您是否在任何網站上看到顯示時間戳記的通知?它顯示諸如“12 分鐘前”、“2 天前”、“10 小時前”等內容。它與兩個日期或時間之間的時間戳差異有關。
此外,某些應用程式顯示該裝置的上次登入時間是 22 小時前。因此,取得兩個日期之間的時間戳差異有很多用途。
在本教程中,我們將學習不同的方法來取得兩個日期之間的相對時間戳差異。
使用帶有日期的 getTime() 方法並建立自訂演算法
在 JavaScript 中,我們可以使用 new Date() 建構函數來建立日期物件。此外,我們可以將特定日期作為 Date() 建構函數的參數傳遞,以使用該日期值初始化日期物件。
getTime() 方法傳回 1970 年 1 月 1 日至今的總秒數。因此,我們可以找到兩個日期的總毫秒數,然後減去它們以獲得毫秒差。使用該毫秒,我們可以找到以秒、分鐘、年等為單位的時間戳差。
文法
使用者可以按照下面的語法來取得兩個日期之間的相對時間戳記差異。
let second_diff = (current_date.getTime() - previous_date.getTime())/1000;
在上面的語法中,current_date 和 pervious_date 是兩個不同的日期。我們使用 getTime() 方法來取得兩個日期之間的毫秒差。
注意- 透過將 second_diff 變數的值與毫秒進行比較,您可以獲得相對時間戳記差異。
步驟
使用者可以按照以下步驟尋找不同單位(例如天、月、年等)的兩個日期之間的相對時間戳記。
第 1 步 - 建立兩個不同的日期。
步驟 2 - 使用 getTime() 方法取得兩個日期的總毫秒數並取得它們之間的差異。另外,將毫秒差除以 1000 將其轉換為秒,並將其儲存在 secondary_diff 變數中。
步驟 3 - 現在,使用 if-else 條件語句來找出相對時間戳差異。
步驟 4 - 如果 second_diff 的 值小於 60,則差異以秒為單位。 Second_diff 的值在 60 到 3600 之間,差異以小時為單位。用戶還可以像這樣計算日、月、年。
範例
在下面的範例中,我們使用 Date 建構函數建立了兩個不同的日期對象,並使用上述步驟來尋找兩個日期之間的相對時間戳記。
在輸出中,使用者可以觀察到以下程式碼代表月份的時間戳記差異。
<html> <body> <h3 id="Getting-the-relative-timestamp-difference-between-two-dates-using-the-i-custom-algorithm-i">Getting the relative timestamp difference between two dates using the <i> custom algorithm </i></h3> <p id="output"></p> <script> let output = document.getElementById("output"); // creating the current date let current_date = new Date(); // previous date let previous_date = new Date("jan 14, 2022 12:21:45"); // finding the difference in total seconds between two dates let second_diff = (current_date.getTime() - previous_date.getTime()) / 1000; output.innerHTML += "The first date is " + current_date + "</br>"; output.innerHTML += "The second date is " + previous_date + "</br>"; // showing the relative timestamp. if (second_diff < 60) { output.innerHTML += "difference is of " + second_diff + " seconds."; } else if (second_diff < 3600) { output.innerHTML += "difference is of " + second_diff / 60 + " minutes."; } else if (second_diff < 86400) { output.innerHTML += "difference is of " + second_diff / 3600 + " hours."; } else if (second_diff < 2620800) { output.innerHTML += "difference is of " + second_diff / 86400 + " days."; } else if (second_diff < 31449600) { output.innerHTML += "difference is of " + second_diff / 2620800 + " months."; } else { output.innerHTML += "difference is of " + second_diff / 31449600 + " years."; } </script> </body> </html>
使用Intl的RelativeTimeFormat() API
Intl是指國際化API。它包含各種日期和時間格式化方法。我們可以使用 Intl 物件的 RelativeTimeFormat() 方法來取得兩個日期之間的相對時間戳記。
文法
使用者可以依照下列語法使用RelativeTimeFormat() API 來取得兩個日期之間的相對時間戳記。
var relativeTimeStamp = new Intl.RelativeTimeFormat("en", { numeric: "auto",}); // compare the value of RelativeTimeStamp with milliseconds of different time units
在上述語法中,RelativeTimeFormat() 方法傳回時間戳記差異。 time_Stamp_unit 是一個包含不同時間單位及其總毫秒數的物件。
步驟
第 1 步 - 建立一個單位對象,其中包含時間單位作為鍵,總毫秒數作為該時間單位的值。
步驟 2 - 取得兩個日期之間的時間差(以毫秒為單位)。
第3 步驟 - 現在使用for-in 迴圈迭代time_stamp_unit 物件並檢查second_diff 的值是否大於特定時間的總毫秒數;使用RelativeTimeFormat() API 的format 方法來格式化該特定單位的時間戳記。
第 4 步 - 之後,中斷 for 迴圈。
