在Python中,可以使用清單來在單一變數中維護多個項目。清單是Python的四種內建資料類型之一,用於儲存資料集合。另外三種類型,元組、集合和字典,各自有不同的功能。列表使用方括號進行建構。由於清單不必是同質的,它們是Python中最有用的工具。一個列表可以包含字串、物件和整數等資料類型。列表可以在生成後進行修改,因為它們是可變的。
本文的重點是速記和用一句話或一個字來表達這個概念的許多捷徑。這個操作對程式設計師來說非常重要,可以完成許多工作。我們將使用Python來展示四種不同的方法來完成這個任務。
Using The List Comprehension
在使用這種方法時,我們只需要在特定位置旋轉後重新分配清單中每個元素的索引。由於其較小的實現,這種方法在完成任務中起著重要作用。
Algorithm
- Defining a list first.
- #Use the list comprehension.
- #For applying two different sides right(i-index) and left(i index).
- Print the output list.
文法
#左旋轉
list_1 = [list_1[(i + 3) % len(list_1)]#For right rotate
list_1 = [list_1[(i - 3) % len(list_1)]
ExampleHere, in this code we have used the list comprehension to rotate the elements in a list that is the right and left rotate. For loop is used to iterate through the list of elements.
list_1 = [10, 14, 26, 37, 42]
print (" Primary list : " + str(list_1))
list_1 = [list_1[(i + 3) % len(list_1)]
for i, x in enumerate(list_1)]
print ("Output of the list after left rotate by 3 : " + str(list_1))
list_1 = [list_1[(i - 3) % len(list_1)]
for i, x in enumerate(list_1)]
print ("Output of the list after right rotate by 3(back to primary list) : "+str(list_1))
list_1 = [list_1[(i + 2) % len(list_1)]
for i, x in enumerate(list_1)]
print ("Output of the list after left rotate by 2 : " + str(list_1))
list_1 = [list_1[(i - 2) % len(list_1)]
for i, x in enumerate(list_1)]
print ("Output of the list after right rotate by 2 : "+ str(list_1))
輸出
Primary list : [10, 14, 26, 37, 42] Output of the list after left rotate by 3 : [37, 42, 10, 14, 26] Output of the list after right rotate by 3(back to primary list) : [10, 14, 26, 37, 42] Output of the list after left rotate by 2 : [26, 37, 42, 10, 14] Output of the list after right rotate by 2 : [10, 14, 26, 37, 42]Here, in this code we have used the list comprehension to rotate the elements in a list that is the right and left rotate. For loop is used to iterate through the list of elements.
使用切片
This specific technique is the standard technique. With the rotation number, it simply joins the later-sliced component to the earlier-sliced part.
Algorithm
- Defining a list first.
- #Use slicing method.
- #在右旋和左旋後列印每個清單。
文法
FOR SLICING
#左旋轉 -
list_1 = list_1[3:] + list_1[:3]#右旋轉 -
list_1 = list_1[-3:] + list_1[:-3]
ExampleThe following program rearranges the elements of a list. The original list is [11, 34, 26, 57, 92]. First rotate 3 units to the left. That is, the first three elements are moved to the end, the first three elements are moved to the end, resulting in [57, 92, 11, 34, 26]. Then rotate right by 3 so the last three elements move back and forth to their original positions [11,34,26,57,92].
接著向右旋轉2次,使最後兩個元素向前移動,得到 [26, 57, 92, 11, 34]。最後向左旋轉1次,將一個元素從開頭移到結尾,得到 [57, 92, 11, 34, 26]。
list_1 = [11, 34, 26, 57, 92]
print (" Primary list : " + str(list_1))
list_1 = list_1[3:] + list_1[:3]
print ("Output of the list after left rotate by 3 : " + str(list_1))
list_1 = list_1[-3:] + list_1[:-3]
print ("Output of the list after right rotate by 3(back to Primary list) : "+str(list_1))
list_1 = list_1[-2:] + list_1[:-2]
print ("Output of the list after right rotate by 2 : "+ str(list_1))
list_1 = list_1[1:] + list_1[:1]
print ("Output of the list after left rotate by 1 : " + str(list_1))
輸出
Primary list : [11, 34, 26, 57, 92]
Output of the list after left rotate by 3 : [57, 92, 11, 34, 26]
Output of the list after right rotate by 3(back to Primary list) : [11, 34, 26, 57, 92]
Output of the list after right rotate by 2 : [57, 92, 11, 34, 26]
Output of the list after left rotate by 1 : [92, 11, 34, 26, 57]
Using The Numpy Module使用給定的軸,我們也可以使用python中的numpy.roll模組來旋轉清單中的元素。輸入數組的元素會因此被移動。如果一個元素從第一個位置移動到最後一個位置,它會回到初始位置。
Algorithm
- 導入numpy.roll模組
- Define the list and give the particular index.
- Print the output list.
Example
A list 'number' is created and assigned the values 1, 2, 4, 10, 18 and 83. The variable i is set to 1. The np.roll()
function from the NumPy library is then used on the list number with an argument of i which shifts each element in the list by 1 index position (the first element becomes last).
import numpy as np
if __name__ == '__main__':
number = [1, 2, 4, 10, 18, 83]
i = 1
x = np.roll(number, i)
print(x)
輸出
[83 1 2 4 10 18]
Usingcollections.deque.rotate()rotate()函數是由collections模組中的deque類別提供的內建函數,用於實現旋轉操作。儘管不太為人所知,但這個函數更加實用。
Algorithm
- 首先從collection模組匯入deque類別。
- 定義一個列表
- #列印主要清單
- #使用rotate()函數旋轉元素
- Print the output.
