vue元件傳值的方式:1、父傳子;2、子傳父;3、兄弟傳值;4、問號傳值,冒號傳值和父子元件傳值;5、使用“ $ref」傳值;6、使用「inject」為目前實例注入父組件的資料;7、祖傳孫;8、孫傳祖;9、$parent;10、sessionStorage傳值;11、vuex。
1、父元件傳給子元件
#在子元件裡定義一個props,即props:['msg'],msg可以是物件也可以是基本資料型別
如果你想定義一個預設值,也就是props:{msg: {type: String, default: 'hello world'}},
#若預設值是物件類型:props: { msg: { type: Object, default: () => { return { name: 'dan_seek' } } }}
需要注意的是這種傳值是單向的,你無法改變父元件的值(當然引用型別例外);而且如果直接修改props的值會報一個警告。
建議的寫法是在data()裡重新定義一個變數(見Children.vue),並且把props賦值給它,當然計算屬性也行。
Children.vue
<template> <section> 父组件传过来的消息是:{{myMsg}} </section> </template> <script> export default { name: "Children", components: {}, props:['msg'], data() { return { myMsg:this.msg } }, methods: {} } </script>
Parent.vue
<template> <div class="parent"> <Children :msg="message"></Children> </div> </template> <script> import Children from '../components/Children' export default { name: 'Parent', components: { Children }, data() { return { message:'hello world' } }, } </script>
2、子元件傳給父元件
這裡需要使用自訂事件,在子元件中使用this.$emit('myEvent') 觸發,然後在父元件中使用@myEvent監聽
Children.vue
<template> <section> <br> <div @click="clickme">click me</div> </section> </template> <script> export default { name: "Children", components: {}, data() { return { childNum:0 } }, methods: { clickme(){ // 通过自定义事件addNum把值传给父组件 this.$emit('addNum',this.childNum++) } } } </script>
Parent.vue
<template> <div class="parent"> 这里是计数:{{parentNum}} <Children-Com @addNum="getNum"></Children-Com> </div> </template> <script> import ChildrenCom from '../components/Children' export default { name: 'Parent', components: { ChildrenCom }, data() { return { parentNum: 0 } }, methods:{ // childNum是由子组件传入的 getNum(childNum){ this.parentNum = childNum } } } </script>
3、兄弟元件間傳值
運用自訂事件emit的觸發和監聽能力,定義一個公共的事件匯流排eventBus,透過它作為中間橋樑,我們就可以傳值給任意元件了。而且透過eventBus的使用,可以加深emit的理解。
EventBus.js
import Vue from 'vue'export default new Vue() Children1.vue <template> <section> <div @click="pushMsg">push message</div> <br> </section></template><script> import eventBus from './EventBus' export default { name: "Children1", components: {}, data() { return { childNum:0 } }, methods: { pushMsg(){ // 通过事件总线发送消息 eventBus.$emit('pushMsg',this.childNum++) } } }</script>
Children2.vue
<template> <section> children1传过来的消息:{{msg}} </section> </template> <script> import eventBus from './EventBus' export default { name: "Children2", components: {}, data() { return { msg: '' } }, mounted() { // 通过事件总线监听消息 eventBus.$on('pushMsg', (children1Msg) => { this.msg = children1Msg }) } } </script>
Parent.vue
<template> <div class="parent"> <Children1></Children1> <Children2></Children2> </div> </template> <script> import Children1 from '../components/Children1' import Children2 from '../components/Children2' export default { name: 'Parent', components: { Children1, Children2 }, data() { return { } }, methods:{ } } </script>
github上還有一個開源vue-bus庫,可以參考下: https://github.com/yangmingshan/vue-bus#readme
4、路由間傳值
i.使用問號傳值
A頁面跳轉B頁面時使用this.$router.push('/B?name=danseek')
B頁面可以使用this.$route.query.name 來取得A頁面傳過來的值
#上面要注意router和route的區別
ii.使用冒號傳值
配置如下路由:
{ path: '/b/:name', name: 'b', component: () => import( '../views/B.vue') },
在B頁面可以透過this.$route.params .name 來取得路由傳入的name的值
iii.使用父子元件傳值
#由於router-view本身也是一個元件,所以我們也可以使用父子元件傳值方式傳值,然後在對應的子頁面裡加上props,因為type更新後沒有刷新路由,所以不能直接在子頁面的mounted鉤子裡直接取得最新type的值,而要使用watch。
<router-view :type="type"></router-view> // 子页面 ...... props: ['type'] ...... watch: { type(){ // console.log("在这个方法可以时刻获取最新的数据:type=",this.type) }, },
5、使用$ref傳值
透過$ref的能力,給子元件定義一個ID,父元件透過這個ID可以直接存取子元件裡面的方法和屬性
先定義一個子元件Children.vue
<template> <section> 传过来的消息:{{msg}} </section> </template> <script> export default { name: "Children", components: {}, data() { return { msg: '', desc:'The use of ref' } }, methods:{ // 父组件可以调用这个方法传入msg updateMsg(msg){ this.msg = msg } }, } </script>
