問題陳述
我們給了一個字串 str,總共包含 N 個字。我們需要找到給定字串中的所有回文單詞,並透過反轉所有回文單字的順序來創建一個新字串。
範例
輸入
str = ‘nayan was gone to navjivan eye hospital’
輸出
‘eye was gone to navjivan nayan hospital’
說明
此字串包含三個回文字:nayan、navjivan 和 eye。我們顛倒了所有三個單字的順序,並保持所有其他單字相同。
輸入
‘Hello, users! How are you?’
輸出
‘Hello, users! How are you?’
說明
它提供相同的輸出,因為字串不包含任何回文單字。
輸入
‘Your eye is beautiful.’
輸出
‘Your eye is beautiful.’
說明
它提供與僅包含單一回文單字的字串相同的輸出。
方法 1
在這種方法中,我們首先將字串拆分為單字。之後,我們將過濾所有回文詞。接下來,我們反轉所有回文詞的順序。
最後,我們遍歷字串,如果當前單字是回文單詞,我們將用另一個回文單字以相反的順序替換它。
演算法
步驟 1 - 透過傳遞一個字串作為傳回結果字串的參數來執行reversePlaindromic()函數。
第 2 步 - 建立 isPalindrome() 函數,用於檢查單字是否為回文。
步驟 2.1 - 將「start」初始化為 0,將「end」初始化為字串長度 – 1。
步驟 2.2 - 使用 while 循環遍歷字串,比較第一個和最後一個字符,比較第二個和倒數第二個字符,依此類推。如果任何字元不匹配,則傳回 false,因為它不是回文字串。
步驟 2.3 - 如果字串是回文,則傳回 true。
第 3 步 - 建立一個向量來儲存字串的單字。另外,定義「temp」變數來儲存該單字。
步驟 4 - 使用 for 迴圈遍歷字串,如果不等於空格 (‘ ’),則將字元附加到臨時值。否則,將 temp 的值推送到 allWords 向量。
步驟 5 - 迭代 allWords 向量並使用 isPalindrome() 函數檢查目前單字是否為回文。如果是,則將該單字推入「palindromWords」向量。
第 6 步 - 反轉「palindromWords」清單。
第 7 步 - 現在,再次迭代「allWords」向量,並檢查目前單字是否是回文。如果是,請將其替換為「palindromWords」清單中受尊重的單字。
第 8 步 - 迭代「palindromWords」列表,並透過將所有單字附加到結果變數來建立一個字串。傳回結果字串。
範例
#include <iostream> #include <vector> #include <algorithm> using namespace std; // Function to check if a string is a palindrome bool isPalindrome(string str){ int start = 0; int end = str.length() - 1; // iterate till start < end while (start < end){ // check if the character at the start and end are not the same and return false, else increment start and decrement end if (str[start] != str[end]){ return false; } else { start++; end--; } } return true; } string reversePalindromic(string str) { // vectors to store all words and palindromic words vector<string> palindromWords; vector<string> allWords; // variable to store single word string temp = ""; for (char x : str) { // If the current character is not space, then append it to temp; else, add temp to palindrome words and make temp NULL if (x != ' ') { temp += x; } else { allWords.push_back(temp); temp = ""; } } // push the last word to all words allWords.push_back(temp); // fetch all palindromic words for (string x : allWords){ if (isPalindrome(x)){ // Update newlist palindromWords.push_back(x); } } // Reverse the vector reverse(palindromWords.begin(), palindromWords.end()); int k = 0; for (int i = 0; i < allWords.size(); i++){ // If the current word is a palindrome, push it to palindrome words if (isPalindrome(allWords[i])){ allWords[i] = palindromWords[k]; k++; } } string result = ""; for (string x : allWords) { result += x; result += " "; } return result; } int main(){ string str = "nayan was gone to navjivan eye hospital"; string reverse = reversePalindromic(str); cout << reverse << endl; return 0; }
輸出
eye was gone to navjivan nayan hospital
時間複雜度 - O(N),因為我們迭代長度為 N 的字串。
空間複雜度 - O(K),因為我們使用列表來儲存單字,其中 k 是字串中的單字總數。
結論
我們學會了從句子中獲取所有回文單字並以相反的順序添加它們。在上面的程式碼中,程式設計師可以嘗試更改 isPalindrome() 函數的實作來學習新的東西。
以上是透過顛倒所有回文單字的出現順序來修改句子的詳細內容。更多資訊請關注PHP中文網其他相關文章!