Example
The following program uses the deque data structure from the collections module to rotate a list. The original list is printed, then it rotates left by 3 and prints out the new rotated list. It then rotates right to position ) by 3 and prints out the resulting list.
from collections import deque
list_1 = [31, 84, 76, 97, 82]
print ("Primary list : " + str(list_1))
list_1 = deque(list_1)
list_1.rotate(-3)
list_1 = list(list_1)
print ("Output list after left rotate by 3 : " + str(list_1))
list_1 = deque(list_1)
list_1.rotate(3)
list_1 = list(list_1)
print ("Output list after right rotate by 3(back to primary list) : "+ str(list_1))
輸出
Primary list : [31, 84, 76, 97, 82]
Output list after left rotate by 3 : [97, 82, 31, 84, 76]
Output list after right rotate by 3(back to primary list) : [31, 84, 76, 97, 82]
結論在本文中,我們簡要地解釋了四種不同的方法來旋轉清單中的元素。 ###
以上是Python程式旋轉列表元素的詳細內容。更多資訊請關注PHP中文網其他相關文章!
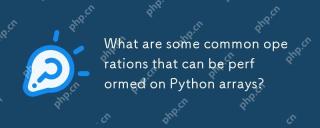
Pythonarrayssupportvariousoperations:1)Slicingextractssubsets,2)Appending/Extendingaddselements,3)Insertingplaceselementsatspecificpositions,4)Removingdeleteselements,5)Sorting/Reversingchangesorder,and6)Listcomprehensionscreatenewlistsbasedonexistin
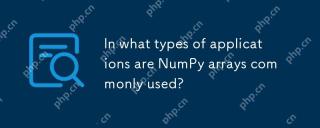
NumPyarraysareessentialforapplicationsrequiringefficientnumericalcomputationsanddatamanipulation.Theyarecrucialindatascience,machinelearning,physics,engineering,andfinanceduetotheirabilitytohandlelarge-scaledataefficiently.Forexample,infinancialanaly
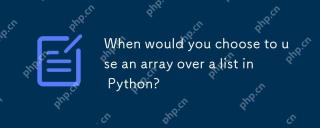
useanArray.ArarayoveralistinpythonwhendeAlingwithHomoGeneData,performance-Caliticalcode,orinterfacingwithccode.1)同質性data:arraysSaveMemorywithTypedElements.2)績效code-performance-calitialcode-calliginal-clitical-clitical-calligation-Critical-Code:Arraysofferferbetterperbetterperperformanceformanceformancefornallancefornalumericalical.3)
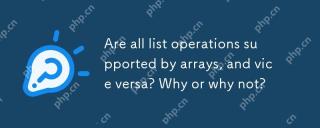
不,notalllistoperationsareSupportedByArrays,andviceversa.1)arraysdonotsupportdynamicoperationslikeappendorinsertwithoutresizing,wheremactsperformance.2)listssdonotguaranteeconecontanttanttanttanttanttanttanttanttanttimecomplecomecomplecomecomecomecomecomecomplecomectacccesslectaccesslecrectaccesslerikearraysodo。
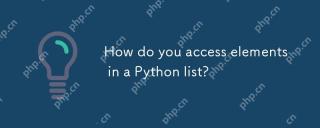
toAccesselementsInapythonlist,useIndIndexing,負索引,切片,口頭化。 1)indexingStartSat0.2)否定indexingAccessesessessessesfomtheend.3)slicingextractsportions.4)iterationerationUsistorationUsisturessoreTionsforloopsoreNumeratorseforeporloopsorenumerate.alwaysCheckListListListListlentePtotoVoidToavoIndexIndexIndexIndexIndexIndExerror。
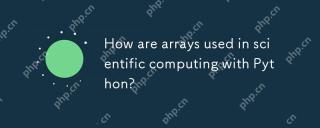
Arraysinpython,尤其是Vianumpy,ArecrucialInsCientificComputingfortheireftheireffertheireffertheirefferthe.1)Heasuedfornumerericalicerationalation,dataAnalysis和Machinelearning.2)Numpy'Simpy'Simpy'simplementIncressionSressirestrionsfasteroperoperoperationspasterationspasterationspasterationspasterationspasterationsthanpythonlists.3)inthanypythonlists.3)andAreseNableAblequick
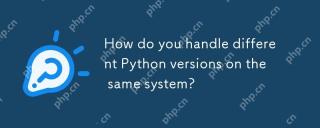
你可以通過使用pyenv、venv和Anaconda來管理不同的Python版本。 1)使用pyenv管理多個Python版本:安裝pyenv,設置全局和本地版本。 2)使用venv創建虛擬環境以隔離項目依賴。 3)使用Anaconda管理數據科學項目中的Python版本。 4)保留系統Python用於系統級任務。通過這些工具和策略,你可以有效地管理不同版本的Python,確保項目順利運行。
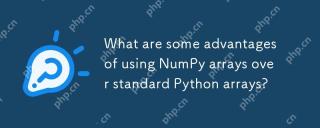
numpyarrayshaveseveraladagesoverandastardandpythonarrays:1)基於基於duetoc的iMplation,2)2)他們的aremoremoremorymorymoremorymoremorymoremorymoremoremory,尤其是WithlargedAtasets和3)效率化,效率化,矢量化函數函數函數函數構成和穩定性構成和穩定性的操作,製造


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。
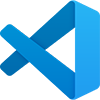
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

SublimeText3漢化版
中文版,非常好用
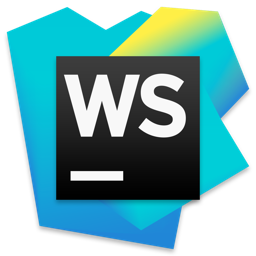
WebStorm Mac版
好用的JavaScript開發工具