陣列是一種線性資料結構,用於儲存具有相似資料類型的元素組。它以順序方式儲存資料。一旦我們建立了一個數組,我們就無法改變它的大小,也就是它是固定長度的。
本文將幫助您了解陣列和陣列綁定的基本概念。此外,我們還將討論在向數組輸入元素時檢查數組邊界的 java 程式。
陣列和陣列綁定
我們可以透過索引存取陣列元素。假設我們有一個長度為N的數組,那麼
#在上圖中我們可以看到陣列中有 7 個元素,但索引值是從 0 到 6,也就是 0 到 7 - 1。
陣列的範圍稱為它的邊界。上面數組的範圍是從 0 到 6,因此,我們也可以說 0 到 6 是給定數組的界限。如果我們嘗試存取超出範圍的索引值或負索引,我們將得到 ArrayIndexOutOfBoundsException。這是運行時發生的錯誤。
宣告數組的語法
Data_Type[] nameOfarray; // declaration Or, Data_Type nameOfarray[]; // declaration Or, // declaration with size Data_Type nameOfarray[] = new Data_Type[sizeofarray]; // declaration and initialization Data_Type nameOfarray[] = {values separated with comma};
我們可以在我們的程式中使用上述任何語法。
在將元素輸入陣列時檢查陣列邊界
範例 1
如果我們存取數組範圍內的元素,那麼我們不會得到任何錯誤。程序將成功執行。
public class Main { public static void main(String []args) { // declaration and initialization of array ‘item[]’ with size 5 String[] item = new String[5]; // 0 to 4 is the indices item[0] = "Rice"; item[1] = "Milk"; item[2] = "Bread"; item[3] = "Butter"; item[4] = "Peanut"; System.out.print(" Elements of the array item: " ); // loop will iterate till 4 and will print the elements of ‘item[]’ for(int i = 0; i <= 4; i++) { System.out.print(item[i] + " "); } } }
輸出
Elements of the array item: Rice Milk Bread Butter Peanut
範例 2
讓我們嘗試列印給定數組範圍之外的值。
public class Tutorialspoint { public static void main(String []args) { String[] item = new String[5]; item[0] = "Rice"; item[1] = "Milk"; item[2] = "Bread"; item[3] = "Butter"; item[4] = "Peanut"; // trying to run the for loop till index 5 for(int i = 0; i <= 5; i++) { System.out.println(item[i]); } } }
輸出
Rice Milk Bread Butter Peanut Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 5 at Tutorialspoint.main(Tutorialspoint.java:11)
正如我們之前討論的,如果我們嘗試存取陣列的索引值超出其範圍或負索引,我們將得到 ArrayIndexOutOfBoundsException。
在上面的程式中,我們嘗試執行 for 迴圈直到陣列「item[]」的索引 5,但其範圍僅為 0 到 4。因此,在列印元素直到 4 後,我們收到了錯誤。
範例 3
在這個範例中,我們嘗試使用 try 和 catch 區塊來處理 ArrayIndexOutOfBoundsException。我們將在使用者將元素輸入數組時檢查數組邊界。
import java.util.*; public class Tutorialspoint { public static void main(String []args) throws ArrayIndexOutOfBoundsException { // Here ‘sc’ is the object of scanner class Scanner sc = new Scanner(System.in); System.out.print("Enter number of items: "); int n = sc.nextInt(); // declaration and initialization of array ‘item[]’ String[] item = new String[n]; // try block to test the error try { // to take input from user for(int i =0; i<= item.length; i++) { item[i] = sc.nextLine(); } } // We will handle the exception in catch block catch (ArrayIndexOutOfBoundsException exp) { // Printing this message to let user know that array bound exceeded System.out.println( " Array Bounds Exceeded \n Can't take more inputs "); } } }
輸出
Enter number of items: 3
結論
在本文中,我們了解了陣列和陣列綁定。我們已經討論了為什麼如果我們嘗試存取超出其範圍的陣列元素會收到錯誤,以及如何使用 try 和 catch 區塊處理此錯誤。
以上是Java程式在輸入數組元素時檢查數組邊界的詳細內容。更多資訊請關注PHP中文網其他相關文章!
