在本文中,我們將描述尋找子陣列中質數數量的方法。我們有一個正數數組 arr[] 和 q 個查詢,其中有兩個整數表示我們的範圍 {l, R},我們需要找到給定範圍內的質數數量。以下是給定問題的範例-
Input : arr[] = {1, 2, 3, 4, 5, 6}, q = 1, L = 0, R = 3 Output : 2 In the given range the primes are {2, 3}. Input : arr[] = {2, 3, 5, 8 ,12, 11}, q = 1, L = 0, R = 5 Output : 4 In the given range the primes are {2, 3, 5, 11}.
尋找解決方案的方法
在這種情況下,我想到了兩種方法-
暴力破解
在這種方法中,我們可以採用範圍並找出該範圍內存在的質數數量。
範例
#include <bits/stdc++.h> using namespace std; bool isPrime(int N){ if (N <= 1) return false; if (N <= 3) return true; if(N % 2 == 0 || N % 3 == 0) return false; for (int i = 5; i * i <= N; i = i + 2){ // as even number can't be prime so we increment i by 2. if (N % i == 0) return false; // if N is divisible by any number then it is not prime. } return true; } int main(){ int N = 6; // size of array. int arr[N] = {1, 2, 3, 4, 5, 6}; int Q = 1; while(Q--){ int L = 0, R = 3; int cnt = 0; for(int i = L; i <= R; i++){ if(isPrime(arr[i])) cnt++; // counter variable. } cout << cnt << "\n"; } return 0; }
輸出
2
但是,這種方法並不是很好,因為這種方法的整體複雜度是O(Q*N* √N),這不是很好。
高效方法
在這種方法中,我們將使用埃拉托斯特尼篩選法建立一個布林數組,告訴我們該元素是否是素數,然後遍歷給定的範圍並找出該數組中素數的總數。布爾數組。
範例
#include <bits/stdc++.h> using namespace std; vector<bool> sieveOfEratosthenes(int *arr, int n, int MAX){ vector<bool> p(n); bool Prime[MAX + 1]; for(int i = 2; i < MAX; i++) Prime[i] = true; Prime[1] = false; for (int p = 2; p * p <= MAX; p++) { // If prime[p] is not changed, then // it is a prime if (Prime[p] == true) { // Update all multiples of p for (int i = p * 2; i <= MAX; i += p) Prime[i] = false; } } for(int i = 0; i < n; i++){ if(Prime[arr[i]]) p[i] = true; else p[i] = false; } return p; } int main(){ int n = 6; int arr[n] = {1, 2, 3, 4, 5, 6}; int MAX = -1; for(int i = 0; i < n; i++){ MAX = max(MAX, arr[i]); } vector<bool> isprime = sieveOfEratosthenes(arr, n, MAX); // boolean array. int q = 1; while(q--){ int L = 0, R = 3; int cnt = 0; // count for(int i = L; i <= R; i++){ if(isprime[i]) cnt++; } cout << cnt << "\n"; } return 0; }
輸出
2
上述程式碼的解釋
這種方法比我們之前應用的蠻力方法要快得多,因為現在的時間複雜度是O(Q*N),也就是比以前的複雜度好得多。
在這種方法中,我們預先計算元素並將它們標記為素數或非素數;因此,這降低了我們的複雜性。除此之外,我們也使用埃拉托斯特尼篩法,這將有助於我們更快找到質數。在此方法中,我們透過使用素數因子標記數字,以 O(N*log(log(N))) 複雜度將所有數字標記為質數或非質數。
結論
在本文中,我們解決了使用埃拉托斯特尼篩選法在 O(Q*N) 中尋找子數組中素數數量的問題。我們也學習了解決這個問題的C 程序以及解決這個問題的完整方法(正常且有效率)。我們可以用其他語言寫相同的程序,例如C、java、python等語言。
以上是使用C++編寫,找出子數組中的質數數的詳細內容。更多資訊請關注PHP中文網其他相關文章!

