Vue和Axios實作資料請求的錯誤處理和提示機制
#引言:
在Vue開發中,經常會使用Axios進行資料請求。然而,在實際開發過程中,我們經常會遇到請求出錯或伺服器回傳錯誤碼的情況。為了提升使用者體驗並及時發現並處理請求錯誤,我們需要使用一些機制來進行錯誤處理和提示。本文將介紹如何使用Vue和Axios實作資料請求的錯誤處理和提示機制,並提供程式碼範例。
-
安裝Axios
首先,我們需要安裝Axios。可以使用以下命令進行安裝:npm install axios
-
建立Axios實例
在使用Axios發送請求前,我們需要建立一個Axios實例。可以在Vue的main.js檔案中加入以下程式碼:import Vue from 'vue' import Axios from 'axios' Vue.prototype.$axios = Axios.create({ baseURL: 'http://api.example.com', // 设置请求的基准URL timeout: 5000 // 设置请求超时时间 })
在上述程式碼中,我們使用Vue的原型屬性$axios建立一個Axios實例,並設定了請求的基準URL和逾時時間。
-
發送請求
現在我們可以在Vue元件中使用Axios發送請求了。在傳送請求時,我們可以使用Axios的interceptors屬性對請求進行攔截,以便進行錯誤處理和提示。可以在Vue的元件中加入以下程式碼:methods: { fetchData() { this.$axios.get('/data') .then(response => { // 请求成功逻辑 console.log(response.data) }) .catch(error => { // 请求失败逻辑 console.error(error) this.handleError(error) }) }, handleError(error) { // 处理请求错误逻辑 if (error.response) { // 请求已发出,但服务器返回错误码 console.error(error.response.data) console.error(error.response.status) console.error(error.response.headers) } else { // 请求未发出,网络错误等 console.error('Error', error.message) } // 错误提示逻辑 this.$message.error('请求出错,请稍后重试') } }
在上述程式碼中,我們使用了Axios的catch方法來捕獲請求錯誤,並呼叫handleError方法進行錯誤處理。在handleError方法中,我們可以根據錯誤的類型進行不同的處理邏輯,例如輸出錯誤訊息、展示錯誤提示。
-
錯誤提示元件
為了更好地展示錯誤提示,我們可以使用一些UI庫中的錯誤提示元件。例如,我們可以使用Element-UI庫中的Message元件。可以在Vue元件中加入以下程式碼:mounted() { this.$message({ message: '页面加载成功', type: 'success' }); }, methods: { handleError(error) { // 处理请求错误逻辑 if (error.response) { // 请求已发出,但服务器返回错误码 console.error(error.response.data) console.error(error.response.status) console.error(error.response.headers) } else { // 请求未发出,网络错误等 console.error('Error', error.message) } // 错误提示逻辑 this.$message.error('请求出错,请稍后重试') } }
在上述程式碼中,我們使用了this.$message方法來展示錯誤提示。
總結:
透過以上的步驟,我們成功地實現了Vue和Axios的資料請求錯誤處理和提示機制。在實際開發中,我們可以根據具體需求,對錯誤處理和提示進行進一步的擴展和最佳化。希望本文能對您在Vue開發中遇到的資料請求問題有所幫助。
參考文獻:
[1] Axios官方文檔- https://github.com/axios/axios
[2] Element-UI官方文件- https://element.eleme. io/
附錄:完整程式碼範例
<template> <div> <button @click="fetchData">点击获取数据</button> </div> </template> <script> export default { mounted() { this.$message({ message: '页面加载成功', type: 'success' }); }, methods: { fetchData() { this.$axios.get('/data') .then(response => { // 请求成功逻辑 console.log(response.data) }) .catch(error => { // 请求失败逻辑 console.error(error) this.handleError(error) }) }, handleError(error) { // 处理请求错误逻辑 if (error.response) { // 请求已发出,但服务器返回错误码 console.error(error.response.data) console.error(error.response.status) console.error(error.response.headers) } else { // 请求未发出,网络错误等 console.error('Error', error.message) } // 错误提示逻辑 this.$message.error('请求出错,请稍后重试') } } } </script>
以上是Vue和Axios實作資料請求的錯誤處理和提示機制的詳細內容。更多資訊請關注PHP中文網其他相關文章!

Netflix使用React作為其前端框架。 1)React的組件化開發模式和強大生態系統是Netflix選擇它的主要原因。 2)通過組件化,Netflix將復雜界面拆分成可管理的小塊,如視頻播放器、推薦列表和用戶評論。 3)React的虛擬DOM和組件生命週期優化了渲染效率和用戶交互管理。

Netflix在前端技術上的選擇主要集中在性能優化、可擴展性和用戶體驗三個方面。 1.性能優化:Netflix選擇React作為主要框架,並開發了SpeedCurve和Boomerang等工具來監控和優化用戶體驗。 2.可擴展性:他們採用微前端架構,將應用拆分為獨立模塊,提高開發效率和系統擴展性。 3.用戶體驗:Netflix使用Material-UI組件庫,通過A/B測試和用戶反饋不斷優化界面,確保一致性和美觀性。

NetflixusesAcustomFrameworkcalled“ Gibbon” BuiltonReact,notReactorVuedIrectly.1)TeamSperience:selectBasedonFamiliarity.2)ProjectComplexity:vueforsimplerprojects:reactforforforproproject,reactforforforcompleplexones.3)cocatizationneedneeds:reactoffipicatizationneedneedneedneedneedneeds:reactoffersizationneedneedneedneedneeds:reactoffersizatization needefersmoreflexibleise.4)

Netflix在框架選擇上主要考慮性能、可擴展性、開發效率、生態系統、技術債務和維護成本。 1.性能與可擴展性:選擇Java和SpringBoot以高效處理海量數據和高並發請求。 2.開發效率與生態系統:使用React提升前端開發效率,利用其豐富的生態系統。 3.技術債務與維護成本:選擇Node.js構建微服務,降低維護成本和技術債務。

Netflix主要使用React作為前端框架,輔以Vue用於特定功能。 1)React的組件化和虛擬DOM提升了Netflix應用的性能和開發效率。 2)Vue在Netflix的內部工具和小型項目中應用,其靈活性和易用性是關鍵。

Vue.js是一種漸進式JavaScript框架,適用於構建複雜的用戶界面。 1)其核心概念包括響應式數據、組件化和虛擬DOM。 2)實際應用中,可以通過構建Todo應用和集成VueRouter來展示其功能。 3)調試時,建議使用VueDevtools和console.log。 4)性能優化可通過v-if/v-show、列表渲染優化和異步加載組件等實現。

Vue.js適合小型到中型項目,而React更適用於大型、複雜應用。 1.Vue.js的響應式系統通過依賴追踪自動更新DOM,易於管理數據變化。 2.React採用單向數據流,數據從父組件流向子組件,提供明確的數據流向和易於調試的結構。

Vue.js適合中小型項目和快速迭代,React適用於大型複雜應用。 1)Vue.js易於上手,適用於團隊經驗不足或項目規模較小的情況。 2)React的生態系統更豐富,適合有高性能需求和復雜功能需求的項目。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

Atom編輯器mac版下載
最受歡迎的的開源編輯器

SublimeText3 Linux新版
SublimeText3 Linux最新版
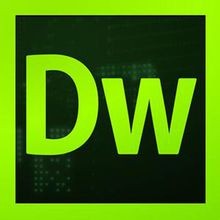
Dreamweaver CS6
視覺化網頁開發工具

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。