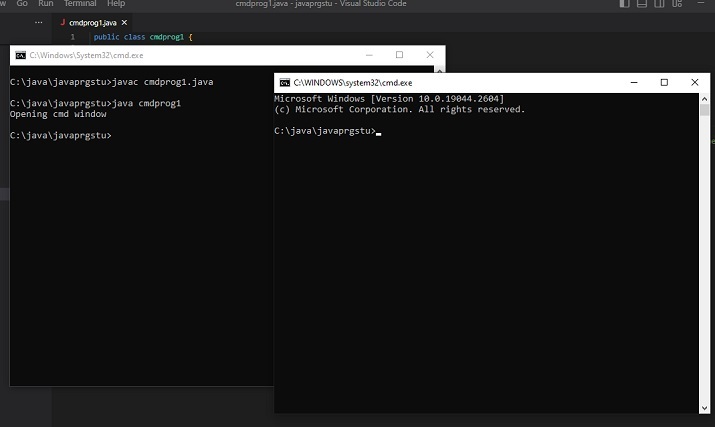
ThisarticleusesvariousapproachesforselectingthecommandsinsertedintheopenedcommandwindowthroughtheJavacode.Thecommandwindowisopenedbyusing‘cmd’.Here,themethodsofdoingthesamearespecifiedusingJavacode.TheCommandwindowisfirstopenedusingtheJavaprogram.Iti
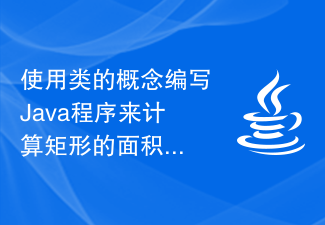
Java语言是当今世界上最常用的面向对象编程语言之一。类的概念是面向对象语言中最重要的特性之一。一个类就像一个对象的蓝图。例如,当我们想要建造一座房子时,我们首先创建一份房子的蓝图,换句话说,我们创建一个显示我们将如何建造房子的计划。根据这个计划,我们可以建造许多房子。同样地,使用类,我们可以创建许多对象。类是创建许多对象的蓝图,其中对象是真实世界的实体,如汽车、自行车、笔等。一个类具有所有对象的特征,而对象具有这些特征的值。在本文中,我们将使用类的概念编写一个Java程序,以找到矩形的周长和面
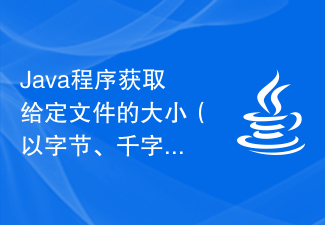
文件的大小是特定文件在特定存储设备(例如硬盘驱动器)上占用的存储空间量。文件的大小以字节为单位来衡量。在本节中,我们将讨论如何实现一个java程序来获取给定文件的大小(以字节、千字节和兆字节为单位)。字节是数字信息的最小单位。一个字节等于八位。1千字节(KB)=1,024字节1兆字节(MB)=1,024KB千兆字节(GB)=1,024MB和1太字节(TB)=1,024GB。文件的大小通常取决于文件的类型及其包含的数据量。以文本文档为例,文件的大小可能只有几千字节,而高分辨率图像或视频文件的大小可
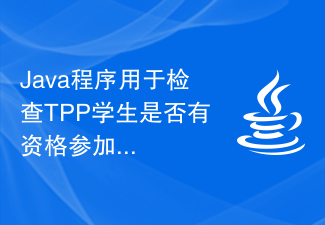
请考虑下表了解不同公司的资格标准-CGPA的中文翻译为:绩点平均成绩符合条件的公司大于或等于8谷歌、微软、亚马逊、戴尔、英特尔、Wipro大于或等于7教程点、accenture、Infosys、Emicon、Rellins大于或等于6rtCamp、Cybertech、Skybags、Killer、Raymond大于或等于5Patronics、鞋子、NoBrokers让我们进入java程序来检查tpp学生参加面试的资格。方法1:使用ifelseif条件通常,当我们必须检查多个条件时,我们会使用
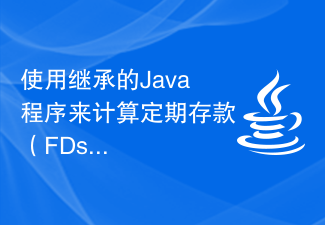
继承是一个概念,它允许我们从一个类访问另一个类的属性和行为。被继承方法和成员变量的类被称为超类或父类,而继承这些方法和成员变量的类被称为子类或子类。在Java中,我们使用“extends”关键字来继承一个类。在本文中,我们将讨论使用继承来计算定期存款和定期存款的利息的Java程序。首先,在您的本地机器IDE中创建这四个Java文件-Acnt.java−这个文件将包含一个抽象类‘Acnt’,用于存储账户详情,如利率和金额。它还将具有一个带有参数‘amnt’的抽象方法‘calcIntrst’,用于计