範例
在下面的範例中,我們使用 RelativeTimeFomrat() 方法來取得兩個日期之間的相對時間戳記差異,如上述語法和步驟所述。
<html> <body> <h3 id="Getting-the-relative-timestamp-difference-between-two-dates-using-the-i-RelativeTimeFormat-i-method">Getting the relative timestamp difference between two dates using the <i> RelativeTimeFormat() </i> method </h3> <p id="output"></p> <script> let output = document.getElementById("output"); let current_date = new Date(); let previous_date = new Date("jan 14, 2022 12:21:45"); // finding the difference in total seconds between two dates let second_diff = current_date.getTime() - previous_date.getTime(); output.innerHTML += "The first date is " + current_date + "</br>"; output.innerHTML += "The second date is " + previous_date + "</br>"; var time_Stamp_unit = { year: 31536000000, month: 31536000000 / 12, day: 86400000, hour: 3600000, minute: 60000, second: 1000, }; var relativeTimeStamp = new Intl.RelativeTimeFormat("en", { numeric: "auto", }); // iterate through all time stamps for (var ele in time_Stamp_unit) { // if second_diff's value is greater than particular timesapm unit's total millisecond value, format accordingly if (Math.abs(second_diff) > time_Stamp_unit[ele] || ele == "second") { output.innerHTML += "The difference between two dates is " + relativeTimeStamp.format( Math.round(second_diff / time_Stamp_unit[ele]), ele ); break; } } </script> </body> </html>
使用者學會了使用 if-else 語句和 RelativeTimeFormat() API 的 format() 方法來找出兩個日期之間的相對時間戳記。使用者可以根據自己的需求使用這兩種方法。
以上是在 JavaScript 中取得日期之間的相對時間戳差異的詳細內容。更多資訊請關注PHP中文網其他相關文章!
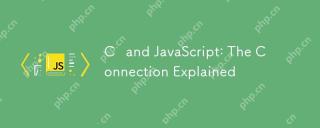
C 和JavaScript通過WebAssembly實現互操作性。 1)C 代碼編譯成WebAssembly模塊,引入到JavaScript環境中,增強計算能力。 2)在遊戲開發中,C 處理物理引擎和圖形渲染,JavaScript負責遊戲邏輯和用戶界面。
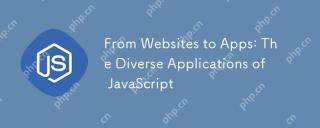
JavaScript在網站、移動應用、桌面應用和服務器端編程中均有廣泛應用。 1)在網站開發中,JavaScript與HTML、CSS一起操作DOM,實現動態效果,並支持如jQuery、React等框架。 2)通過ReactNative和Ionic,JavaScript用於開發跨平台移動應用。 3)Electron框架使JavaScript能構建桌面應用。 4)Node.js讓JavaScript在服務器端運行,支持高並發請求。
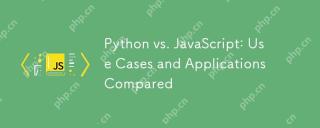
Python更適合數據科學和自動化,JavaScript更適合前端和全棧開發。 1.Python在數據科學和機器學習中表現出色,使用NumPy、Pandas等庫進行數據處理和建模。 2.Python在自動化和腳本編寫方面簡潔高效。 3.JavaScript在前端開發中不可或缺,用於構建動態網頁和單頁面應用。 4.JavaScript通過Node.js在後端開發中發揮作用,支持全棧開發。
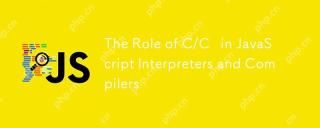
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。1)C 用于解析JavaScript源码并生成抽象语法树。2)C 负责生成和执行字节码。3)C 实现JIT编译器,在运行时优化和编译热点代码,显著提高JavaScript的执行效率。
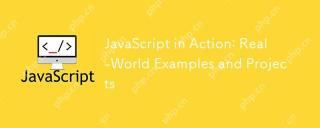
JavaScript在現實世界中的應用包括前端和後端開發。 1)通過構建TODO列表應用展示前端應用,涉及DOM操作和事件處理。 2)通過Node.js和Express構建RESTfulAPI展示後端應用。
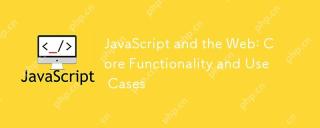
JavaScript在Web開發中的主要用途包括客戶端交互、表單驗證和異步通信。 1)通過DOM操作實現動態內容更新和用戶交互;2)在用戶提交數據前進行客戶端驗證,提高用戶體驗;3)通過AJAX技術實現與服務器的無刷新通信。
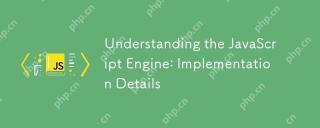
理解JavaScript引擎內部工作原理對開發者重要,因為它能幫助編寫更高效的代碼並理解性能瓶頸和優化策略。 1)引擎的工作流程包括解析、編譯和執行三個階段;2)執行過程中,引擎會進行動態優化,如內聯緩存和隱藏類;3)最佳實踐包括避免全局變量、優化循環、使用const和let,以及避免過度使用閉包。

Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

SublimeText3漢化版
中文版,非常好用

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),