在C#中,LINQ(Language Integrated Query)是一個強大的工具,可以輕鬆地對資料進行排序、過濾和操作。在本文中,我們將示範如何使用LINQ根據員工的薪水和部門對員工名單進行排序。
使用LINQ根據薪資和部門對員工清單進行排序
To sort a list of employees based on their salary and department using LINQ, you can follow the steps below −
1. Create a Class to Represent an Employee
public class Employee { public string Name { get; set; } public int Salary { get; set; } public string Department { get; set; } }
2. Create a List of Employees
List<employee> employees = new List { new Employee { Name = "John", Salary = 50000, Department = "ABC" }, new Employee { Name = "Mary", Salary = 60000, Department = "DEF" }, new Employee { Name = "Bob", Salary = 40000, Department = "ABC" }, new Employee { Name = "Alice", Salary = 70000, Department = "XYZ" } };
3. Use LINQ to Sort the List of Employees by Salary and Department
var sortedEmployees = employees .Where(e => e.Department == "ABC") .OrderByDescending(e => e.Salary) .ThenBy(e => e.Name);
4. Iterate through the Sorted List and Print out each Employee's Name and Salary
foreach (var employee in sortedEmployees) { Console.WriteLine($"{employee.Name}: {employee.Salary}"); }
Explanation
的中文翻譯為:解釋
Step 1 - 我們定義一個名為Employee的類別來表示一個員工。這個類別有三個屬性:Name(姓名),Salary(薪水)和Department(部門)。
Step 2 - We create a list of employees and initialize it with some sample data.
步驟 3 - 我們使用 LINQ 將員工清單依照薪水和部門排序。我們先篩選出部門為「ABC」的員工,然後依照薪水降序和姓名升序對篩選後的清單進行排序。結果就是一個滿足篩選條件的員工排序清單。
第四步 - 我們遍歷已排序的員工列表,並使用字串插值列印每個員工的姓名和薪水。
範例
using System; using System.Collections.Generic; using System.Linq; public class Employee { public string Name { get; set; } public int Salary { get; set; } public string Department { get; set; } } class Program { static void Main(string[] args) { List<Employee> employees = new List <Employee>{ new Employee { Name = "John", Salary = 50000, Department = "ABC" }, new Employee { Name = "Mary", Salary = 60000, Department = "DEF" }, new Employee { Name = "Bob", Salary = 40000, Department = "ABC" }, new Employee { Name = "Alice", Salary = 70000, Department = "XYZ" } }; var sortedEmployees = employees .Where(e => e.Department == "ABC") .OrderByDescending(e => e.Salary) .ThenBy(e => e.Name); foreach (var employee in sortedEmployees) { Console.WriteLine($"{employee.Name}: {employee.Salary}"); } } }
輸出
John: 50000 Bob: 40000
Conclusion
使用LINQ根據薪資和部門對員工清單進行排序是C#中操作資料的一種簡單且有效率的方式。透過使用LINQ,您可以只使用幾行程式碼就可以輕鬆地過濾、排序和操作大量的資料。我們希望本文能幫助您理解如何使用LINQ根據薪資和部門對員工名單進行排序。
以上是C# 程式使用 LINQ 根據薪水對部門為 ABC 的員工清單進行排序的詳細內容。更多資訊請關注PHP中文網其他相關文章!

如何將C#.NET應用部署到Azure或AWS?答案是使用AzureAppService和AWSElasticBeanstalk。 1.在Azure上,使用AzureAppService和AzurePipelines自動化部署。 2.在AWS上,使用AmazonElasticBeanstalk和AWSLambda實現部署和無服務器計算。
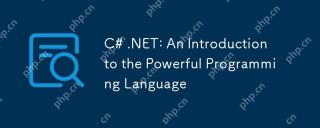
C#和.NET的結合為開發者提供了強大的編程環境。 1)C#支持多態性和異步編程,2).NET提供跨平台能力和並發處理機制,這使得它們在桌面、Web和移動應用開發中廣泛應用。
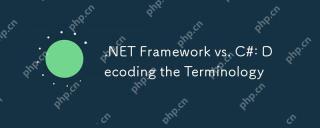
.NETFramework是一個軟件框架,C#是一種編程語言。 1..NETFramework提供庫和服務,支持桌面、Web和移動應用開發。 2.C#設計用於.NETFramework,支持現代編程功能。 3..NETFramework通過CLR管理代碼執行,C#代碼編譯成IL後由CLR運行。 4.使用.NETFramework可快速開發應用,C#提供如LINQ的高級功能。 5.常見錯誤包括類型轉換和異步編程死鎖,調試需用VisualStudio工具。
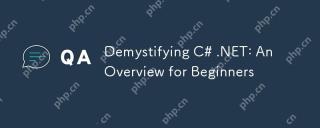
C#是一種由微軟開發的現代、面向對象的編程語言,.NET是微軟提供的開發框架。 C#結合了C 的性能和Java的簡潔性,適用於構建各種應用程序。 .NET框架支持多種語言,提供垃圾回收機制,簡化內存管理。
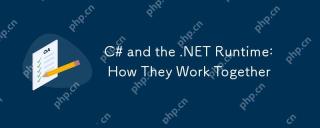
C#和.NET運行時緊密合作,賦予開發者高效、強大且跨平台的開發能力。 1)C#是一種類型安全且面向對象的編程語言,旨在與.NET框架無縫集成。 2).NET運行時管理C#代碼的執行,提供垃圾回收、類型安全等服務,確保高效和跨平台運行。
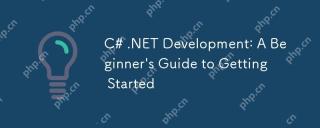
要開始C#.NET開發,你需要:1.了解C#的基礎知識和.NET框架的核心概念;2.掌握變量、數據類型、控制結構、函數和類的基本概念;3.學習C#的高級特性,如LINQ和異步編程;4.熟悉常見錯誤的調試技巧和性能優化方法。通過這些步驟,你可以逐步深入C#.NET的世界,並編寫高效的應用程序。
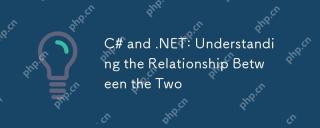
C#和.NET的關係是密不可分的,但它們不是一回事。 C#是一門編程語言,而.NET是一個開發平台。 C#用於編寫代碼,編譯成.NET的中間語言(IL),由.NET運行時(CLR)執行。
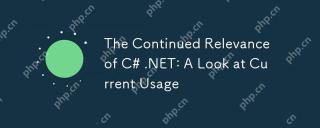
C#.NET依然重要,因為它提供了強大的工具和庫,支持多種應用開發。 1)C#結合.NET框架,使開發高效便捷。 2)C#的類型安全和垃圾回收機制增強了其優勢。 3).NET提供跨平台運行環境和豐富的API,提升了開發靈活性。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SublimeText3漢化版
中文版,非常好用
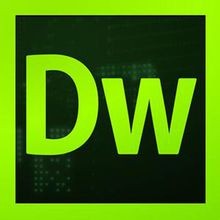
Dreamweaver CS6
視覺化網頁開發工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!