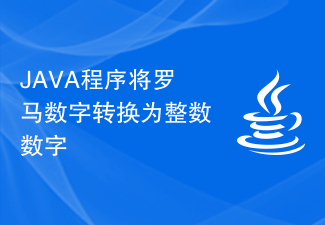
罗马数字-基于古罗马系统,使用符号来表示数字。这些数字称为罗马数字。符号为I、V、X、L、C、D和M,分别代表1、5、10、50、100、500和1,000。整数-整数就是由正值、负值和零值组成的整数。分数不是整数。这里我们根据整数值设置符号值。每当输入罗马数字作为输入时,我们都会将其划分为单位,然后计算适当的罗马数字。I-1II–2III–3IV–4V–5VI–6...X–10XI–11..XV-15在本文中,我们将了解如何在Java中将罗马数字转换为整数。向您展示一些实例-实例1InputR
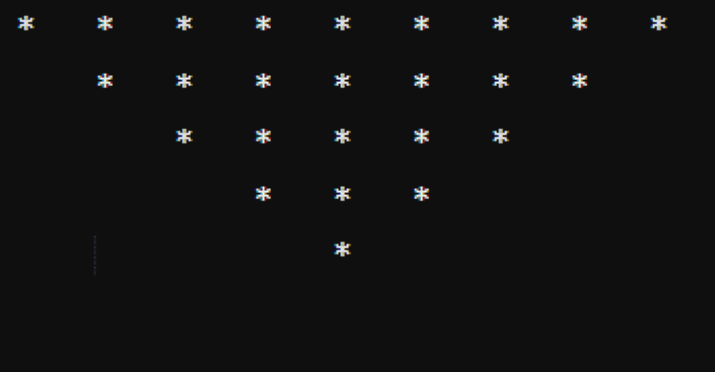
如果有人想在Java编程语言方面打下坚实的基础。然后,有必要了解循环的工作原理。此外,解决金字塔模式问题是增强Java基础知识的最佳方法,因为它包括for和while循环的广泛使用。本文旨在提供一些Java程序,借助Java中可用的不同类型的循环来打印金字塔图案。创建金字塔图案的Java程序我们将通过Java程序打印以下金字塔图案-倒星金字塔星金字塔数字金字塔让我们一一讨论。模式1:倒星金字塔方法声明并初始化一个指定行数的整数“n”。接下来,将空间的初始计数定义为0,将星形的初始计数定义为“n+
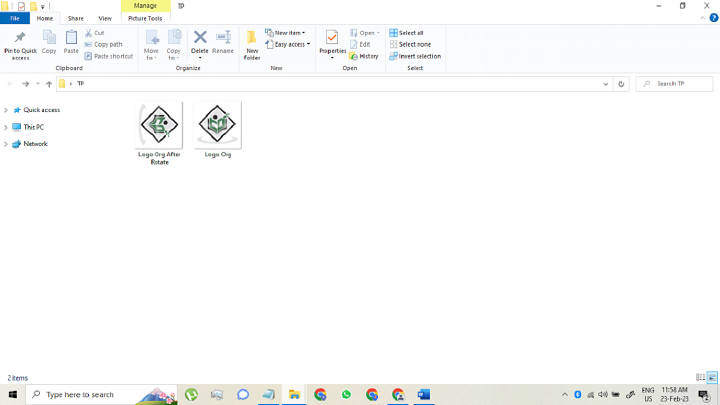
一个图像文件可以顺时针或逆时针旋转。要旋转图像,需要下载一个随机的图像文件并将其保存在系统的任何文件夹中。此外,需要一个.pdf文件,在打开下载的图像文件后,可以在该特定的.pdf文件中旋转一些角度。对于90度的旋转,新图像的锚点可以帮助我们使用Java中的平移变换执行旋转操作。锚点是任何特定图像的中心。AlgorithmtoRotateanImagebyUsingJavaThe"AffineTransformOp"classisthesimplestwaytorotatea


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
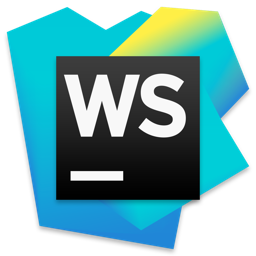
WebStorm Mac版
好用的JavaScript開發工具
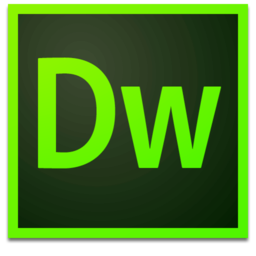
Dreamweaver Mac版
視覺化網頁開發工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。
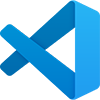
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

記事本++7.3.1
好用且免費的程式碼編輯器