產生所有可能的字串就是將字串中的某個字元替換為對應的符號,產生所有可能的字串。我們將得到一個大小為“N”的字串“s”和一個大小為“M”的字元對的無序映射“mp”。在這裡,我們可以將字串「s」中的 mp[i][0] 替換為 mp[i][1],這樣我們的任務就是產生所有可能的字串。
範例範例
Input: s = “xyZ”, mp = {‘x’ : ‘$’, ‘y’ : ‘#’, ‘Z’ : ‘^’} Output: xyZ xy^ x#Z z#^ $yZ $y^ $#Z $#^
解釋 − 在上面的範例中,總共產生了8個字串。
Input: s = “pQ”, mp = {‘p’ : ‘#’, ‘Q’ : ‘$’} Output: pQ #Q p$ #$
說明 - 在上面的範例中,總共產生了 4 個字串。
Input: s = “w”, mp = {‘w’ : ‘#’} Output: w #
Explanation − 在上面的範例中,總共產生了2個字串。
方法
在這個方法中,我們將使用蠻力的概念來找到所有可能的組合。
首先,我們將建立一個函數,該函數將以字串、當前索引和給定的映射作為參數,並且傳回類型將為void。
在這個函數中,我們將定義基本條件,即當前索引等於字串的大小,然後我們將列印該字串並從函數中返回。
否則,我們將有兩個選擇,一個是不改變當前索引並移動到下一個,這將始終是一個選項。
第二個選擇只有在目前字元有替換時才可能發生。如果替換存在,則我們將呼叫替換。
之後我們將從函數傳回,它將自動產生所有所需的結果。
讓我們討論上述方法的程式碼,以便更好地理解。
範例
#include <bits/stdc++.h> using namespace std; // Function to generate all possible strings by replacing the characters with paired symbols void possibleStrings(string str, int idx, unordered_map<char, char> mp){ if (idx == str.size()) { cout << str << endl; return; } // Function call with the idx-th character not replaced possibleStrings(str, idx + 1, mp); // Replace the idx-th character str[idx] = mp[str[idx]]; // Function call with the idx-th character replaced possibleStrings(str, idx + 1, mp); return; } int main(){ string str = "xyZ"; unordered_map<char, char> mp; mp['x'] = '$'; mp['y'] = '#'; mp['Z'] = '^'; mp['q'] = '&'; mp['2'] = '*'; mp['1'] = '!'; mp['R'] = '@'; int idx = 0; // Call 'possibleStrings' function to generate all possible strings //Here in the 'possible strings' function, we have passed string 'str', index 'idx', and map 'mp' possibleStrings(str, idx, mp); return 0; }
輸出
xyZ xy^ x#Z x#^ $yZ $y^ $#Z $#^
時間與空間複雜度
上述程式碼的時間複雜度為O(N*2^N),因為我們剛剛在N個元素上進行了回溯,其中N是字串's'的大小。
上述程式碼的空間複雜度為O(N*N),因為我們將字串作為完整的傳送,同時可能存在N個字串的副本。
回溯演算法
在先前的方法中,我們發送的字串沒有指針,這導致佔用了很多空間。為了減少空間和時間複雜度,我們將使用回溯的概念。
範例
#include <bits/stdc++.h> using namespace std; // Function to generate all possible strings by replacing the characters with paired symbols void possibleStrings(string& str, int idx, unordered_map<char, char> mp){ if (idx == str.size()) { cout << str << endl; return; } // Function call with the idx-th character not replaced possibleStrings(str, idx + 1, mp); // storing the current element char temp = str[idx]; // Replace the idx-th character str[idx] = mp[str[idx]]; // Function call with the idx-th character replaced possibleStrings(str, idx + 1, mp); // backtracking str[idx] = temp; return; } int main(){ string str = "xyZ"; unordered_map<char, char> mp; mp['x'] = '$'; mp['y'] = '#'; mp['Z'] = '^'; mp['q'] = '&'; mp['2'] = '*'; mp['1'] = '!'; mp['R'] = '@'; int idx = 0; // Call 'possibleStrings' function to generate all possible strings //Here in the 'possible strings' function, we have passed string 'str', index 'idx', and map 'mp' possibleStrings(str, idx, mp); return 0; }
輸出
xyZ xy^ x#Z x#^ $yZ $y^ $#Z $#^
時間與空間複雜度
上述程式碼的時間複雜度為O(N*2^N),因為我們剛剛在N個元素上進行了回溯,其中N是字串's'的大小。
上述程式碼的空間複雜度為O(N),因為我們傳送的是字串的位址,只會最多有N個堆疊向下。
結論
在本教程中,我們已經實作了一個程序,用給定的符號替換字母來產生所有可能的字串。在這裡,我們已經看到了回溯法的方法,並且程式碼的時間複雜度是O(N*2^N),其中N是字串的大小,空間複雜度與時間複雜度相同。為了減少空間複雜度,我們已經實現了回溯過程。
以上是產生由給定的相應符號替換字母而形成的所有可能字串的詳細內容。更多資訊請關注PHP中文網其他相關文章!