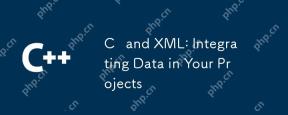
在C 項目中集成XML可以通過以下步驟實現:1)使用pugixml或TinyXML庫解析和生成XML文件,2)選擇DOM或SAX方法進行解析,3)處理嵌套節點和多級屬性,4)使用調試技巧和最佳實踐優化性能。
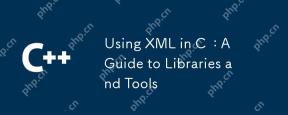
在C 中使用XML是因為它提供了結構化數據的便捷方式,尤其在配置文件、數據存儲和網絡通信中不可或缺。 1)選擇合適的庫,如TinyXML、pugixml、RapidXML,根據項目需求決定。 2)了解XML解析和生成的兩種方式:DOM適合頻繁訪問和修改,SAX適用於大文件或流數據。 3)優化性能時,TinyXML適合小文件,pugixml在內存和速度上表現好,RapidXML處理大文件優異。
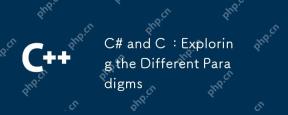
C#和C 的主要區別在於內存管理、多態性實現和性能優化。 1)C#使用垃圾回收器自動管理內存,C 則需要手動管理。 2)C#通過接口和虛方法實現多態性,C 使用虛函數和純虛函數。 3)C#的性能優化依賴於結構體和並行編程,C 則通過內聯函數和多線程實現。
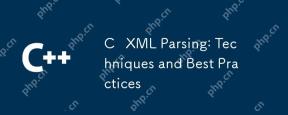
C 中解析XML數據可以使用DOM和SAX方法。 1)DOM解析將XML加載到內存,適合小文件,但可能佔用大量內存。 2)SAX解析基於事件驅動,適用於大文件,但無法隨機訪問。選擇合適的方法並優化代碼可提高效率。
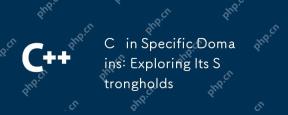
C 在遊戲開發、嵌入式系統、金融交易和科學計算等領域中的應用廣泛,原因在於其高性能和靈活性。 1)在遊戲開發中,C 用於高效圖形渲染和實時計算。 2)嵌入式系統中,C 的內存管理和硬件控制能力使其成為首選。 3)金融交易領域,C 的高性能滿足實時計算需求。 4)科學計算中,C 的高效算法實現和數據處理能力得到充分體現。
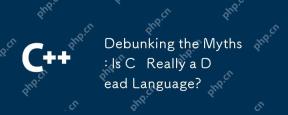
C 沒有死,反而在許多關鍵領域蓬勃發展:1)遊戲開發,2)系統編程,3)高性能計算,4)瀏覽器和網絡應用,C 依然是主流選擇,展現了其強大的生命力和應用場景。
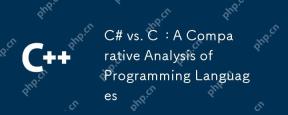
C#和C 的主要區別在於語法、內存管理和性能:1)C#語法現代,支持lambda和LINQ,C 保留C特性並支持模板。 2)C#自動內存管理,C 需要手動管理。 3)C 性能優於C#,但C#性能也在優化中。
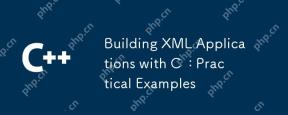
在C 中處理XML數據可以使用TinyXML、Pugixml或libxml2庫。 1)解析XML文件:使用DOM或SAX方法,DOM適合小文件,SAX適合大文件。 2)生成XML文件:將數據結構轉換為XML格式並寫入文件。通過這些步驟,可以有效地管理和操作XML數據。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
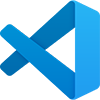
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器
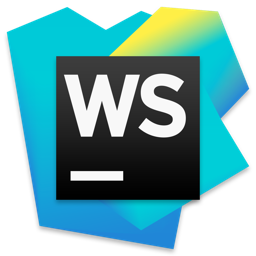
WebStorm Mac版
好用的JavaScript開發工具

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

記事本++7.3.1
好用且免費的程式碼編輯器