Golang實作圖片去雜訊和降噪的方法
圖片去雜訊和降噪是影像處理中常見的問題,它們能夠有效地移除圖片中的噪聲,提高影像的品質和清晰度。 Golang作為一種高效且並發能力強的程式語言,可以實現這些影像處理任務。本文將介紹如何使用Golang實現圖片去噪和降噪的方法,並給出對應的程式碼範例。
- 圖片去雜訊的基本原理
圖片去雜訊的基本原理是透過濾波器對影像進行處理,將雜訊部分進行濾除,從而得到去雜訊後的影像。常用的濾波器有中值濾波器、均值濾波器等。在Golang中,我們可以使用映像處理庫github.com/nfnt/resize
和github.com/disintegration/imaging
來實現圖片的濾波處理。 - 使用中值濾波器去雜訊
中值濾波器是一種常用的去雜訊方法,它的原理是用像素點周圍的鄰近像素的中值來取代目前像素的值。以下是使用Golang實作中值濾波器去雜訊的程式碼範例:
import ( "image" _ "image/jpeg" "os" "github.com/disintegration/imaging" ) func medianFilter(imgPath string) image.Image { // 打开原始图片 file, err := os.Open(imgPath) if err != nil { panic(err) } defer file.Close() // 解码图片 img, _, err := image.Decode(file) if err != nil { panic(err) } // 使用中值滤波器处理图片 filteredImg := imaging.Median(img, 3) return filteredImg } func main() { // 原始图片路径 imgPath := "original.jpg" // 处理图片 filteredImg := medianFilter(imgPath) // 保存处理后的图片 err := imaging.Save(filteredImg, "filtered.jpg") if err != nil { panic(err) } }
在上述程式碼中,我們首先使用os.Open
函數開啟原始圖片,然後使用image.Decode
函數解碼圖片取得image.Image
物件。接著,我們使用中值濾波器對圖片進行處理,其中imaging.Median
函數的第二個參數表示濾波器的大小,這裡我們設定為3。最後,使用imaging.Save
函數將處理後的圖片儲存到磁碟。
- 使用均值濾波器降噪
均值濾波器是另一種常用的降噪方法,它的原理是用像素點周圍的鄰近像素的平均值來代替當前像素的值。以下是使用Golang實作均值濾波器降噪的程式碼範例:
import ( "image" _ "image/jpeg" "os" "github.com/disintegration/imaging" ) func meanFilter(imgPath string) image.Image { // 打开原始图片 file, err := os.Open(imgPath) if err != nil { panic(err) } defer file.Close() // 解码图片 img, _, err := image.Decode(file) if err != nil { panic(err) } // 使用均值滤波器处理图片 filteredImg := imaging.Blur(img, 3) return filteredImg } func main() { // 原始图片路径 imgPath := "original.jpg" // 处理图片 filteredImg := meanFilter(imgPath) // 保存处理后的图片 err := imaging.Save(filteredImg, "filtered.jpg") if err != nil { panic(err) } }
在上述程式碼中,我們使用imaging.Blur
函數實作了均值濾波器的降噪效果。同樣的,可以透過調整第二個參數來控制濾波器的大小。
透過以上程式碼範例,我們實作了基於中值濾波器和平均值濾波器的圖片去噪和降噪方法。當然,除了中值濾波器和均值濾波器,還有其他更複雜的濾波器,可以根據實際的需求進行選擇和實現。同時,Golang提供了強大的並發能力,可以進一步優化影像處理的效率。希望本文能夠幫助您。
以上是Golang實現圖片去雜訊和降噪的方法的詳細內容。更多資訊請關注PHP中文網其他相關文章!
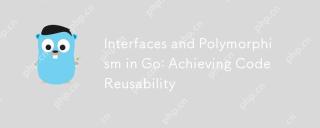
Interfacesand -polymormormormormormingingoenhancecodereusanity和Maintainability.1)defineInterfaceSattherightabStractractionLevel.2)useInterInterFacesFordEffordExpentIndention.3)ProfileCodeTomeAgePerformancemacts。
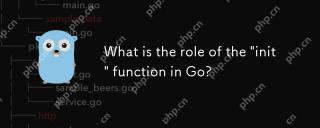
initiTfunctioningOrunSautomation beforeTheMainFunctionToInitializePackages andSetUptheNvironment.it'susefulforsettingupglobalvariables,資源和performingOne-timesEtepaskSarpaskSacraskSacrastAscacrAssanyPackage.here'shere'shere'shere'shere'shodshowitworks:1)Itcanbebeusedinanananainapthecate,NotjustAckAckAptocakeo
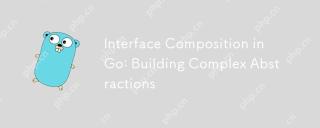
接口組合在Go編程中通過將功能分解為小型、專注的接口來構建複雜抽象。 1)定義Reader、Writer和Closer接口。 2)通過組合這些接口創建如File和NetworkStream的複雜類型。 3)使用ProcessData函數展示如何處理這些組合接口。這種方法增強了代碼的靈活性、可測試性和可重用性,但需注意避免過度碎片化和組合複雜性。
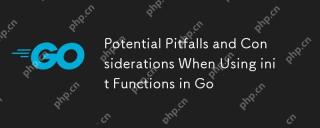
initfunctionsingoareAutomationalCalledBeLedBeForeTheMainFunctionandAreuseFulforSetupButcomeWithChallenges.1)executiondorder:totiernitFunctionSrunIndIndefinitionorder,cancancapationSifsUsiseSiftheyDepplothother.2)測試:sterfunctionsmunctionsmunctionsMayInterfionsMayInterferfereWithTests,b
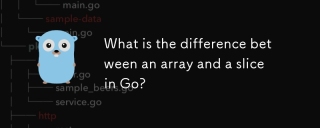
本文討論了GO中的數組和切片之間的差異,重點是尺寸,內存分配,功能傳遞和用法方案。陣列是固定尺寸的,分配的堆棧,而切片是動態的,通常是堆積的,並且更靈活。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

禪工作室 13.0.1
強大的PHP整合開發環境
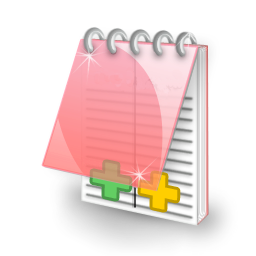
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能
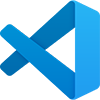
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。
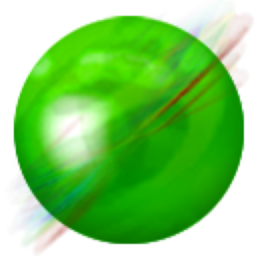
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境