然後在父元件Parent.vue中引用Children.vue,並定義ref屬性
<template> <div class="parent"> <!-- 给子组件设置一个ID ref="children" --> <Children ref="children"></Children> <div @click="pushMsg">push message</div> </div> </template> <script> import Children from '../components/Children' export default { name: 'parent', components: { Children, }, methods:{ pushMsg(){ // 通过这个ID可以访问子组件的方法 this.$refs.children.updateMsg('Have you received the clothes?') // 也可以访问子组件的属性 console.log('children props:',this.$refs.children.desc) } }, } </script>
6、使用依賴注入傳給後代子孫曾孫
假設父元件有一個方法getName(),需要把它提供給所有的後代
provide: function () { return { getName: this.getName() } }
provide 選項允許我們指定我們想要提供給後代元件的資料/方法
然後在任何後代元件裡,我們都可以使用inject 來為目前實例注入父元件的資料/方法:
inject: ['getName']
Parent.vue
<template> <div class="parent"> <Children></Children> </div> </template> <script> import Children from '../components/Children' export default { name: 'Parent', components: { Children, }, data() { return { name:'dan_seek' } }, provide: function () { return { getName: this.name } }, } </script>
Children.vue
父组件传入的值:{{getName}} <script> export default { name: "Children", components: {}, data() { return { } }, inject: [&#39;getName&#39;], } </script>
7、祖傳孫$attrs
正常情況下需要藉助父親的props作為中間過渡,但是這樣在父親組件就會多了一些跟父組件業務無關的屬性,耦合度高,借助$attrs可以簡化些,而且祖跟孫都無需做修改
GrandParent.vue
<template> <section> <parent name="grandParent" sex="男" age="88" hobby="code" @sayKnow="sayKnow"></parent> </section> </template> <script> import Parent from './Parent' export default { name: "GrandParent", components: { Parent }, data() { return {} }, methods: { sayKnow(val){ console.log(val) } }, mounted() { } } </script>
Parent.vue
<template> <section> <p>父组件收到</p> <p>祖父的名字:{{name}}</p> <children v-bind="$attrs" v-on="$listeners"></children> </section> </template> <script> import Children from './Children' export default { name: "Parent", components: { Children }, // 父组件接收了name,所以name值是不会传到子组件的 props:['name'], data() { return {} }, methods: {}, mounted() { } } </script>
Children.vue
<template> <section> <p>子组件收到</p> <p>祖父的名字:{{name}}</p> <p>祖父的性别:{{sex}}</p> <p>祖父的年龄:{{age}}</p> <p>祖父的爱好:{{hobby}}</p> <button @click="sayKnow">我知道啦</button> </section> </template> <script> export default { name: "Children", components: {}, // 由于父组件已经接收了name属性,所以name不会传到子组件了 props:['sex','age','hobby','name'], data() { return {} }, methods: { sayKnow(){ this.$emit('sayKnow','我知道啦') } }, mounted() { } } </script>
顯示結果
父元件收到
祖父的名字:grandParent
子元件收到
祖父的名字:
祖父的性別:男
祖父的年齡:88
祖父的愛好:code
8、孫傳祖
借助$ listeners中間事件,孫可以方便的通知祖,程式碼範例見7
9、$parent
透過parent可以獲父元件實例,然後透過這個實例就可以存取父元件的屬性和方法,它還有一個兄弟root,可以取得根元件實例。
語法:
// 获父组件的数据 this.$parent.foo // 写入父组件的数据 this.$parent.foo = 2 // 访问父组件的计算属性 this.$parent.bar // 调用父组件的方法 this.$parent.baz()
於是,在子元件傳給父元件範例中,可以使用this.$parent.getNum(100)傳值給父元件。
10、sessionStorage傳值
sessionStorage 是瀏覽器的全域對象,存在它裡面的資料會在頁面關閉時清除 。運用這個特性,我們可以在所有頁面分享一份資料。
語法:
// 保存数据到 sessionStorage sessionStorage.setItem('key', 'value'); // 从 sessionStorage 获取数据 let data = sessionStorage.getItem('key'); // 从 sessionStorage 删除保存的数据 sessionStorage.removeItem('key'); // 从 sessionStorage 删除所有保存的数据 sessionStorage.clear();
注意:裡面存的是鍵值對,只能是字串類型,如果要存物件的話,需要使用let objStr = JSON.stringify(obj) 轉成字串然後再儲存(使用的時候let obj = JSON.parse(objStr) 解析為物件)。
這樣存物件是不是很麻煩呢,推薦一個函式庫 good-storage ,它封裝了sessionStorage ,可以直接用它的API存物件
// localStorage storage.set(key,val) storage.get(key, def) // sessionStorage storage.session.set(key, val) storage.session.get(key, val)
11、vuex
這裡我也不打算介紹這個大名鼎鼎的vuex怎麼用,因為要把它寫清楚篇幅太長了…
如果您不打算開發大型單頁應用,使用Vuex 可能是繁瑣冗餘的。確實是如此——如果您的應用夠簡單,您也許不需要使用 Vuex。
以上是vue組件傳值的方式的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
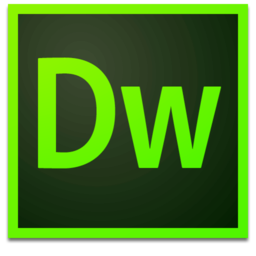
Dreamweaver Mac版
視覺化網頁開發工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。
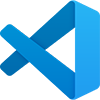
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具