如果一個數可以表示為兩個奇質數對的加法,則該數稱為哥德巴赫數。
如果我們遵循上述條件,那麼我們可以發現,每個大於4的偶數都是哥德巴赫數,因為它必須有任意一對奇素數對。但奇數並不令人滿意,因為我們知道兩個數字相加永遠不可能是奇數。
在本文中,我們將了解如何使用 Java 程式語言檢查一個數字是否為哥德巴赫數。
向您展示一些實例
實例1
輸入數字為50。
讓我們用哥德巴赫數的邏輯來檢驗一下。
求奇素數對,我們得到:
(3 , 47) (7 , 43) (13 , 37) (19 , 31)
正如我們在這裡注意到的,我們得到了一些奇素數對,它們的加法值等於 50。
因此,50 是一個哥德巴赫數。
實例2
輸入數字為47。
讓我們用哥德巴赫數的邏輯來檢驗一下。
找到奇數素數對,我們得到− 沒有可用的質數對
正如我們在這裡注意到的,我們沒有得到任何加法值等於 47 的奇素數對。
因此,47 不是哥德巴赫數。
哥德巴赫數的其他一些例子包括 20、52、48、122 等。
演算法
第 1 步 - 透過初始化或使用者輸入來取得整數。
步驟 2 - 然後宣告兩個連續儲存素數的陣列。
步驟 3 - 然後開始迭代,迭代中將從兩個陣列中找到兩個奇素數對,其加法與輸入數相同。
步驟 4 - 如果我們得不到任何奇素數對,那麼我們可以印出給定的數字不是哥德巴赫數。
第 5 步 - 如果我們得到一些對,那麼我們只需列印這些對以及輸入數字是哥德巴赫數的結果訊息。
多種方法
我們透過不同的方式提供了解決方案。
透過使用靜態輸入值
#透過使用使用者定義的方法
讓我們一一看看該程式及其輸出。
方法 1:使用靜態輸入值
在這個方法中,將在程式中初始化一個整數值,然後透過使用演算法我們可以檢查一個數字是否是哥德巴赫數字。
範例
import java.io.*; import java.util.*; public class Main { public static void main(String args[]) { //declare all the variables int i, j, n, temp, b=0, c=0, sum=0; //declare a variable which stores the input number //assign a value to it int inputNumber=30; //declare a temporary variable which stores the input value temp=inputNumber; //declare two arrays with the capacity equal to input number int array1[]=new int[inputNumber]; int array2[]=new int[inputNumber]; //check whether the number is even or if(inputNumber%2!=0) { //if the input is not even then print it is not a Goldbach number System.out.println(inputNumber + " is not a Goldbach number."); } //if the input is even then proceed with further calculations else { //initiate the loop for finding the prime numbers for(i=1; i<=inputNumber; i++) { for(j=1; j<=i; j++) { if(i%j==0) { c++; } } //find the odd prime numbers if((c==2)&&(i%2!=0)) { //stores odd prime numbers into first array array1[b]=i; //stores odd prime numbers into second array array2[b]=i; //increments the value of b by 1 b++; } c=0; } //print the odd prime number pairs System.out.println("Odd Prime Pairs are: "); //loop for printing the value of ArrayStoreException for(i=0; i<b; i++) { for(j=i; j<b; j++) { //find the sum of two odd prime numbers sum=array1[i]+array2[j]; //condition for comparing the sum value with input number if(sum==temp) { //print pair of odd prime numbers System.out.print("(" + array1[i]+" , "+array2[j] + ")"); System.out.println(); } } } //print the final result if it is Goldbach number System.out.println(temp+" is a Goldbach number."); } } }
輸出
Odd Prime Pairs are: (7 , 23) (11 , 19) (13 , 17) 30 is a Goldbach number.
方法2:使用使用者定義的方法
在此方法中,初始化一個整數值,然後我們透過將此輸入數字作為參數傳遞來呼叫使用者定義的方法。
在該方法中,我們將使用演算法檢查一個數字是否為哥德巴赫數字。
範例
import java.io.*; import java.util.*; public class Main { public static void main(String args[]) { //declare a variable which stores the input number //assign a value to it int inp=98; if(checkGoldbach(inp)) { //if true it is Goldbach number System.out.println(inp+" is a Goldbach number."); } else { //if false it is not a Goldbach number System.out.println(inp + " is not a Goldbach number."); } } //define the user defined method static boolean checkGoldbach(int inputNumber) { //declare all the variables int i, j, n, temp, b=0, c=0, sum=0; //declare a temporary variable which stores the input value temp=inputNumber; //declare two arrays with the capacity equal to input number int array1[]=new int[inputNumber]; int array2[]=new int[inputNumber]; //check whether the number is even or if(inputNumber%2!=0) { return false; } //if the input is even then proceed with further calculations else { //initiate the loop for finding the prime numbers for(i=1; i<=inputNumber; i++) { for(j=1; j<=i; j++) { if(i%j==0) { c++; } } //find the odd prime numbers if((c==2)&&(i%2!=0)) { //stores odd prime numbers into first array array1[b]=i; //stores odd prime numbers into second array array2[b]=i; //increments the value of b by 1 b++; } c=0; } //print the odd prime number pairs System.out.println("Odd Prime Pairs are: "); //loop for printing the value of Arrays for(i=0; i<b; i++) { for(j=i; j<b; j++) { //find the sum of two odd prime numbers sum=array1[i]+array2[j]; //condition for comparing the sum value with input number if(sum==temp) { //print pair of odd prime numbers System.out.print("(" + array1[i]+" , "+array2[j] + ")"); System.out.println(); } } } return true; } } }
輸出
Odd Prime Pairs are: (19 , 79) (31 , 67) (37 , 61) 98 is a Goldbach number.
在本文中,我們探討如何使用三種不同的方法在 Java 中檢查一個數字是否為哥德巴赫數。
以上是在Java中如何檢查一個數字是否為哥德巴赫數?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

SublimeText3漢化版
中文版,非常好用
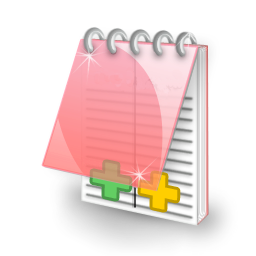
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能

Atom編輯器mac版下載
最受歡迎的的開源編輯器

禪工作室 13.0.1
強大的PHP整合開發環境