Python是一種功能強大的程式語言,它提供了許多高階程式庫和模組來幫助我們解決各種問題。其中之一就是itertools模組,它提供了一組用於迭代器操作的函數。本文將介紹如何在Python 3.x中使用itertools模組進行迭代器操作,並提供一些程式碼範例。
首先,我們要了解什麼是迭代器。迭代器是一種可迭代對象,它可以依照一定的規則產生一個序列。使用迭代器可以更有效率地處理大量數據,減少記憶體消耗。而itertools模組提供了一些函數,可以產生各種不同類型的迭代器,方便我們進行迭代器操作。
下面是一些常用的itertools函數以及它們的用法和程式碼範例:
- count():產生一個無限迭代器,從指定的起始值開始,每次遞增指定的步長。
from itertools import count for i in count(5, 2): if i > 10: break print(i)
輸出:
5 7 9 11
- cycle():對一個可迭代物件進行無限迴圈。
from itertools import cycle colors = ['red', 'green', 'blue'] count = 0 for color in cycle(colors): if count > 10: break print(color) count += 1
輸出:
red green blue red green blue red green blue red green
- repeat():產生一個重複的值。
from itertools import repeat for i in repeat('hello', 3): print(i)
輸出:
hello hello hello
- chain():將多個可迭代物件連接起來。
from itertools import chain colors = ['red', 'green', 'blue'] numbers = [1, 2, 3] for item in chain(colors, numbers): print(item)
輸出:
red green blue 1 2 3
- compress():根據指定的遮罩過濾可迭代物件的元素。
from itertools import compress letters = ['a', 'b', 'c', 'd', 'e'] mask = [True, False, False, True, False] filtered_letters = compress(letters, mask) for letter in filtered_letters: print(letter)
輸出:
a d
- dropwhile():丟棄可迭代物件中滿足指定條件的元素,直到遇到第一個不滿足條件的元素。
from itertools import dropwhile numbers = [1, 3, 5, 2, 4, 6] result = dropwhile(lambda x: x < 4, numbers) for number in result: print(number)
輸出:
5 2 4 6
- takewhile():傳回可迭代物件中滿足指定條件的元素,直到遇到第一個不滿足條件的元素。
from itertools import takewhile numbers = [1, 3, 5, 2, 4, 6] result = takewhile(lambda x: x < 4, numbers) for number in result: print(number)
輸出:
1 3
- permutations():產生可迭代物件的所有排列組合。
from itertools import permutations items = ['a', 'b', 'c'] result = permutations(items) for permutation in result: print(permutation)
輸出:
('a', 'b', 'c') ('a', 'c', 'b') ('b', 'a', 'c') ('b', 'c', 'a') ('c', 'a', 'b') ('c', 'b', 'a')
以上僅是itertools模組中的一部分函數。透過使用這些函數,我們可以更方便地進行迭代器操作,提高程式碼的效率和可讀性。
總結來說,itertools模組提供了一組強大的函數,用於產生和操作各種類型的迭代器。透過靈活地使用這些函數,我們可以更好地處理和操作數據,提高程式碼的效能。希望本文對你在Python 3.x中使用itertools模組進行迭代器操作有所幫助。
以上是Python 3.x 中如何使用itertools模組進行迭代器操作的詳細內容。更多資訊請關注PHP中文網其他相關文章!
