使用Zend框架實作API身分認證和存取控制的步驟
引言:
在現代應用程式中,使用API進行資料交換和服務呼叫是非常常見的。然而,為了確保資料的安全性和保護敏感資訊,我們需要對API進行身份認證和存取控制。本文將介紹如何使用Zend框架來實現API身分認證和存取控制,並提供相關的程式碼範例。
步驟1:安裝Zend框架
首先,我們需要在專案中安裝Zend框架。可以透過Composer進行安裝,執行以下命令:
composer require zendframework/zend-authentication composer require zendframework/zend-permissions-acl composer require zendframework/zend-expressive
步驟2:建立認證適配器
接下來,我們需要建立一個認證適配器來驗證API請求中的身份資訊。通常情況下,我們會將身分識別資訊儲存在資料庫或其他儲存系統中。這裡我們以資料庫為例來進行說明。首先,建立一個名為DbAdapter
的類,實作ZendAuthenticationAdapterAdapterInterface
接口,並編寫對應的驗證方法。具體程式碼範例如下:
use ZendAuthenticationAdapterAdapterInterface; use ZendAuthenticationResult; class DbAdapter implements AdapterInterface { protected $username; protected $password; public function __construct($username, $password) { $this->username = $username; $this->password = $password; } public function authenticate() { // 在此处根据数据库中的用户表验证用户名和密码的正确性 // 如果验证成功,返回Result::SUCCESS,否则返回Result::FAILURE } }
步驟3:建立身分驗證服務
接下來,我們需要建立一個身分驗證服務,用於處理API請求中的身分認證。在Zend框架中,我們可以使用ZendAuthenticationAuthenticationService
來實作。可以在全域設定檔中進行相關配置,程式碼如下:
return [ 'dependencies' => [ 'invokables' => [ 'AuthModelAdapter' => 'AuthModelDbAdapter', ], 'factories' => [ 'AuthServiceAuthenticationService' => function($container) { return new ZendAuthenticationAuthenticationService( $container->get('AuthModelAdapter') ); }, ], ], ];
步驟4:編寫API控制器
接下來,我們需要編寫API控制器,並在控制器的操作方法中進行身份認證和存取控制的判斷。具體程式碼範例如下:
use ZendAuthenticationResult; use ZendPermissionsAclAcl; class ApiController { protected $authService; protected $acl; public function __construct($authService, $acl) { $this->authService = $authService; $this->acl = $acl; } public function action() { // 进行身份认证 $result = $this->authService->authenticate(); // 判断认证结果 if ($result->getCode() != Result::SUCCESS) { return $this->unauthorizedResponse(); } // 获取认证成功的用户信息 $user = $result->getIdentity(); // 使用用户信息进行访问控制判断 if (!$this->acl->isAllowed($user->getRole(), 'resource', 'action')) { return $this->forbiddenResponse(); } // 执行API操作... return $this->successResponse(); } // 省略其他方法... }
步驟5:設定存取控制清單
最後,我們需要在全域設定檔中設定存取控制清單(ACL)。具體程式碼範例如下:
return [ 'acl' => [ 'roles' => [ 'guest', 'user', 'admin', ], 'resources' => [ 'resource', ], 'allow' => [ 'guest' => [ 'resource' => [ 'action', ], ], 'user' => [ 'resource' => [ 'action', ], ], 'admin' => [ 'resource' => [ 'action', ], ], ], ]; ];
總結:
使用Zend框架可以方便地實作API身分認證和存取控制。透過上述步驟,我們可以建立一個認證適配器來驗證使用者身分資訊,建立一個身分認證服務來處理API請求中的身分認證,編寫API控制器來進行身分認證和存取控制的判斷,以及設定存取控制清單。這樣就可以確保API的安全性,並依照使用者的角色進行對應的存取控制。
以上就是使用Zend框架實作API身分認證和存取控制的步驟和程式碼範例。希望本文對您在開發API時有所幫助。如有任何疑問,歡迎留言討論。謝謝!
以上是使用Zend框架實現API身份認證和存取控制的步驟的詳細內容。更多資訊請關注PHP中文網其他相關文章!
