Go語言中物件導向的特點及應用實例
摘要:本文將介紹Go語言中的物件導向程式設計特點及應用實例,並透過程式碼範例詳細說明在Go語言中如何使用物件導向的思想進行程式設計。
引言:物件導向程式設計是一種非常廣泛應用的程式設計範式,它透過將資料和操作封裝在一個物件中,並透過物件之間的互動來實現程式的邏輯。在Go語言中,物件導向程式設計也有著獨特的特點和應用實例,本文將對其進行詳細介紹。
一、物件導向的特點
- 封裝:封裝是物件導向程式設計的核心特點之一。在Go語言中,我們可以透過定義結構體來封裝資料和方法。結構體中的成員變數可以使用存取控制標識符來限制外部訪問,從而確保資料的安全性。
範例程式碼1:
package main import "fmt" type Rect struct { width float64 height float64 } func (r *Rect) Area() float64 { return r.width * r.height } func main() { rect := Rect{width: 3, height: 4} fmt.Println(rect.Area()) }
- 繼承:繼承是物件導向程式設計中的另一個重要特點。在Go語言中,可以使用匿名欄位和結構體嵌套的方式來實現繼承。透過繼承,可以實現程式碼的複用和擴充。
範例程式碼2:
package main import "fmt" type Animal struct { name string } func (a *Animal) SayName() { fmt.Println("My name is", a.name) } type Dog struct { Animal } func main() { dog := Dog{Animal: Animal{name: "Tom"}} dog.SayName() }
- 多態:多態是指相同的方法在不同物件上可以有不同的行為。在Go語言中,透過介面實現多型態。介面定義了一組方法簽名,任何類型只要實作了介面中的所有方法,就成為該介面的實作類型。
範例程式碼3:
package main import "fmt" type Shape interface { Area() float64 } type Rect struct { width float64 height float64 } func (r *Rect) Area() float64 { return r.width * r.height } type Circle struct { radius float64 } func (c *Circle) Area() float64 { return 3.14 * c.radius * c.radius } func printArea(s Shape) { fmt.Println("Area:", s.Area()) } func main() { rect := &Rect{width: 3, height: 4} circle := &Circle{radius: 2} printArea(rect) printArea(circle) }
二、物件導向的應用實例
- 圖形計算器:透過物件導向的思想,可以定義圖形對象,並實現各種圖形的計算方法,例如計算面積、週長等。
範例程式碼4:
package main import "fmt" type Shape interface { Area() float64 Perimeter() float64 } type Rectangle struct { length float64 width float64 } func (r *Rectangle) Area() float64 { return r.length * r.width } func (r *Rectangle) Perimeter() float64 { return 2 * (r.length + r.width) } type Circle struct { radius float64 } func (c *Circle) Area() float64 { return 3.14 * c.radius * c.radius } func (c *Circle) Perimeter() float64 { return 2 * 3.14 * c.radius } func main() { rectangle := &Rectangle{length: 3, width: 4} circle := &Circle{radius: 2} shapes := []Shape{rectangle, circle} for _, shape := range shapes { fmt.Println("Area:", shape.Area()) fmt.Println("Perimeter:", shape.Perimeter()) } }
- 購物車:透過物件導向的思想,可以定義商品物件和購物車對象,並實現購物車的新增、刪除、結算等功能。
範例程式碼5:
package main import "fmt" type Product struct { name string price float64 } type ShoppingCart struct { products []*Product } func (sc *ShoppingCart) AddProduct(product *Product) { sc.products = append(sc.products, product) } func (sc *ShoppingCart) RemoveProduct(name string) { for i, product := range sc.products { if product.name == name { sc.products = append(sc.products[:i], sc.products[i+1:]...) break } } } func (sc *ShoppingCart) CalculateTotalPrice() float64 { totalPrice := 0.0 for _, product := range sc.products { totalPrice += product.price } return totalPrice } func main() { product1 := &Product{name: "Apple", price: 2.5} product2 := &Product{name: "Banana", price: 1.5} product3 := &Product{name: "Orange", price: 1.0} shoppingCart := &ShoppingCart{} shoppingCart.AddProduct(product1) shoppingCart.AddProduct(product2) shoppingCart.AddProduct(product3) fmt.Println("Total Price:", shoppingCart.CalculateTotalPrice()) shoppingCart.RemoveProduct("Banana") fmt.Println("Total Price:", shoppingCart.CalculateTotalPrice()) }
總結:本文介紹了Go語言中物件導向程式設計的特點及應用實例,並透過程式碼範例詳細說明了在Go語言中如何使用面向對象的思想進行程式設計。物件導向程式設計能夠提高程式碼的複用性和擴充性,並且能夠更好地組織和管理程式邏輯,是一種非常重要且實用的程式設計範式。
以上是Go語言中物件導向的特性及應用實例的詳細內容。更多資訊請關注PHP中文網其他相關文章!
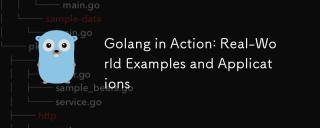
Golang在实际应用中表现出色,以简洁、高效和并发性著称。1)通过Goroutines和Channels实现并发编程,2)利用接口和多态编写灵活代码,3)使用net/http包简化网络编程,4)构建高效并发爬虫,5)通过工具和最佳实践进行调试和优化。
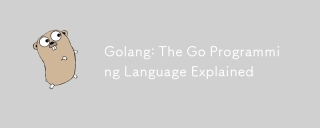
Go語言的核心特性包括垃圾回收、靜態鏈接和並發支持。 1.Go語言的並發模型通過goroutine和channel實現高效並發編程。 2.接口和多態性通過實現接口方法,使得不同類型可以統一處理。 3.基本用法展示了函數定義和調用的高效性。 4.高級用法中,切片提供了動態調整大小的強大功能。 5.常見錯誤如競態條件可以通過gotest-race檢測並解決。 6.性能優化通過sync.Pool重用對象,減少垃圾回收壓力。
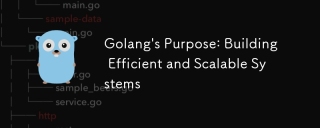
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
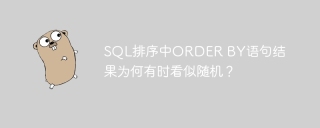
關於SQL查詢結果排序的疑惑學習SQL的過程中,常常會遇到一些令人困惑的問題。最近,筆者在閱讀《MICK-SQL基礎�...
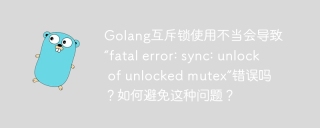
golang ...
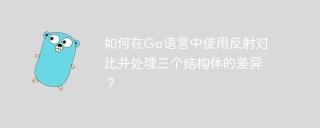
Go語言中如何對比並處理三個結構體在Go語言編程中,有時需要對比兩個結構體的差異,並將這些差異應用到第�...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中
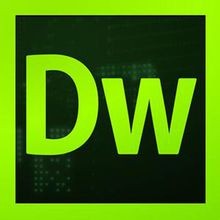
Dreamweaver CS6
視覺化網頁開發工具
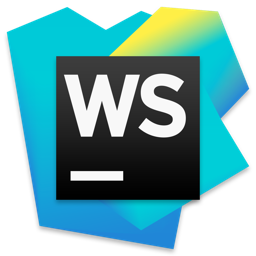
WebStorm Mac版
好用的JavaScript開發工具