { if (vis_s[(int)dig - '0'] == 0) { num[in] = dig; break; } } char LargestDig = '0'; for (char dig = '9'; dig >= '0'; dig--) { if (vis_s[dig - '0'] == false) { LargestDig = dig; break; } } for (int i = in + 1; i < n; i++) { num[i] = LargestDig; } int Count = 0; for (int i = 0; i < n; i++) { if (num[i] == '0') Count++; else break; } num.erase(0, Count); if ((int)num.size() == 0) return "0"; return num; } int main() { string N = "161516"; string S = "756"; cout << LargestNumberFinder(N, S); return 0; }
Output
149999
结论
通过这篇文章,我们更接近理解这些问题背后的原因,并理解了这些概念,这些概念将帮助我们在之前提到的重大实际问题中使用这些基本概念。就像在我们的代码中,我们分别解决每个问题,然后像制作美丽的手工品一样将代码缝合在一起,同样,我们将使用这个概念,尝试逐个解决问题。我们通常会从朴素的方法开始,但通过敏锐的眼光和努力,我们会找到更高效的方法。谁知道在阅读完这篇文章后,你会找到更好、更高效的方法,并进一步简化解决方案。所以,让我们坚持我们的信念和对思维和编码的信任,同时告别。
以上是不超過N且不包含S中任何數字的最大數字的詳細內容。更多資訊請關注PHP中文網其他相關文章!
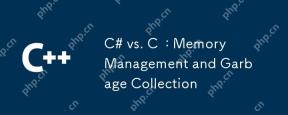
C#使用自動垃圾回收機制,而C 採用手動內存管理。 1.C#的垃圾回收器自動管理內存,減少內存洩漏風險,但可能導致性能下降。 2.C 提供靈活的內存控制,適合需要精細管理的應用,但需謹慎處理以避免內存洩漏。

C 在現代編程中仍然具有重要相關性。 1)高性能和硬件直接操作能力使其在遊戲開發、嵌入式系統和高性能計算等領域佔據首選地位。 2)豐富的編程範式和現代特性如智能指針和模板編程增強了其靈活性和效率,儘管學習曲線陡峭,但其強大功能使其在今天的編程生態中依然重要。
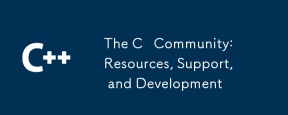
C 學習者和開發者可以從StackOverflow、Reddit的r/cpp社區、Coursera和edX的課程、GitHub上的開源項目、專業諮詢服務以及CppCon等會議中獲得資源和支持。 1.StackOverflow提供技術問題的解答;2.Reddit的r/cpp社區分享最新資訊;3.Coursera和edX提供正式的C 課程;4.GitHub上的開源項目如LLVM和Boost提陞技能;5.專業諮詢服務如JetBrains和Perforce提供技術支持;6.CppCon等會議有助於職業
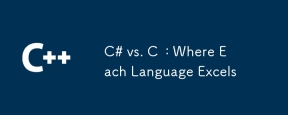
C#適合需要高開發效率和跨平台支持的項目,而C 適用於需要高性能和底層控制的應用。 1)C#簡化開發,提供垃圾回收和豐富類庫,適合企業級應用。 2)C 允許直接內存操作,適用於遊戲開發和高性能計算。
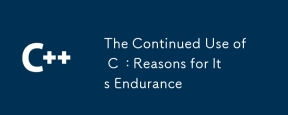
C 持續使用的理由包括其高性能、廣泛應用和不斷演進的特性。 1)高效性能:通過直接操作內存和硬件,C 在系統編程和高性能計算中表現出色。 2)廣泛應用:在遊戲開發、嵌入式系統等領域大放異彩。 3)不斷演進:自1983年發布以來,C 持續增加新特性,保持其競爭力。
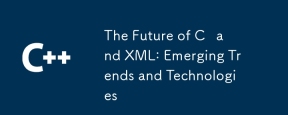
C 和XML的未來發展趨勢分別為:1)C 將通過C 20和C 23標準引入模塊、概念和協程等新特性,提升編程效率和安全性;2)XML將繼續在數據交換和配置文件中佔據重要地位,但會面臨JSON和YAML的挑戰,並朝著更簡潔和易解析的方向發展,如XMLSchema1.1和XPath3.1的改進。
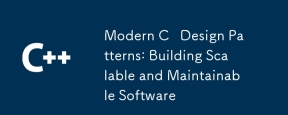
現代C 設計模式利用C 11及以後的新特性實現,幫助構建更靈活、高效的軟件。 1)使用lambda表達式和std::function簡化觀察者模式。 2)通過移動語義和完美轉發優化性能。 3)智能指針確保類型安全和資源管理。
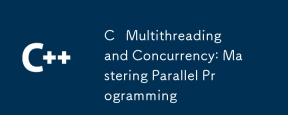
C 多線程和並發編程的核心概念包括線程的創建與管理、同步與互斥、條件變量、線程池、異步編程、常見錯誤與調試技巧以及性能優化與最佳實踐。 1)創建線程使用std::thread類,示例展示瞭如何創建並等待線程完成。 2)同步與互斥使用std::mutex和std::lock_guard保護共享資源,避免數據競爭。 3)條件變量通過std::condition_variable實現線程間的通信和同步。 4)線程池示例展示瞭如何使用ThreadPool類並行處理任務,提高效率。 5)異步編程使用std::as


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。

Atom編輯器mac版下載
最受歡迎的的開源編輯器

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。