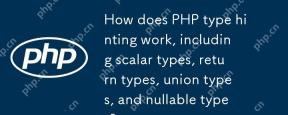
PHP類型提示提升代碼質量和可讀性。 1)標量類型提示:自PHP7.0起,允許在函數參數中指定基本數據類型,如int、float等。 2)返回類型提示:確保函數返回值類型的一致性。 3)聯合類型提示:自PHP8.0起,允許在函數參數或返回值中指定多個類型。 4)可空類型提示:允許包含null值,處理可能返回空值的函數。
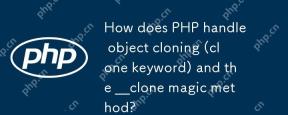
PHP中使用clone關鍵字創建對象副本,並通過\_\_clone魔法方法定制克隆行為。 1.使用clone關鍵字進行淺拷貝,克隆對象的屬性但不克隆對象屬性內的對象。 2.通過\_\_clone方法可以深拷貝嵌套對象,避免淺拷貝問題。 3.注意避免克隆中的循環引用和性能問題,優化克隆操作以提高效率。
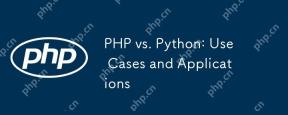
PHP適用於Web開發和內容管理系統,Python適合數據科學、機器學習和自動化腳本。 1.PHP在構建快速、可擴展的網站和應用程序方面表現出色,常用於WordPress等CMS。 2.Python在數據科學和機器學習領域表現卓越,擁有豐富的庫如NumPy和TensorFlow。
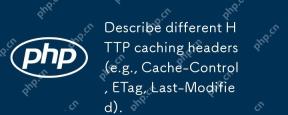
HTTP緩存頭的關鍵玩家包括Cache-Control、ETag和Last-Modified。 1.Cache-Control用於控制緩存策略,示例:Cache-Control:max-age=3600,public。 2.ETag通過唯一標識符驗證資源變化,示例:ETag:"686897696a7c876b7e"。 3.Last-Modified指示資源最後修改時間,示例:Last-Modified:Wed,21Oct201507:28:00GMT。
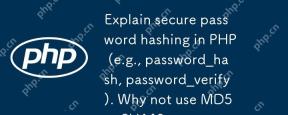
在PHP中,應使用password_hash和password_verify函數實現安全的密碼哈希處理,不應使用MD5或SHA1。1)password_hash生成包含鹽值的哈希,增強安全性。 2)password_verify驗證密碼,通過比較哈希值確保安全。 3)MD5和SHA1易受攻擊且缺乏鹽值,不適合現代密碼安全。
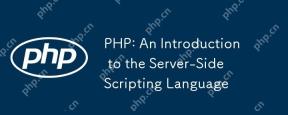
PHP是一種服務器端腳本語言,用於動態網頁開發和服務器端應用程序。 1.PHP是一種解釋型語言,無需編譯,適合快速開發。 2.PHP代碼嵌入HTML中,易於網頁開發。 3.PHP處理服務器端邏輯,生成HTML輸出,支持用戶交互和數據處理。 4.PHP可與數據庫交互,處理表單提交,執行服務器端任務。
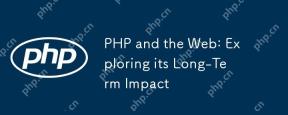
PHP在過去幾十年中塑造了網絡,並將繼續在Web開發中扮演重要角色。 1)PHP起源於1994年,因其易用性和與MySQL的無縫集成成為開發者首選。 2)其核心功能包括生成動態內容和與數據庫的集成,使得網站能夠實時更新和個性化展示。 3)PHP的廣泛應用和生態系統推動了其長期影響,但也面臨版本更新和安全性挑戰。 4)近年來的性能改進,如PHP7的發布,使其能與現代語言競爭。 5)未來,PHP需應對容器化、微服務等新挑戰,但其靈活性和活躍社區使其具備適應能力。
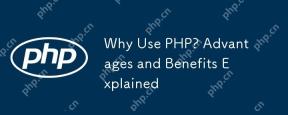
PHP的核心優勢包括易於學習、強大的web開發支持、豐富的庫和框架、高性能和可擴展性、跨平台兼容性以及成本效益高。 1)易於學習和使用,適合初學者;2)與web服務器集成好,支持多種數據庫;3)擁有如Laravel等強大框架;4)通過優化可實現高性能;5)支持多種操作系統;6)開源,降低開發成本。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

SublimeText3漢化版
中文版,非常好用
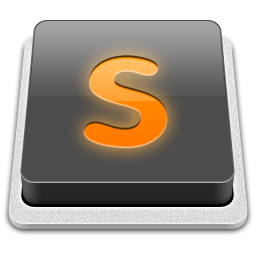
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)