如何處理C 大數據開發中的資料冗餘問題?
資料冗餘是指在開發過程中,多次儲存相同或相似的數據,導致資料儲存空間浪費,嚴重影響程式的效能和效率。在大數據開發中,資料冗餘問題特別突出,因此解決資料冗餘問題是提高大數據開發效率和降低資源消耗的重要任務。
本文將介紹如何使用C 語言來處理大數據開發中的資料冗餘問題,並提供對應的程式碼範例。
一、使用指標減少資料複製
在處理大數據時,常常需要進行資料複製操作,這會耗費大量時間和記憶體。為了解決這個問題,我們可以使用指標來減少資料複製。以下是一個範例程式碼:
#include <iostream> int main() { int* data = new int[1000000]; // 假设data为一个大数据数组 // 使用指针进行数据操作 int* temp = data; for (int i = 0; i < 1000000; i++) { *temp++ = i; // 数据赋值操作 } // 使用指针访问数据 temp = data; for (int i = 0; i < 1000000; i++) { std::cout << *temp++ << " "; // 数据读取操作 } delete[] data; // 释放内存 return 0; }
在上面的程式碼中,我們使用指標temp來取代複製操作,這樣可以減少資料的複製次數,提高程式碼的執行效率。
二、使用資料壓縮技術減少儲存空間
資料冗餘導致儲存空間的浪費,為了解決這個問題,我們可以使用壓縮技術來減少資料的儲存空間。常用的資料壓縮演算法有哈夫曼編碼、LZW壓縮演算法等。以下是使用哈夫曼編碼進行資料壓縮的範例程式碼:
#include <iostream> #include <queue> #include <vector> #include <map> struct Node { int frequency; char data; Node* left; Node* right; Node(int freq, char d) { frequency = freq; data = d; left = nullptr; right = nullptr; } }; struct compare { bool operator()(Node* left, Node* right) { return (left->frequency > right->frequency); } }; void generateCodes(Node* root, std::string code, std::map<char, std::string>& codes) { if (root == nullptr) { return; } if (root->data != '') { codes[root->data] = code; } generateCodes(root->left, code + "0", codes); generateCodes(root->right, code + "1", codes); } std::string huffmanCompression(std::string text) { std::map<char, int> frequencies; for (char c : text) { frequencies[c]++; } std::priority_queue<Node*, std::vector<Node*>, compare> pq; for (auto p : frequencies) { pq.push(new Node(p.second, p.first)); } while (pq.size() > 1) { Node* left = pq.top(); pq.pop(); Node* right = pq.top(); pq.pop(); Node* newNode = new Node(left->frequency + right->frequency, ''); newNode->left = left; newNode->right = right; pq.push(newNode); } std::map<char, std::string> codes; generateCodes(pq.top(), "", codes); std::string compressedText = ""; for (char c : text) { compressedText += codes[c]; } return compressedText; } std::string huffmanDecompression(std::string compressedText, std::map<char, std::string>& codes) { Node* root = new Node(0, ''); Node* current = root; std::string decompressedText = ""; for (char c : compressedText) { if (c == '0') { current = current->left; } else { current = current->right; } if (current->data != '') { decompressedText += current->data; current = root; } } delete root; return decompressedText; } int main() { std::string text = "Hello, world!"; std::string compressedText = huffmanCompression(text); std::cout << "Compressed text: " << compressedText << std::endl; std::map<char, std::string> codes; generateCodes(compressedText, "", codes); std::string decompressedText = huffmanDecompression(compressedText, codes); std::cout << "Decompressed text: " << decompressedText << std::endl; return 0; }