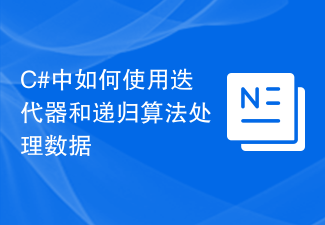
C#中如何使用迭代器和递归算法处理数据,需要具体代码示例在C#中,迭代器和递归算法是两种常用的数据处理方法。迭代器可以帮助我们遍历集合中的元素,而递归算法则能够有效地处理复杂的问题。本文将详细介绍如何使用迭代器和递归算法来处理数据,并提供具体的代码示例。使用迭代器处理数据在C#中,我们可以使用迭代器来遍历集合中的元素,而无需事先知道集合的大小。通过迭代器,我
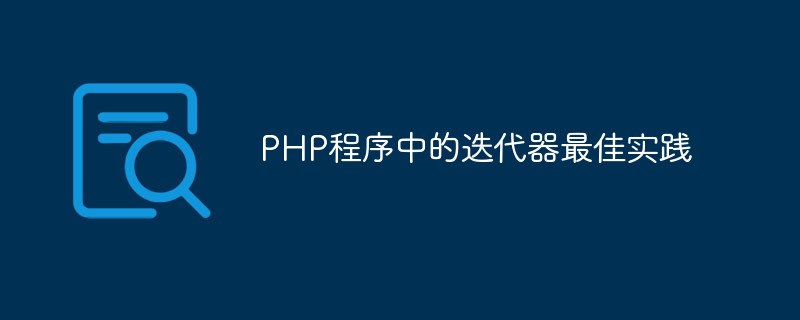
PHP程序中的迭代器最佳实践迭代器在PHP编程中是一种非常常用的设计模式。通过实现迭代器接口,我们可以遍历一个集合对象中的元素,而且还可以轻松的实现自己的迭代器对象。在PHP中,迭代器模式可以帮助我们更有效地操作数组、列表等集合对象。在本文中,我们将介绍PHP程序中迭代器的最佳实践,希望能帮助同样在迭代器应用方面工作的PHP开发人员。一、使用标准迭代器接口P
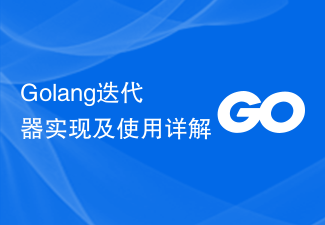
Golang是一个快速、高效的静态编译型语言,其简洁的语法和强大的性能让它在软件开发领域备受青睐。在Golang中,迭代器(Iterator)是一种常用的设计模式,用于遍历集合中的元素而无需暴露集合的内部结构。本文将详细介绍如何在Golang中实现和使用迭代器,通过具体的代码示例帮助读者更好地理解。1.迭代器的定义在Golang中,迭代器通常由一个接口和实
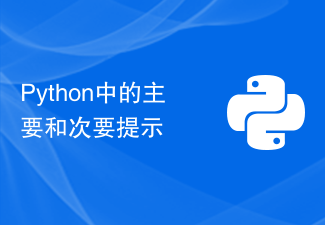
简介主要和次要提示,要求用户输入命令并与解释器进行通信,使得这种交互模式成为可能。主要提示通常由>>>表示,表示Python已准备好接收输入并执行相应的代码。了解这些提示的作用和功能对于发挥Python的交互式编程能力至关重要。在本文中,我们将讨论Python中的主要和次要提示符,强调它们的重要性以及它们如何增强交互式编程体验。我们将研究它们的功能、格式选择以及在快速代码创建、实验和测试方面的优势。开发人员可以通过理解主要和次要提示符来使用Python的交互模式,从而改善他们的
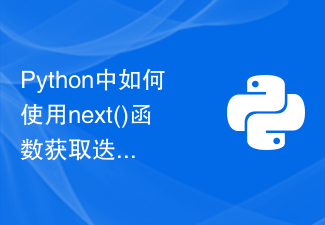
Python中如何使用next()函数获取迭代器的下一个元素迭代器是Python中很常用的一个概念,它允许我们按照特定的顺序遍历一个数据集合。在迭代过程中,我们经常需要获取迭代器的下一个元素,这时就可以使用next()函数来实现。在Python中,我们可以使用iter()函数将一个可迭代对象转换成一个迭代器。例如,如果我们有一个列表,可以将其转换成迭代器后进
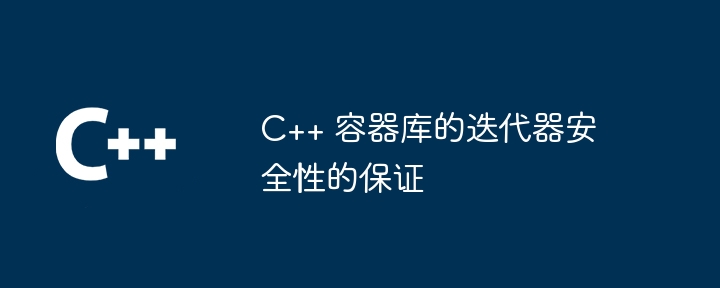
C++容器库提供以下机制确保迭代器的安全性:1.容器不变性保证;2.复制迭代器;3.范围for循环;4.Const迭代器;5.异常安全。
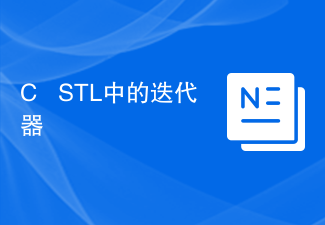
C++STL(StandardTemplateLibrary)是C++程序语言的标准库之一,它包含了一系列的标准数据结构和算法。在STL中,迭代器(iterator)是一种非常重要的工具,用于在STL的容器中进行遍历和访问。迭代器是一个类似于指针的对象,它可以指向容器(例如vector、list、set、map等)中的某个元素,并可以在容器中进行移动、

概念差异:Iterator:Iterator是一个接口,代表一个从集合中获取值的迭代器。它提供了MoveNext()、Current()和Reset()等方法,允许你遍历集合中的元素,并对当前元素进行操作。Iterable:Iterable也是一个接口,代表一个可迭代的对象。它提供了Iterator()方法,用于返回一个Iterator对象,以便于遍历集合中的元素。使用方式:Iterator:要使用Iterator,需要先获得一个Iterator对象,然后调用MoveNext()方法来移动到下一


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。
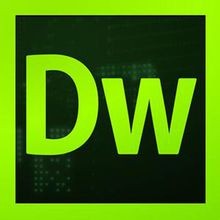
Dreamweaver CS6
視覺化網頁開發工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。
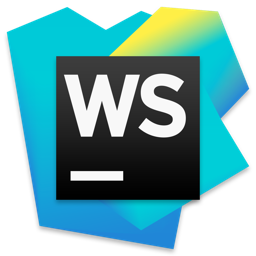
WebStorm Mac版
好用的JavaScript開發工具

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。