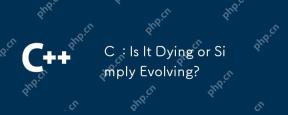
1)c relevantduetoItsAverity and效率和效果臨界。 2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
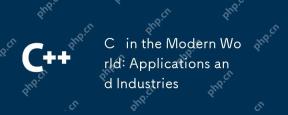
C 在現代世界中的應用廣泛且重要。 1)在遊戲開發中,C 因其高性能和多態性被廣泛使用,如UnrealEngine和Unity。 2)在金融交易系統中,C 的低延遲和高吞吐量使其成為首選,適用於高頻交易和實時數據分析。
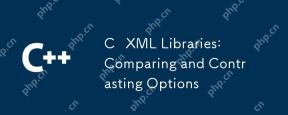
C 中有四種常用的XML庫:TinyXML-2、PugiXML、Xerces-C 和RapidXML。 1.TinyXML-2適合資源有限的環境,輕量但功能有限。 2.PugiXML快速且支持XPath查詢,適用於復雜XML結構。 3.Xerces-C 功能強大,支持DOM和SAX解析,適用於復雜處理。 4.RapidXML專注於性能,解析速度極快,但不支持XPath查詢。
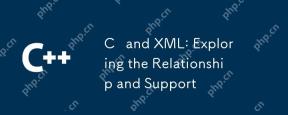
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
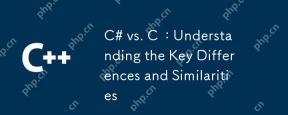
C#和C 的主要區別在於語法、性能和應用場景。 1)C#語法更簡潔,支持垃圾回收,適用於.NET框架開發。 2)C 性能更高,需手動管理內存,常用於系統編程和遊戲開發。
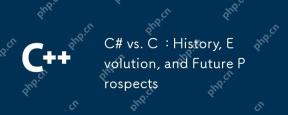
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
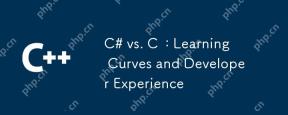
C#和C 的学习曲线和开发者体验有显著差异。1)C#的学习曲线较平缓,适合快速开发和企业级应用。2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
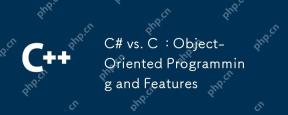
C#和C 在面向对象编程(OOP)中的实现方式和特性上有显著差异。1)C#的类定义和语法更为简洁,支持如LINQ等高级特性。2)C 提供更细粒度的控制,适用于系统编程和高性能需求。两者各有优势,选择应基于具体应用场景。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
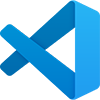
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

Atom編輯器mac版下載
最受歡迎的的開源編輯器
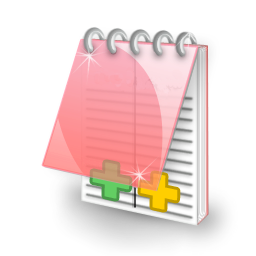
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能
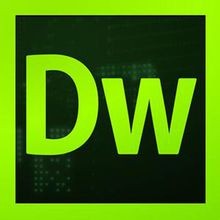
Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!