在上面的程式碼中,我們使用哈夫曼編碼對文字進行壓縮。首先統計文本中每個字元的頻率,然後根據頻率建立哈夫曼樹。接著產生每個字元的編碼,用0和1表示編碼,減少儲存空間的佔用。最後將文字進行壓縮和解壓縮,並輸出結果。
總結:
透過使用指標減少資料複製和使用資料壓縮技術減少儲存空間,我們可以有效解決大數據開發中的資料冗餘問題。在實際開發中,需要根據具體情況選擇合適的方法來處理資料冗餘,以提高程式的效能和效率。
以上是如何處理C++大數據開發中的資料冗餘問題?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
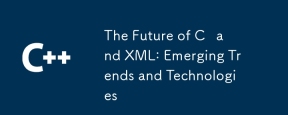
C 和XML的未來發展趨勢分別為:1)C 將通過C 20和C 23標準引入模塊、概念和協程等新特性,提升編程效率和安全性;2)XML將繼續在數據交換和配置文件中佔據重要地位,但會面臨JSON和YAML的挑戰,並朝著更簡潔和易解析的方向發展,如XMLSchema1.1和XPath3.1的改進。
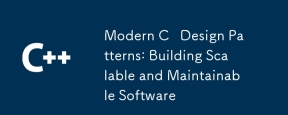
現代C 設計模式利用C 11及以後的新特性實現,幫助構建更靈活、高效的軟件。 1)使用lambda表達式和std::function簡化觀察者模式。 2)通過移動語義和完美轉發優化性能。 3)智能指針確保類型安全和資源管理。
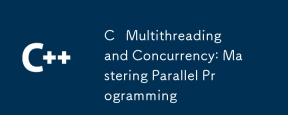
C 多線程和並發編程的核心概念包括線程的創建與管理、同步與互斥、條件變量、線程池、異步編程、常見錯誤與調試技巧以及性能優化與最佳實踐。 1)創建線程使用std::thread類,示例展示瞭如何創建並等待線程完成。 2)同步與互斥使用std::mutex和std::lock_guard保護共享資源,避免數據競爭。 3)條件變量通過std::condition_variable實現線程間的通信和同步。 4)線程池示例展示瞭如何使用ThreadPool類並行處理任務,提高效率。 5)異步編程使用std::as
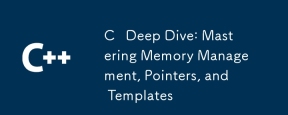
C 的內存管理、指針和模板是核心特性。 1.內存管理通過new和delete手動分配和釋放內存,需注意堆和棧的區別。 2.指針允許直接操作內存地址,使用需謹慎,智能指針可簡化管理。 3.模板實現泛型編程,提高代碼重用性和靈活性,需理解類型推導和特化。
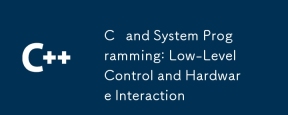
C 適合系統編程和硬件交互,因為它提供了接近硬件的控制能力和麵向對象編程的強大特性。 1)C 通過指針、內存管理和位操作等低級特性,實現高效的系統級操作。 2)硬件交互通過設備驅動程序實現,C 可以編寫這些驅動程序,處理與硬件設備的通信。
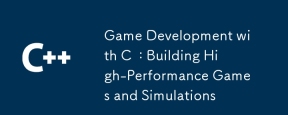
C 適合構建高性能遊戲和仿真係統,因為它提供接近硬件的控制和高效性能。 1)內存管理:手動控制減少碎片,提高性能。 2)編譯時優化:內聯函數和循環展開提昇運行速度。 3)低級操作:直接訪問硬件,優化圖形和物理計算。
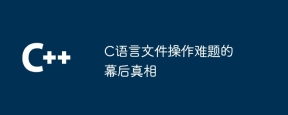
文件操作難題的真相:文件打開失敗:權限不足、路徑錯誤、文件被佔用。數據寫入失敗:緩衝區已滿、文件不可寫、磁盤空間不足。其他常見問題:文件遍歷緩慢、文本文件編碼不正確、二進製文件讀取錯誤。
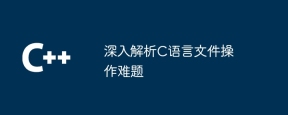
深入解析C語言文件操作難題前言文件操作是C語言編程中一項重要的功能。然而,它也可能是一個有挑戰性的領域,尤其是在處理複雜文件結構時。本文將深入解析C語言文件操作的常見難題,並提供實戰案例來闡明解決方法。打開和關閉文件打開文件時,有兩種主要的模式:r(只讀)和w(寫只)。要打開文件,可以使用fopen()函數:FILE*fp=fopen("file.txt","r");打開文件後,必須在使用完後將其關閉,以釋放資源:fclose(fp);讀取和寫入數據可以使


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
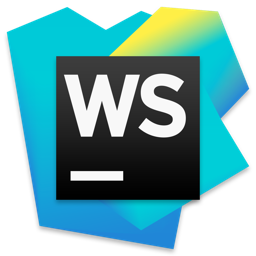
WebStorm Mac版
好用的JavaScript開發工具

禪工作室 13.0.1
強大的PHP整合開發環境

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!
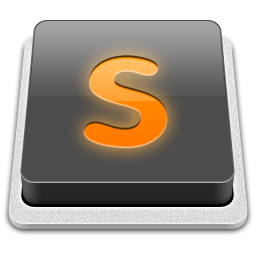
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中