C 在現代編程中仍然具有重要相關性。 1)高性能和硬件直接操作能力使其在遊戲開發、嵌入式系統和高性能計算等領域佔據首選地位。 2)豐富的編程範式和現代特性如智能指針和模板編程增強了其靈活性和效率,儘管學習曲線陡峭,但其強大功能使其在今天的編程生態中依然重要。
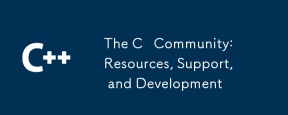
C 學習者和開發者可以從StackOverflow、Reddit的r/cpp社區、Coursera和edX的課程、GitHub上的開源項目、專業諮詢服務以及CppCon等會議中獲得資源和支持。 1.StackOverflow提供技術問題的解答;2.Reddit的r/cpp社區分享最新資訊;3.Coursera和edX提供正式的C 課程;4.GitHub上的開源項目如LLVM和Boost提陞技能;5.專業諮詢服務如JetBrains和Perforce提供技術支持;6.CppCon等會議有助於職業
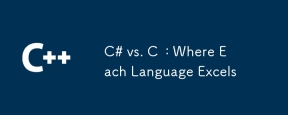
C#適合需要高開發效率和跨平台支持的項目,而C 適用於需要高性能和底層控制的應用。 1)C#簡化開發,提供垃圾回收和豐富類庫,適合企業級應用。 2)C 允許直接內存操作,適用於遊戲開發和高性能計算。
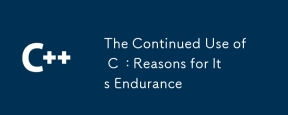
C 持續使用的理由包括其高性能、廣泛應用和不斷演進的特性。 1)高效性能:通過直接操作內存和硬件,C 在系統編程和高性能計算中表現出色。 2)廣泛應用:在遊戲開發、嵌入式系統等領域大放異彩。 3)不斷演進:自1983年發布以來,C 持續增加新特性,保持其競爭力。
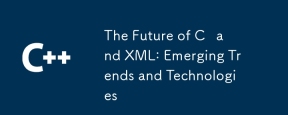
C 和XML的未來發展趨勢分別為:1)C 將通過C 20和C 23標準引入模塊、概念和協程等新特性,提升編程效率和安全性;2)XML將繼續在數據交換和配置文件中佔據重要地位,但會面臨JSON和YAML的挑戰,並朝著更簡潔和易解析的方向發展,如XMLSchema1.1和XPath3.1的改進。
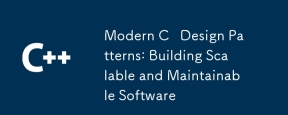
現代C 設計模式利用C 11及以後的新特性實現,幫助構建更靈活、高效的軟件。 1)使用lambda表達式和std::function簡化觀察者模式。 2)通過移動語義和完美轉發優化性能。 3)智能指針確保類型安全和資源管理。
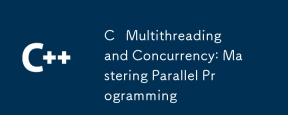
C 多線程和並發編程的核心概念包括線程的創建與管理、同步與互斥、條件變量、線程池、異步編程、常見錯誤與調試技巧以及性能優化與最佳實踐。 1)創建線程使用std::thread類,示例展示瞭如何創建並等待線程完成。 2)同步與互斥使用std::mutex和std::lock_guard保護共享資源,避免數據競爭。 3)條件變量通過std::condition_variable實現線程間的通信和同步。 4)線程池示例展示瞭如何使用ThreadPool類並行處理任務,提高效率。 5)異步編程使用std::as
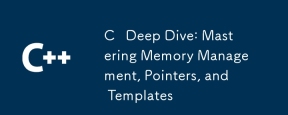
C 的內存管理、指針和模板是核心特性。 1.內存管理通過new和delete手動分配和釋放內存,需注意堆和棧的區別。 2.指針允許直接操作內存地址,使用需謹慎,智能指針可簡化管理。 3.模板實現泛型編程,提高代碼重用性和靈活性,需理解類型推導和特化。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SublimeText3 Linux新版
SublimeText3 Linux最新版
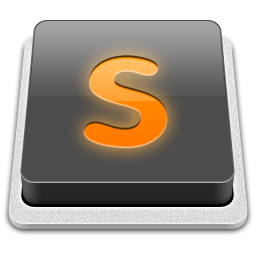
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
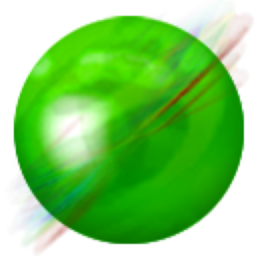
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。
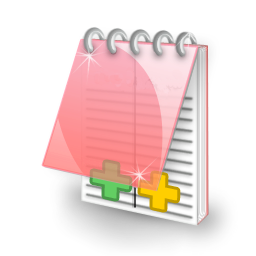
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能